cppreference.com
Copy constructors.
A copy constructor is a constructor which can be called with an argument of the same class type and copies the content of the argument without mutating the argument.

[ edit ] Syntax
[ edit ] explanation.
The copy constructor is called whenever an object is initialized (by direct-initialization or copy-initialization ) from another object of the same type (unless overload resolution selects a better match or the call is elided ), which includes
- initialization: T a = b ; or T a ( b ) ; , where b is of type T ;
- function argument passing: f ( a ) ; , where a is of type T and f is void f ( T t ) ;
- function return: return a ; inside a function such as T f ( ) , where a is of type T , which has no move constructor .
[ edit ] Implicitly-declared copy constructor
If no user-defined copy constructors are provided for a class type, the compiler will always declare a copy constructor as a non- explicit inline public member of its class. This implicitly-declared copy constructor has the form T :: T ( const T & ) if all of the following are true:
- each direct and virtual base B of T has a copy constructor whose parameters are of type const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy constructor whose parameters are of type const M & or const volatile M & .
Otherwise, the implicitly-declared copy constructor is T :: T ( T & ) .
Due to these rules, the implicitly-declared copy constructor cannot bind to a volatile lvalue argument.
A class can have multiple copy constructors, e.g. both T :: T ( const T & ) and T :: T ( T & ) .
The implicitly-declared (or defaulted on its first declaration) copy constructor has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17) .
[ edit ] Implicitly-defined copy constructor
If the implicitly-declared copy constructor is not deleted, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++11) . For union types, the implicitly-defined copy constructor copies the object representation (as by std::memmove ). For non-union class types, the constructor performs full member-wise copy of the object's direct base subobjects and member subobjects, in their initialization order, using direct initialization. For each non-static data member of a reference type, the copy constructor binds the reference to the same object or function to which the source reference is bound.
[ edit ] Deleted copy constructor
The implicitly-declared or explicitly-defaulted (since C++11) copy constructor for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that
- M has a destructor that is deleted or (since C++11) inaccessible from the copy constructor, or
- the overload resolution as applied to find M 's copy constructor
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy constructor
The copy constructor for class T is trivial if all of the following are true:
- it is not user-provided (that is, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy constructor selected for every direct base of T is trivial;
- the copy constructor selected for every non-static class type (or array of class type) member of T is trivial;
A trivial copy constructor for a non-union class effectively copies every scalar subobject (including, recursively, subobject of subobjects and so forth) of the argument and performs no other action. However, padding bytes need not be copied, and even the object representations of the copied subobjects need not be the same as long as their values are identical.
TriviallyCopyable objects can be copied by copying their object representations manually, e.g. with std::memmove . All data types compatible with the C language (POD types) are trivially copyable.
[ edit ] Eligible copy constructor
Triviality of eligible copy constructors determines whether the class is an implicit-lifetime type , and whether the class is a trivially copyable type .
[ edit ] Notes
In many situations, copy constructors are optimized out even if they would produce observable side-effects, see copy elision .
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy assignment
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 4 June 2024, at 23:47.
- Privacy policy
- About cppreference.com
- Disclaimers

This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Copy constructors and copy assignment operators (C++)
- 8 contributors
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment . In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++) .
Both the assignment operation and the initialization operation cause objects to be copied.
Assignment : When one object's value is assigned to another object, the first object is copied to the second object. So, this code copies the value of b into a :
Initialization : Initialization occurs when you declare a new object, when you pass function arguments by value, or when you return by value from a function.
You can define the semantics of "copy" for objects of class type. For example, consider this code:
The preceding code could mean "copy the contents of FILE1.DAT to FILE2.DAT" or it could mean "ignore FILE2.DAT and make b a second handle to FILE1.DAT." You must attach appropriate copying semantics to each class, as follows:
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x); .
Use the copy constructor.
If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you. Similarly, if you don't declare a copy assignment operator, the compiler generates a member-wise copy assignment operator for you. Declaring a copy constructor doesn't suppress the compiler-generated copy assignment operator, and vice-versa. If you implement either one, we recommend that you implement the other one, too. When you implement both, the meaning of the code is clear.
The copy constructor takes an argument of type ClassName& , where ClassName is the name of the class. For example:
Make the type of the copy constructor's argument const ClassName& whenever possible. This prevents the copy constructor from accidentally changing the copied object. It also lets you copy from const objects.
Compiler generated copy constructors
Compiler-generated copy constructors, like user-defined copy constructors, have a single argument of type "reference to class-name ." An exception is when all base classes and member classes have copy constructors declared as taking a single argument of type const class-name & . In such a case, the compiler-generated copy constructor's argument is also const .
When the argument type to the copy constructor isn't const , initialization by copying a const object generates an error. The reverse isn't true: If the argument is const , you can initialize by copying an object that's not const .
Compiler-generated assignment operators follow the same pattern for const . They take a single argument of type ClassName& unless the assignment operators in all base and member classes take arguments of type const ClassName& . In this case, the generated assignment operator for the class takes a const argument.
When virtual base classes are initialized by copy constructors, whether compiler-generated or user-defined, they're initialized only once: at the point when they are constructed.
The implications are similar to the copy constructor. When the argument type isn't const , assignment from a const object generates an error. The reverse isn't true: If a const value is assigned to a value that's not const , the assignment succeeds.
For more information about overloaded assignment operators, see Assignment .
Was this page helpful?
Additional resources

14.14 — Introduction to the copy constructor
Consider the following program:
You might be surprised to find that this program compiles just fine, and produces the result:
Let’s take a closer look at how this program works.
The initialization of variable f is just a standard brace initialization that calls the Fraction(int, int) constructor.
But what about the next line? The initialization of variable fCopy is also clearly an initialization, and you know that constructor functions are used to initialize classes. So what constructor is this line calling?
The answer is: the copy constructor.
The copy constructor
A copy constructor is a constructor that is used to initialize an object with an existing object of the same type. After the copy constructor executes, the newly created object should be a copy of the object passed in as the initializer.
An implicit copy constructor
If you do not provide a copy constructor for your classes, C++ will create a public implicit copy constructor for you. In the above example, the statement Fraction fCopy { f }; is invoking the implicit copy constructor to initialize fCopy with f .
By default, the implicit copy constructor will do memberwise initialization. This means each member will be initialized using the corresponding member of the class passed in as the initializer. In the example above, fCopy.m_numerator is initialized using f.m_numerator (which has value 5 ), and fCopy.m_denominator is initialized using f.m_denominator (which has value 3 ).
After the copy constructor has executed, the members of f and fCopy have the same values, so fCopy is a copy of f . Thus calling print() on either has the same result.
Defining your own copy constructor
We can also explicitly define our own copy constructor. In this lesson, we’ll make our copy constructor print a message, so we can show you that it is indeed executing when copies are made.
The copy constructor looks just like you’d expect it to:
When this program is run, you get:
The copy constructor we defined above is functionally equivalent to the one we’d get by default, except we’ve added an output statement to prove the copy constructor is actually being called. This copy constructor is invoked when fCopy is initialized with f .
Access controls work on a per-class basis (not a per-object basis). This means the member functions of a class can access the private members of any class object of the same type (not just the implicit object).
We use that to our advantage in the Fraction copy constructor above in order to directly access the private members of the fraction parameter. Otherwise, we would have no way to access those members directly (without adding access functions, which we might not want to do).
A copy constructor should not do anything other than copy an object. This is because the compiler may optimize the copy constructor out in certain cases. If you are relying on the copy constructor for some behavior other than just copying, that behavior may or may not occur. We discuss this further in lesson 14.15 -- Class initialization and copy elision .
Best practice
Copy constructors should have no side effects beyond copying.
Prefer the implicit copy constructor
Unlike the implicit default constructor, which does nothing (and thus is rarely what we want), the memberwise initialization performed by the implicit copy constructor is usually exactly what we want. Therefore, in most cases, using the implicit copy constructor is perfectly fine.
Prefer the implicit copy constructor, unless you have a specific reason to create your own.
We’ll see cases where the copy constructor needs to be overwritten when we discuss dynamic memory allocation ( 21.13 -- Shallow vs. deep copying ).
The copy constructor’s parameter must be a reference
It is a requirement that the parameter of a copy constructor be an lvalue reference or const lvalue reference. Because the copy constructor should not be modifying the parameter, using a const lvalue reference is preferred.
If you write your own copy constructor, the parameter should be a const lvalue reference.
Pass by value and the copy constructor
When an object is passed by value, the argument is copied into the parameter. When the argument and parameter are the same class type, the copy is made by implicitly invoking the copy constructor.
This is illustrated in the following example:
On the author’s machine, this example prints:
In the above example, the call to printFraction(f) is passing f by value. The copy constructor is invoked to copy f from main into the f parameter of function printFraction() .
Return by value and the copy constructor
In lesson 2.5 -- Introduction to local scope , we noted that return by value creates a temporary object (holding a copy of the return value) that is passed back to the caller. When the return type and the return value are the same class type, the temporary object is initialized by implicitly invoking the copy constructor.
For example:
When generateFraction returns a Fraction back to main , a temporary Fraction object is created and initialized using the copy constructor.
Because this temporary is used to initialize Fraction f2 , this invokes the copy constructor again to copy the temporary into f2 .
And when f2 is passed to printFraction() , the copy constructor is called a third time.
Thus, on the author’s machine, this example prints:
If you compile and execute the above example, you may find that only two calls to the copy constructor occur. This is a compiler optimization known as copy elision . We discuss copy elision further in lesson 14.15 -- Class initialization and copy elision .
Using = default to generate a default copy constructor
If a class has no copy constructor, the compiler will implicitly generate one for us. If we prefer, we can explicitly request the compiler create a default copy constructor for us using the = default syntax:
Using = delete to prevent copies
Occasionally we run into cases where we do not want objects of a certain class to be copyable. We can prevent this by marking the copy constructor function as deleted, using the = delete syntax:
In the example, when the compiler goes to find a constructor to initialize fCopy with f , it will see that the copy constructor has been deleted. This will cause it to emit a compile error.
As an aside…
You can also prevent the public from making copies of class object by making the copy constructor private (as private functions can’t be called by the public). However, a private copy constructor can still be called from other members of the class, so this solution is not advised unless that is desired.
For advanced readers
The rule of three is a well known C++ principle that states that if a class requires a user-defined copy constructor, destructor, or copy assignment operator, then it probably requires all three. In C++11, this was expanded to the rule of five , which adds the move constructor and move assignment operator to the list.
Not following the rule of three/rule of five is likely to lead to malfunctioning code. We’ll revisit the rule of three and rule of five when we cover dynamic memory allocation.
We discuss destructors in lesson 15.4 -- Introduction to destructors and 19.3 -- Destructors , and copy assignment in lesson 21.12 -- Overloading the assignment operator .
Question #1
In the lesson above, we noted that the parameter for a copy constructor must be a (const) reference. Why aren’t we allowed to use pass by value?
Show Solution
When we pass a class type argument by value, the copy constructor is implicitly invoked to copy the argument into the parameter.
If the copy constructor used pass by value, the copy constructor would need to call itself to copy the initializer argument into the copy constructor parameter. But that call to the copy constructor would also be pass by value, so the copy constructor would be invoked again to copy the argument into the function parameter. This would lead to an infinite chain of calls to the copy constructor.
- C++ Classes and Objects
- C++ Polymorphism
- C++ Inheritance
- C++ Abstraction
- C++ Encapsulation
- C++ OOPs Interview Questions
- C++ OOPs MCQ
- C++ Interview Questions
- C++ Function Overloading
- C++ Programs
- C++ Preprocessor
- C++ Templates
Copy Constructor in C++
A copy constructor is a type of constructor that initializes an object using another object of the same class. In simple terms, a constructor which creates an object by initializing it with an object of the same class, which has been created previously is known as a copy constructor .
The process of initializing members of an object through a copy constructor is known as copy initialization . It is also called member-wise initialization because the copy constructor initializes one object with the existing object, both belonging to the same class on a member-by-member copy basis.
Syntax of Copy Constructor in C++
Copy constructor takes a reference to an object of the same class as an argument:
Here, the const qualifier is optional but is added so that we do not modify the obj by mistake.
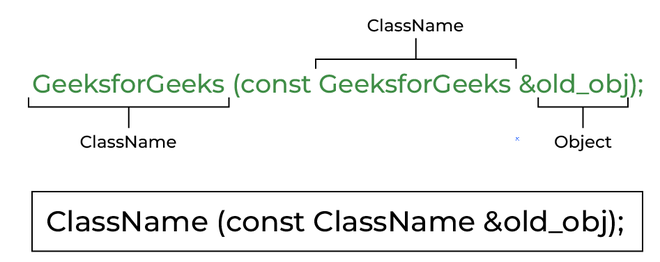
Syntax of Copy Constructor
For a deeper understanding of constructors and memory management, check out our Complete C++ Course , which covers constructors, destructors, and advanced object management techniques.

Examples of Copy Constructor in C++
Example 1: user defined copy constructor.
If the programmer does not define the copy constructor, the compiler does it for us.
Example 2: Default Copy Constructor
An implicitly defined copy constructor will copy the bases and members of an object in the same order that a constructor would initialize the bases and members of the object.
Need of User Defined Copy Constructor
If we don’t define our own copy constructor, the C++ compiler creates a default copy constructor for each class which works fine in general. However, we need to define our own copy constructor only if an object has pointers or any runtime allocation of the resource like a file handle , a network connection, etc because the default constructor does only shallow copy.
Shallow Copy means that only the pointers will be copied not the actual resources that the pointers are pointing to. This can lead to dangling pointers if the original object is deleted.
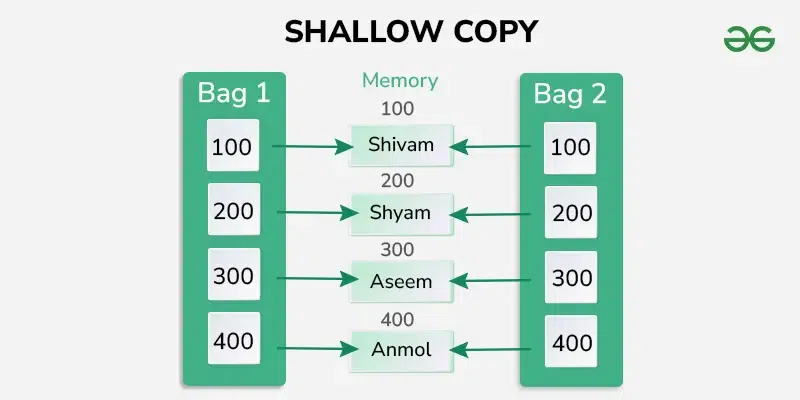
Deep copy is possible only with a user-defined copy constructor. In a user-defined copy constructor, we make sure that pointers (or references) of copied objects point to new copy of the dynamic resource allocated manually in the copy constructor using new operators.
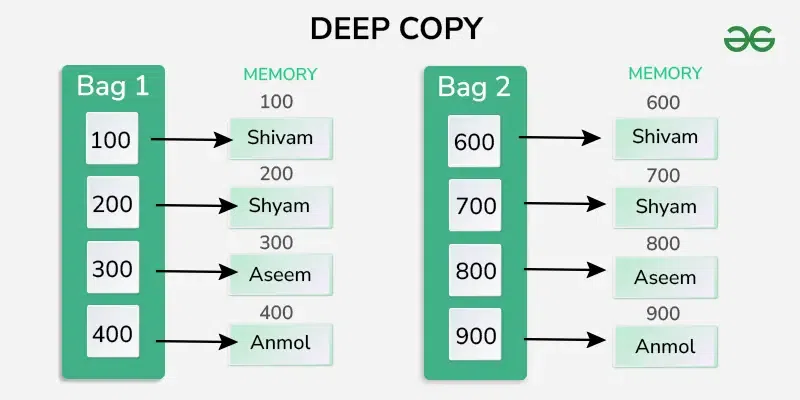
Example: Class Where a Copy Constructor is Required
Following is a complete C++ program to demonstrate the use of the Copy constructor. In the following String class, we must write a copy constructor.
Note: Such classes also need the overloaded assignment operator. See this article for more info – C++ Assignment Operator Overloading
What would be the problem if we remove the copy constructor from the above code?
If we remove the copy constructor from the above program, we don’t get the expected output. The c hanges made to str2 reflect in str1 as well which is never expected. Also, if the str1 is destroyed, the str2’s data member s will be pointing to the deallocated memory.
When is the Copy Constructor Called?
In C++, a copy constructor may be called in the following cases:
- When an object of the class is returned by value.
- When an object of the class is passed (to a function) by value as an argument.
- When an object is constructed based on another object of the same class.
- When the compiler generates a temporary object.
It is, however, not guaranteed that a copy constructor will be called in all these cases, because the C++ Standard allows the compiler to optimize the copy away in certain cases, one example is the return value optimization (sometimes referred to as RVO).
Refer to this article for more details – When is a Copy Constructor Called in C++?
Copy Elision
In copy elision, the compiler prevents the making of extra copies by making the use to techniques such as NRVO and RVO which results in saving space and better the program complexity (both time and space); Hence making the code more optimized.
Copy Constructor vs Assignment Operator
The main difference between Copy Constructor and Assignment Operator is that the Copy constructor makes a new memory storage every time it is called while the assignment operator does not make new memory storage.
Which of the following two statements calls the copy constructor and which one calls the assignment operator?
A copy constructor is called when a new object is created from an existing object, as a copy of the existing object. The assignment operator is called when an already initialized object is assigned a new value from another existing object. In the above example (1) calls the copy constructor and (2) calls the assignment operator.
Frequently Asked Questions in C++ Copy Constructors
Can we make the copy constructor private .
Yes, a copy constructor can be made private. When we make a copy constructor private in a class, objects of that class become non-copyable. This is particularly useful when our class has pointers or dynamically allocated resources. In such situations, we can either write our own copy constructor like the above String example or make a private copy constructor so that users get compiler errors rather than surprises at runtime.
Why argument to a copy constructor must be passed as a reference?
If you pass the object by value in the copy constructor, it will result in a recursive call to the copy constructor itself. This happens because passing by value involves making a copy, and making a copy involves calling the copy constructor, leading to an infinite recursion. Using a reference avoids this recursion. So, we use reference of objects to avoid infinite calls.
Why argument to a copy constructor should be const?
One reason for passing const reference is, that we should use const in C++ wherever possible so that objects are not accidentally modified. This is one good reason for passing reference as const , but there is more to it than ‘ Why argument to a copy constructor should be const?’
Related Articles:
- Constructors in C++
Similar Reads
- cpp-constructor
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
There are two obvious differences between copy constructor and copy assignment operator. copy constructor NO NEED to delete old elements, it just copy construct a new object. (as it Vector v2) copy constructor NO NEED to return the this pointer. (Furthermore, all the constructor does not return a value).
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them: Copy constructor. Assignment operator. It is called when a new object is created from an existing object, as a copy of the existing object.
the difference between a copy constructor and an assignment constructor is: In case of a copy constructor it creates a new object. (<classname> <o1>=<o2>) In case of an assignment constructor it will not create any object means it apply on already created objects (<o1>=<o2>).
Copy assignment operator. A copy assignment operator is a non-template non-static member function with the name operator= that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
A copy constructor is a constructor which can be called with an argument of the same class type and copies the content of the argument without mutating the argument. Syntax. class-name. - the class whose copy constructor is being declared. parameter-list. -
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment. In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++).
A copy constructor is a constructor that is used to initialize an object with an existing object of the same type. After the copy constructor executes, the newly created object should be a copy of the object passed in as the initializer.
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them: Copy constructor Assignment operator It is called when a new object is created from an existing object, as a copy of the existing objectThis operator is called when an already
Copy Constructors. Write a prototype for the constructor that would want to be called by the red line of code. Realm of Reasonable Answers: We want a constructor that will build a new Complex object (c3) by making a copy of another (c1) class Complex { public: Complex(); Complex(double r, double i);
C++ handles object copying and assignment through two functions called copy constructors and assignment operators. While C++ will automatically provide these functions if you don't explicitly define them, in some cases you'll need to manually control how your objects are duplicated.