Palindrome in Python

Today we are going to learn about the palindrome series and how to implement and identify a palindrome in Python. So let’s dive right into it!

What is a Palindrome?
A number is defined as a Palindrome number if it reads the exact same from both forward and backward. And the crazy thing is that it is not only valid to numbers. Even if a string reads the same forwards and backward, then it is a Palindrome as well!
Let us look at some examples to understand it better.
What is a Palindrome series?
1. palindrome numbers.
Let us consider two numbers: 123321 and 1234561.
The first number 123321 , when read forward and backward is the same number. Hence it is a palindrome number.
On the other hand, 1234561 , when reading backward is 1654321 which is definitely not the same as the original number. Hence, it is not a Palindrome Number.
2. Palindrome Strings
The logic that was explained for the Palindrome Numbers is also applicable to the strings. Let’s consider two basic strings: aba and abc.
String aba reads the same no matter how it is read (backward or forward). But on the other hand string abc when reading backward results in cba which is not same as the original string.
Hence aba is a Palindrome while abc isn’t.
How to verify for Palindrome?
To check if a number is a Palindrome number or not, we first take the input of the number and create a copy of the number taken as an input.
We then create a new variable to store the reversed number and initialize it with 0.
Traverse through the number using mod 10 and division by 10 operations and in each loop make sure to add the digit in the reversed number variable*10.
To check for a string, we take a string as input and calculate its length . We also initialize an empty string to store the reverse of the string.
We create a decrementing loop starting from the last index and going to the first and each time concatenate the current reversed string with the new letter obtained.
Pseudo-code to implement Palindrome in Python
Code to implement palindrome checking in python.
Now that you know what Palindromes are and how to deal with them in the case of strings and numbers, let me show you the code for both.
1. Palindrome Implementation: Numbers
Let’s check for palindrome numbers using Python.
2. Palindrome Implementation: Strings
Let’s now check for Palindrome strings in Python
Palindrome Numbers
Palindrome strings.
Congratulations! Today in this tutorial you learned about Palindromes and how to implement them as well! Hope you learned something! Thank you for reading!
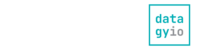
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Python Program to Check If a String is a Palindrome (6 Methods)
- October 6, 2021 July 25, 2023
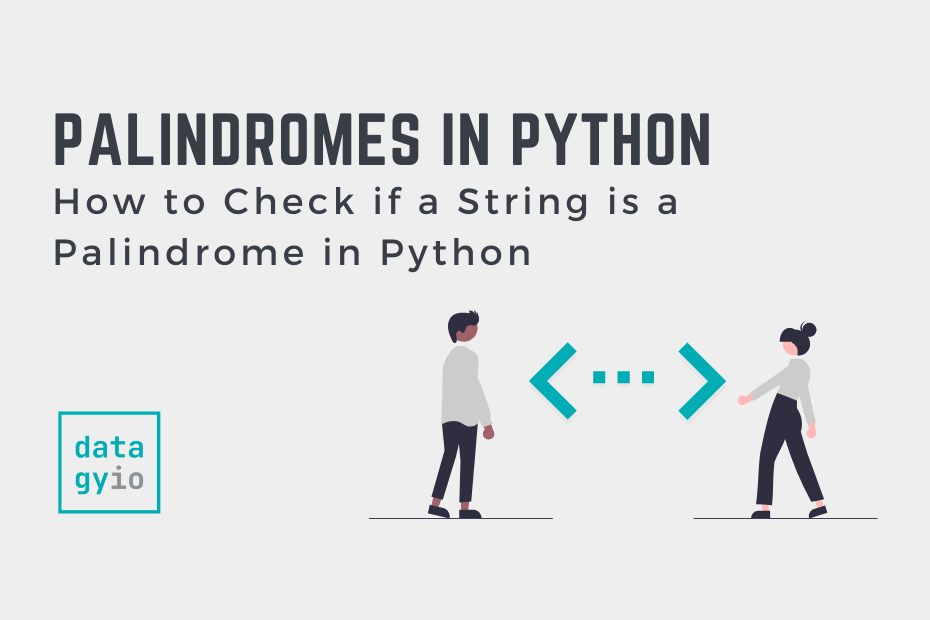
In this tutorial, you’ll learn how to use Python to check if a string is a palindrome . You’ll learn six different ways to check if a string is a palindrome, including using string indexing, for loops, the reversed() function.
You’ll also learn the limitations of the different approaches, and when one might be better to use than another. But before we dive in, let’s answer a quick question:
A palindrome is a word, phrase, or sequence that is the same spelled forward as it is backward .
The Quick Answer: Use String Indexing
Table of Contents
Use Python String Indexing to Check if a String is a Palindrome
String indexing provides the simplest way in Python to check if a string is a palindrome. String indexing can be used to iterate over a string in reverse order.
One of the greatest things about Python indexing is that you can set a step counter as well, meaning you can move over an iterable at the desired rate . The way that we’ll make use of this is to use a step of -1 , meaning that we move over the iterable from the back to the front.
An important thing to keep in mind is that your string may have different casing as well as spaces. Because of this, we’ll need to preprocess our string to remove capitalization and any spaces.
Let’s take a look at using string indexing to check if a string is a palindrome in Python:
Let’s explore what we’ve done in the code above:
- We define a function , palindrome(), that takes a single string as its only parameter
- We then re-assign the string to itself but lower all the character cases (using the .lower() method ) and remove any spaces (using the .replace() method)
- We then evaluate whether or not the modified string is equal to the modified string in reverse order
- This returns True if the string is a palindrome, and False if it is not
Now, let’s take a look at how we can use the Python reversed() function to check if a string is a palindrome.
Use the Python Reversed Function to Check if a String is a Palindrome
Python comes with a built-in function, reversed() , which reverses an iterable item, such as a string. You can pass in some iterable item, be it a string, a list, or anything else ordered, and the function returns the reversed version of it.
Let’s take a look at how we can use the reversed() function:
Let’s take a look at what our function does:
- Similar to the method above, the function first changes all casing to lower case and removes all spaces
- Then, it uses the reverse() function to reverse the string.
- Because the reverse() function returns a reversed object, we need to turn it back into a string. This can be done using the .join() method.
- Finally, the two strings are evaluated against whether or not they’re equal.
In the next section, you’ll learn how to use a for loop to check if a string is a palindrome.
Using a For Loop to Check if a Python String is a Palindrome
You can also use a Python for-loop to loop over a string in reverse order, to see if a string is a palindrome or not.
Let’s see how we can use a for loop to check if a string is a palindrome:
What we’ve done here is traversed the list from the last index, -1 , to its first, 0 . We then assign that value to a string reversed . Finally, we evaluate whether the two strings are equal.
In the next section, you’ll learn how to use a Python while loop to see if a palindrome exists.
Using a Python While Loop to Check if a String is a Palindrome
In this section, let’s explore how to use a Python while loop to see if a string is a palindrome or not.
One of the benefits of this approach is that we don’t actually need to reassign a reversed string , which, if your strings are large, won’t consume much memory.
Let’s see how we can use a Python while loop:
Let’s break down what the code block above is doing:
- We define a function, palindrome() , which accepts a string as its only argument
- We format our string in all lowercase and replace any spaces
- We then create two variables, first and last which are equal to 0 and the length of the list minus 1, respectively
- We then create a while loop, which runs as long as the value of first is less than last
- The loop evaluates if the indexed value of first and last are equal to one another
- If they are, the values are incremented and decremented by 1 , respectively
- If not, the function returns False
A major performance benefit here can be that if a string is clearly not a palindrome, say if the first and last characters don’t match, then the loop breaks. This saves us significant memory and time.
Check if a String is a Palindrome Using Recursion in Python
In this section, you’ll learn how to use recursion to check if a string is a palindrome in Python. Recursive functions can be used to make your code simpler and cleaner while providing extensive functionality.
Let’s take a look at how we can develop a recursive function that checks whether or not a string is a palindrome:
Let’s break down what we did in the code above:
- We defined a function, palindrome() , which takes a string as its only parameter
- The function first lowercases the string and removes any spaces
- Then, it checks if the first and last characters are the same
- If they are not, then the function returns False
- If they are, the function is called recursively, ignoring the first and last letters
In the next section, you’ll learn how to check whether or not a number is a palindrome.
Check if a Number is a Palindrome in Python
The easiest way to check if a number is a Python palindrome is to convert the number to a string and apply any of the methods mentioned above .
Let’s see how we can do this using the string indexing method:
In order to check if a number is a palindrome in Python, we converted the number to a string using the str() method. From there, we can simply check if the resulting string is a palindrome using any of the methods above. In the code example, we used string indexing to reverse the string.
What is the Fastest Way to Check if a String is a Palindrome in Python?
In the code above, you learned six different ways to use Python to check if a string is a palindrome. At this point, you may be wondering what method to use. In many cases, you’ll want to strive for readability and speed.
In this section, we tested the speed of the different methods using a palindrome that is over ten million characters long . Because recursion is generally capped at 1,000 recursive calls, this method is not tested.
The fastest way to check if a string is a palindrome using Python is to use string indexing, which can be up to 70 times faster than using a for loop.
Below, you’ll find the results of the tests:
Method | Execution Time (10,000,000 characters) |
---|---|
String indexing | 22.7 ms |
reversed() Function | 303 ms |
While Loop | 1.02 s |
For Loop | 1.6 s |
The image below breaks down the speed comparison:
In this post, you learned a number of different ways to check if a Python string is a palindrome. You learned how to do this with Python string indexing, the Python reversed() function, both for and while loops. You also learned how to check if a number is a Python palindrome and how to search a larger string for a substring that is a palindrome.
Additional Documentation
To learn more about related topics, check out the tutorials below:
- Python String Contains: Check if a String Contains a Substring
- How to Check if a String is Empty in Python
- How to Concatenate Strings in Python: A Complete Guide
- Python: Count Words in a String or File
- To learn more about the reversed() function, check out the official documentation here .
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
4 thoughts on “Python Program to Check If a String is a Palindrome (6 Methods)”
for palindromic phrases with punctuation (ex: “Eva, can I see bees in a cave?”), I wrote a little something.
pal = input(‘Enter phrase: ‘) stripped = “”.join(x for x in pal if x.isalnum()) if stripped.lower() == stripped[::-1].lower(): print(f'”{pal}” is a palindrome.’) else: print(f'”{pal}” is not a palindrome.’)
This is great! Thanks for sharing, Ryan.
# Using Recursion to Check if a String is a Palindrome in Python It’s throwing out of Index error , I have corrected my self with below code. Can you please correct your code if below is not accurate one?
a_string = ‘Was it a car or a cat I saw’
def palindrome(a_string): a_string = a_string.lower().replace(‘ ‘, ”) if a_string[0] != a_string[-1]: return False elif (len(a_string) == 1): return True else: return palindrome(a_string[1:-1])
print(palindrome(a_string))
Thanks Ashok! I have corrected the code with your version. I appreciate it!
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

Palindrome Program In Python
In this article, you will learn how to create a palindrome program in python using multiple different methods for both strings and numbers.
Table Of Contents
Palindrome Introduction
- Method 1: String Palindrome
Method 2: Reverse String
Method 3: recursive method, method 1: creating new number in reverse order, method 2: convert number to string.

Any series of characters can be considered a palindrome if it is the same when reversed. For example, the following are palindromes:
The concept of a palindrome is used in finding a similar sequence in DNA and RNA.
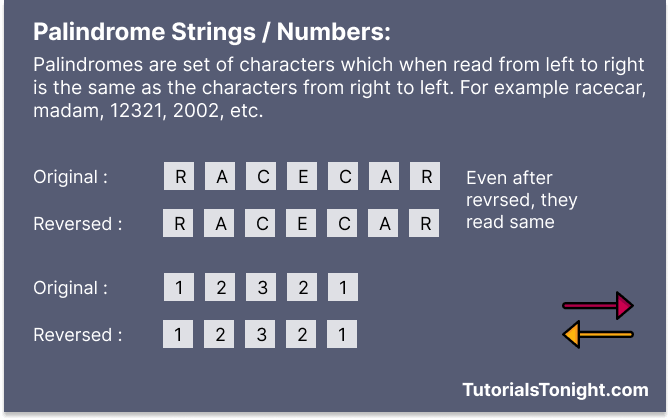
String Palindrome Program In Python
Now you understand palindromes . Let's create Python programs that tell whether a given string is a palindrome or not.
There can be many different methods to check if a string is a palindrome. We are going to discuss 3 different methods in this article.
Method 1: Find String Palindrome
You know that any string which is a palindrome must get the same character either from the left or right side. So we can use this concept and match characters from the left and right sides. If we get a match then we can move to the next character. If we don't get a match then the string is not a palindrome.
For this take 2 variables one starts at 0 and one at the start at the end of the string. We will keep on comparing characters from the left and right sides by moving towards the middle using a while loop in python .
Here is the Python program using this concept:
Code Explanation:
- Start the program by taking input from the user.
- Create a Python function that returns True if the string is a palindrome and False if the string is not a palindrome.
- The isPalindrome() (our function) executes a while loop and checks if characters from the left and right sides are equal. If they are equal then we move to the next character. If they are not equal then the string is not a palindrome.
- Use the python if-else statement to check and print results accordingly.
Another method could be by reversing the string and then comparing it with the original string. If they are the same, then the string is a palindrome.
- This code is very simple. Python creates the reverse of string by s[::-1] . s[::-1] means taking the string from start to end and -1 means taking the string in reverse order.
- Now we have the reverse of the string and can compare it with the original string. If they are equal then the string is a palindrome.
Another method is to use recursion to check if the string is a palindrome or not. In each iteration, we can pass the string without the first character and last character. If we get a match then we can move to the next character. If we don't get a match then the string is not a palindrome.
- This program uses recursion to solve the problem. The function isPalindrome() checks the length of the string and if it is less than or equal to 1 then it returns True. If the first and last character is not equal then it returns False.
- After that, if non of the above condition is true then it calls itself again by passing string without the first and last character.
- Then the same process is repeated until we get a match or we reach the end of the string.
Number Palindrome Program In Python
We can't directly access individual digits of a number. So we need to use some logic to access individual digits of a number.
Look at these methods to find if a number is palindrome or not:
In this method, we will create a new number by reversing the position of digits of the original number.
- The function isPalindrome() checks if the number is less than 0 then it returns False. Because a negative number is not a palindrome.
- Now create a temporary variable and assign a number to it. Also, create a variable to store the reverse of the number.
- Now Execute a while loop and check if the temporary variable is greater than 0. If it is then we can continue.
- In each iteration, multiple reverse variables by 10 and add the remainder of the temporary variable to the reverse variable.
- After that, we divide the temporary variable by 10 to get the next digit.
- If the number and reverse variable are equal then it is a palindrome otherwise it is not a palindrome.
Another method is to convert the number to string and then use any of the above discussed methods to check if the number is a palindrome.
In this brief guide, we have discussed how to check if a string or number is a palindrome or not using 5 different methods.
Above we have seen 5 palindrome program in Python using different approaches. Don't stop learning here check these out: Armstrong Number in Python , Factorial Program in Python , Fibonacci Sequence in Python .
Tutorial Playlist
Python tutorial for beginners.
The Best Tips for Learning Python
Top 10 Reason Why You Should Learn Python
How to install python on windows, top 20 python ides in 2024: choosing the best one, a beginner’s guide to python variables, python numbers: integers, floats, complex numbers.
Understanding Python If-Else Statement
Introduction to Python Strings
The basics of python loops, python for loops explained with examples, introduction to python while loop, everything you need to know about python arrays, all you need to know about python list, how to easily implement python sets and dictionaries, tuples in python: a complete guide, everything you need to know about python slicing, python regular expression (regex), learn a to z about python functions, objects and classes in python: create, modify and delete, python oops concept: here's what you need to know, an introduction to python threading, getting started with jupyter network, pycharm tutorial: getting started with pycharm, the best numpy tutorial for beginners, the best python pandas tutorial, an introduction to matplotlib for beginners, the best guide to time series analysis in python, an introduction to scikit-learn: machine learning in python, a beginner's guide to web scraping with python, expressions in python, python django tutorial: the best guide on django framework, 10 cool python project ideas for beginners in 2024, top 20 python automation projects ideas for beginners, how to become a python developer: a complete guide, the best guide for rpa using python, comprehending web development with php vs. python, the best way to learn about box and whisker plot, an interesting guide to visualizing data using python seaborn, the complete guide to data visualization in python, everything you need to know about game designing with pygame in python, python bokeh: what is bokeh, types of graphs and layout, top 155 python interview questions and answers for 2024, the supreme guide to understand the workings of cpython, the best guide to string formatting in python, how to automate an excel sheet in python: all you need to know, how to make a chatbot in python, what is a multiline comment in python, palindrome in python, data structures in python: a comprehensive guide, fibonacci series in python, mastering palindromes in python: a step-by-step guide.
Lesson 48 of 50 By Simplilearn
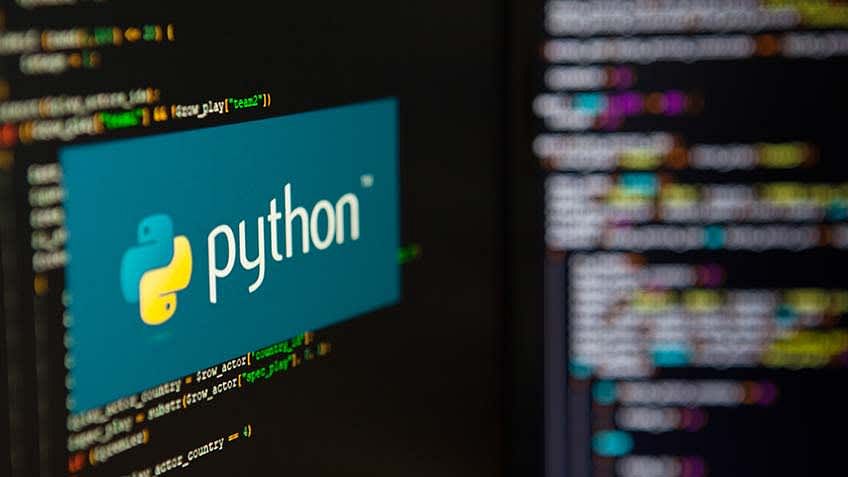
Table of Contents
Growing up and learning computers, we all have found ourselves fascinated by strings of peculiar sequences or numbers. Reading reverse strings was fun when we were little, and as we grew from computer science lovers to coding enthusiasts, we learned that strings that read the same both ways are called palindromes in Python.
Consequently, we took our love for coding further by wanting our machines to understand what palindromes are and how to check them in Python using different ways. Python enthusiasts, let’s admit no other language can do it better.
Want a Top Software Development Job? Start Here!
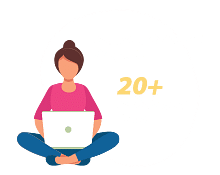
What is a Palindrome?
For a number or string to be palindrome in Python, the number or string must be the same when inverted. If the string or number does not remain unaltered when reversed, it is not a Palindrome.
For instance, 11, 414, 1221, and 123321 are palindrome numbers in Python.
MOM, MADAM, and RADAR are palindrome strings in Python.
Example: KAYAK
Output: Yes, a palindrome string.
Example: 1234321
Output: Yes, a palindrome number.
Therefore, a palindrome in Python is any word, number, sentence, or string that reads the same backward or forward.
Methods to Check Palindrome in Python
A palindrome program in Python allows us to check whether the given string or number is a Palindrome. Moreover, there are several methods in Python to determine this; as a beginner or learner in Python, we have encountered this several times.
Using the reverse and compare method
To use this procedure, which was previously mentioned, we will first look for the reversed string and then compare it to the original string. It is a palindrome if the strings line up. It is as easy as it seems!
#Define a function def isPalindrome(string): if (string == string[::-1]) : return "The string is a palindrome." else: return "The string is not a palindrome." #Enter input string string = input ("Enter string: ") print(isPalindrome(string)) |
Enter string: dad
The string is a palindrome.
The isPalindrome() method is first declared in the code above, and the string argument is passed in.
Then, using the slice operator string[::-1], we get the input string's reverse. The step option, in this case, is -1, which guarantees that the slicing will begin at the end of the string and move back one step each time.
It is now a palindrome if the reversed string equals the input string.
Otherwise, it wouldn't be a palindrome.
Master Web Scraping, Django & More!
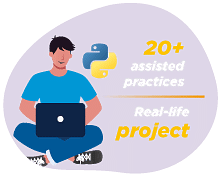
Using inbuilt method
The predefined function "'.join(reversed(string))" is used in this method to reverse the string.
# function to check string is # palindrome or not def isPalindrome(s):
# Using predefined function to # reverse to string print(s) rev = ''.join(reversed(s))
# Checking if both string are # equal or not if (s == rev): return True return False
# main function s = "radar" ans = isPalindrome(s)
if (ans): print("Yes") else: print("No") |
Enter string: radar
The string is palindrome.
In this, by converting the input number to a sequence and utilizing the reversed technique to determine the reverse of the sequence, we can use Python's built-in reversed(sequence) function to check for palindromes. The palindrome can then be verified by comparing these two sequences.
Using for loop
In this method, we'll cycle through each character in the provided string using a for loop, joining it with each element kept in an empty variable we define.
#Enter input string string = input("Enter string : ")
#Declare an empty string variable revstr = ""
#Iterate string with for loop for i in string: revstr = i + revstr print("Reversed string : ", revstr)
if(string == revstr): print("The string is a palindrome.") else: print("The string is not a palindrome.") |
Enter string: malayalam
Reversed string: malayalam
Therefore, a string is referred to as a palindrome if its reverse is the same string.
Using while loop
The most preferred choice among programmers because the string does not need to be reassigned throughout loop execution, a while loop is frequently used over a for loop because it allows the programme to use less memory for longer strings.
#Define a function def isPalindrome(string): string = string.lower().replace(' ', '') first, last = 0, len(string) - 1 while(first < last): if(string[first] == string[last]): first += 1 last -= 1 else: return "The string is not a palindrome."
return "The string is a palindrome."
#Enter input string str1 = input("Enter string : ")
print(isPalindrome(str1)) #Returns True |
Enter string: kayak
Therefore, we begin by declaring the isPalindrome() method and supplying the string parameter in the code above.
Next, two variables, first and last, are defined and given the values 0 and len(string) -1, respectively. We enter an input string in the code above.
The input string is then iterated over all characters from beginning to end using the while loop. Whether the nth index value from the front and the nth index value from the back match will be determined by the loop. The function indicates that the string is a palindrome if True.
Unlike a for loop, this loop ends immediately if the initial and last characters are not the same, and it does not check the full string.
Using the reverse and join method
In this instance, we'll cycle through the string's characters in reverse order using the built-in reversed() method. Next, in order to determine whether the input string is a palindrome, we will compare it with the inverted string. This is similar to the reverse and compare method.
#Define a function def isPalindrome(string): revstr=''.join(reversed(string)) if string==revstr: return "The string is a palindrome." return "The string is not a palindrome."
#Enter input string string = input ("Enter string: ")
print(isPalindrome(string)) |
Enter string: madam
The input string is then passed to the function body, where the reversed() function is used to loop through the string's characters backwards.
The join() function is then used to unite the reversed characters, which are subsequently saved in the revstr variable.
Next, we check to see if the reversed string—a palindrome—matches the input string using the if loop. It is not a palindrome.
Using an iterative loop
In this method, we will run a loop from beginning to length/2, checking the string's initial character from the last one, its second from the second last, and so on. A character mismatch would prevent the string from being a palindrome.
#Define a function def isPalindrome(string): for i in range(int(len(string)/2)): if string[i] != string[len(string)-i-1]: return "The string is not a palindrome." return "The string is a palindrome."
#Enter input string string = input ("Enter string: ")
print(isPalindrome(string)) |
Enter string: tenet
The isPalindrome() function has been declared and the string argument has been supplied to it in the code above.
Next, we execute a for loop from range 0 to the center of the input string in the function body.
We verify if the nth index value from the front and the nth index value from the back match while the for loop executes.
Consequently, the string is NOT a palindrome if they DO NOT match.
If not, the string is a reversed pair.

Dive Deep into Core Python Concepts
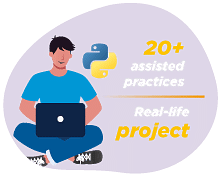
Using recursion
This technique uses the recursion approach, which is a process in which the function calls itself to reverse a string. Then, as in the earlier cases, we verify if the reversed string corresponds to the original string.
#Define a function def isPalindrome(string): if len(string) < 1: return True else: if string[0] == string[-1]: return isPalindrome(string[1:-1]) else: return False
#Enter input string str1 = input("Enter string : ")
if(isPalindrome(str1)==True): print("The string is a palindrome.") else: print("The string is not a palindrome.") |
Enter string: civic
The isPalindrome() method has once more been declared and given a string argument in the code above.
Alternatively, the function is invoked recursively with the parameter as the sliced string with the first and last characters removed, returning False otherwise, if the string's end character matches its beginning character.
Finally, we check whether the returned answer is True or False using an if statement, and then we print the output as indicated.
Hope this text tutorial was able to give you a complete understanding about how to implement Palindrome in Python. If you are looking to enhance your software development skills further, we would highly recommend you to check Simplilearn’s Python Training . This course, in collaboration with IIT Madras, can help you hone the right development skills and make you job-ready in no time.
If you have any questions or queries, please feel free to post them in the comments section below. Our team will get back to you at the earliest.
1. How to do a palindrome function in Python?
There are numerous ways to perform palindromes in Python. We can do this using reverse and compare method, in-built method, for loop, while loop, reverse and join, iterative loop and recursion method.
2. How do you find a palindrome number?
Any number that runs back on itself or remains the same when reversed is a palindrome number. For example, 16461, 11 and 12321.
About the Author
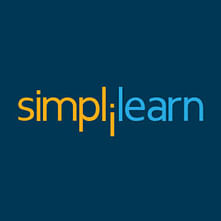
Simplilearn is one of the world’s leading providers of online training for Digital Marketing, Cloud Computing, Project Management, Data Science, IT, Software Development, and many other emerging technologies.
Recommended Programs
Python Training
Full Stack Web Developer - MEAN Stack
*Lifetime access to high-quality, self-paced e-learning content.
Recommended Resources
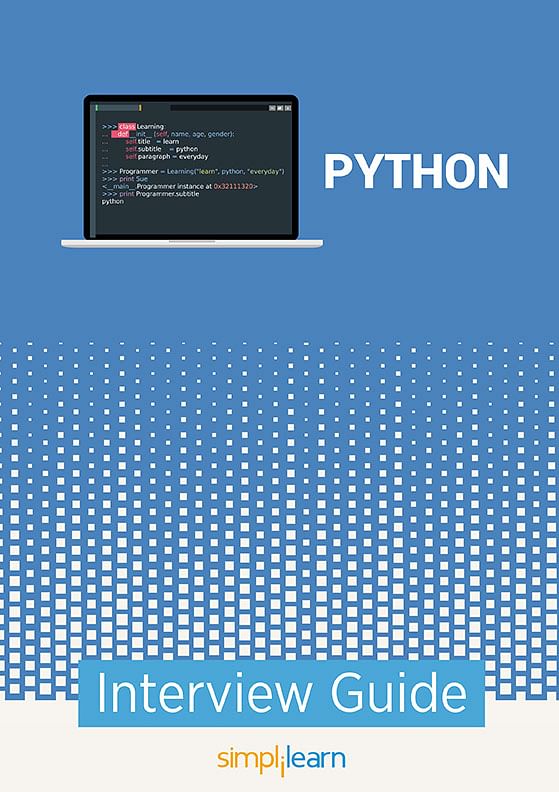
Python Interview Guide
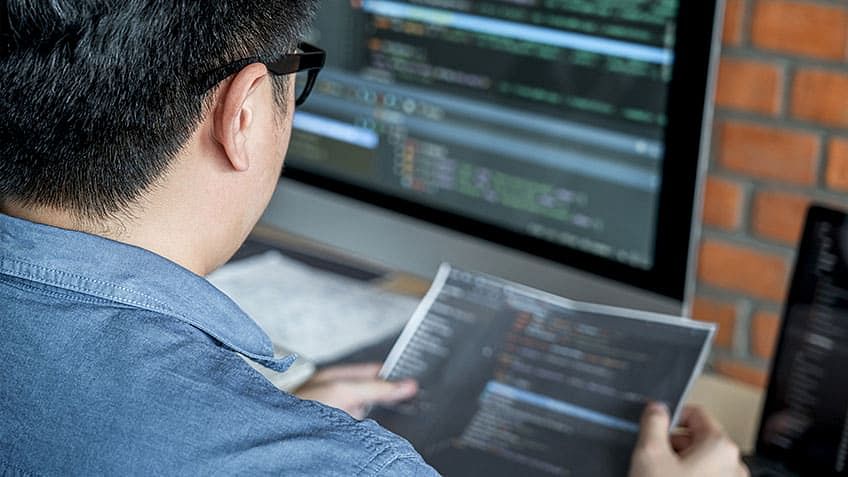
Filter in Python
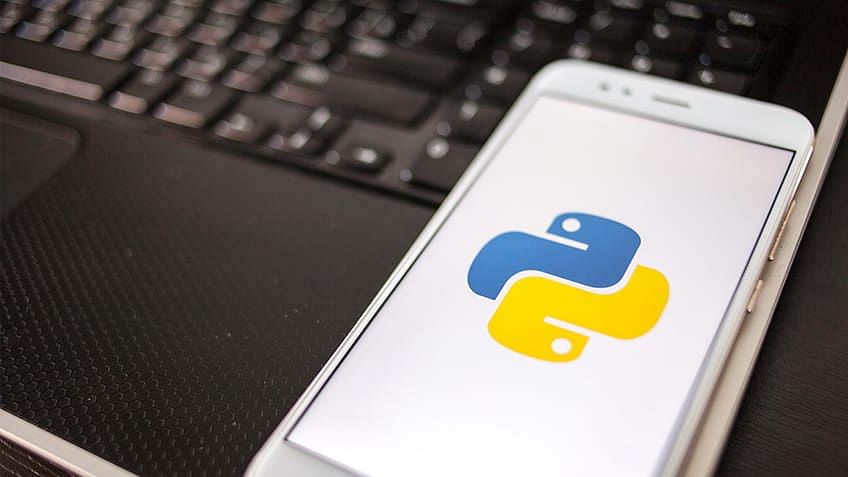
Top Job Roles in the Field of Data Science
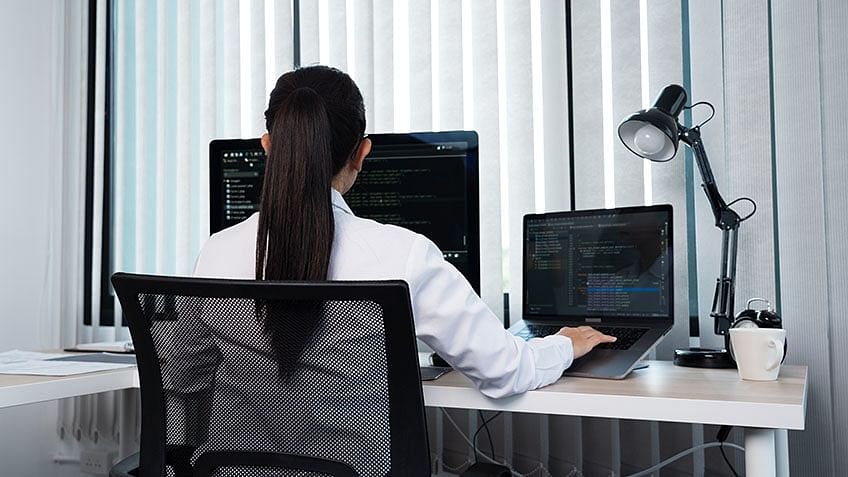
Yield in Python: An Ultimate Tutorial on Yield Keyword in Python
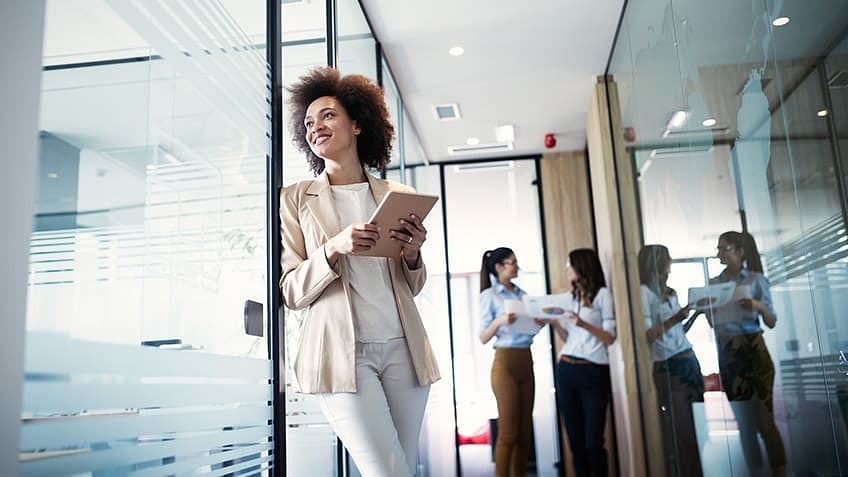
- PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc.
How to Write a Palindrome Checker in Python: A Step-by-Step Guide
A palindrome is a word, phrase, or sequence that reads the same backwards as forwards. Some examples of palindromes include “racecar,” “rotator,” and “nurses run.” Palindromes can make for fun recreational puzzles or programming challenges.
In Python, you can write a function to check if a given string is a palindrome. This how-to guide will walk through the key concepts and steps for writing a palindrome checker program in Python.
Table of Contents
Basic approach, palindrome checker in python, handling empty strings, improving efficiency, ignoring spaces and case, function with cleaner logic, palindrome sentence checker, example code, applications.
What is a Palindrome?
A palindrome is a string that reads the same forward and backward. Some key properties of palindromes:
- The sequence of characters is the same in reverse as forward order.
- Letter case and punctuation marks are ignored.
- Palindromes can be one word, multiple words, sentences, or even numbers.
Some examples of palindromes:
- "A man, a plan, a canal: Panama"
- "Never odd or even"
Palindromes make for interesting recreational puzzles and programming challenges. In Python, we can write a function that takes a string as input and checks if it is a palindrome.
To check for a palindrome, we can:
- Convert the string to lowercase to ignore case.
- Remove any non-alphanumeric characters (punctuation, spaces etc).
- Compare the string with the reversed string.
- If original string is equal to reversed string, then it is a palindrome.
The general logic in pseudocode:
Next, let’s translate this logic into actual Python code.
Here is an implementation of a palindrome checker function in Python:
Let’s break down what this code is doing:
First we import the re module to use regular expressions.
Define a function named is_palindrome() that accepts a string.
Use the regex \W+ to remove any non-alphanumeric characters from the string.
\W matches any non-alphanumeric character.
- matches one or more occurrences of the pattern.
re.sub() replaces these characters with an empty string to remove them.
Convert the cleaned string to lowercase using string.lower() .
Reverse the string using string slicing string[::-1] . This is a handy trick in Python.
Compare the original and reversed string using the equality operator == .
Based on the comparison, return True if it is a palindrome, False if not.
Let’s test it out on some example strings:
Our palindrome checker correctly identifies these strings!
One edge case to consider is handling empty strings or strings with only one character. An empty string can technically be considered a palindrome since it reads the same forwards and backwards.
We can update our function to handle this:
Now empty strings and single character strings will be identified as palindromes.
Our current logic works fine, but it is not very efficient since we slice the string to reverse it.
Slicing creates a new string, which takes up additional memory. Instead, we can reverse the string in-place without slicing by using a for loop and swapping indices.
Here is an implementation with a more optimized approach:
- Initialize indexes pointing to both ends of the string.
- Use a while loop to increment inwards, comparing characters at opposite indices.
- If any pair of characters don’t match, return False.
- If loop terminates with no mismatch, return True.
This implements a two-pointer technique that reverses the string in-place without slicing.
Currently our function only ignores non-alphanumeric characters. We can update it to also ignore spaces and case-sensitivity.
Here’s one way to do this:
- Lowercase the string
- Remove spaces using string.replace(" ", "")
- Same two-pointer comparison logic
Now our function will properly identify palindromes regardless of case or extra spaces.
By breaking the processing and comparison steps into separate helper functions, we can improve modularity and clean up the main function logic:
- clean_string() preprocesses the input string.
- reverse_string() handles reversing the string.
- is_palindrome() handles the main logic and comparisons.
This makes each step more modular and readable.
So far our examples have looked only at single word palindromes. To check for palindrome sentences, we need to compare entire phrases as well as account for spaces between words.
Here is one approach:
- Split the sentence into words
- Reverse each word separately
- Join reversed words back with spaces
- Compare cleaned original vs reversed
This allows our palindrome checker to work on phrases with multiple words:
We can also write a recursive palindrome checker function in Python. The recursive approach is:
- Base case - Empty string or one character string is palindrome.
- Recursion - Check first and last char, if equal call function on substring with first and last char removed.
- Build string back up recursively.
Here is an implementation:
- Base case handles empty or single char string.
- Recursive case slices off first and last char.
- Calls function again on substring without those chars.
Let’s test it:
The recursive approach can be slower due to repeated string slicing and function calls. But it provides an alternative, elegant palindrome checking solution.
In this guide, we learned several methods to check if a string is a palindrome in Python:
- Reverse the string explicitly and compare to original.
- Use a two-pointer technique to reverse and compare in-place.
- Handle sentence palindromes by reversing each word.
- Write a recursive palindrome checker.
The key ideas include:
- Preprocessing the string: lowercasing, removing spaces and punctuation.
- Reversing the string either explicitly or in-place.
- Comparing original vs reversed version.
To handle sentence palindromes, split into words, reverse each word, then join and compare.
The recursive approach provides an elegant alternative using string slicing and recursion.
Palindrome checking makes for an interesting programming challenge in Python. The skills used can be applied to other string manipulation tasks as well.
Here is the full palindrome checker code example:
This implements the in-place two-pointer technique to check for palindromes in an efficient manner. Feel free to build upon this base code for your own applications.
Some examples of practical applications and use cases for palindrome checking functions in Python:
Recreational puzzles - Palindromes make for fun recreational brain teasers and puzzles. A palindrome checker can quickly identify valid palindromes.
Word games - Palindromes are sometimes used in word games like Scrabble or hangman. A program to find or validate palindromes could assist in gameplay.
String manipulation - The techniques used for palindrome checking can be applied to other string reversal, comparison, and manipulation problems.
Algorithm practice - Implementing a palindrome checker is a good introductory algorithm challenge for new programmers.
Technical interview prep - Palindrome questions are commonly asked during coding interviews for software engineering positions. Practicing these skills prepares candidates for the job hunt.
Code golf - Programming challenge to implement palindrome checker in as few characters as possible, good for experienced coders.
Overall, palindrome checkers make for a fun and enlightening Python programming exercise!
This guide covered a variety of methods and techniques for writing a palindrome checker program in Python. We looked at:
- Palindrome definition and properties
- Basic algorithm logic
- Reversing and comparing strings
- Improving efficiency
- Handling spaces and case
- Cleaner modular function design
- Processing palindrome sentences
- Recursive implementation
You should now have a solid foundational understanding of how to check for palindromes in Python. The skills and concepts can be applied to other string manipulation tasks you encounter as a programmer.
There are always opportunities for extension such as handling unicode characters, adding user interfaces, and more robust input handling. I hope you found this comprehensive guide helpful. Happy Python programming!
- python
- technical-coding-interview
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to check for palindrome using Python logic
I'm trying to check for a palindrome with Python. The code I have is very for -loop intensive.
And it seems to me the biggest mistake people do when going from C to Python is trying to implement C logic using Python, which makes things run slowly, and it's just not making the most of the language.
I see on this website. Search for "C-style for", that Python doesn't have C-style for loops. Might be outdated, but I interpret it to mean Python has its own methods for this.
I've tried looking around, I can't find much up to date (Python 3) advice for this. How can I solve a palindrome challenge in Python, without using the for loop?
I've done this in C in class, but I want to do it in Python, on a personal basis. The problem is from the Euler Project , great site By the way,.
I'm missing a lot of code here. The five hashes are just reminders for myself.
Concrete questions:
In C, I would make a for loop comparing index 0 to index max, and then index 0+1 with max-1, until something something. How to best do this in Python?
My for loop (in in range (999, 100, -1), is this a bad way to do it in Python?
Does anybody have any good advice, or good websites, or resources for people in my position? I'm not a programmer, I don't aspire to be one, I just want to learn enough so that when I write my bachelor's degree thesis (electrical engineering), I don't have to simultaneously LEARN an applicable programming language while trying to obtain good results in the project. "How to go from basic C to great application of Python", that sort of thing.
Any specific bits of code to make a great solution to this problem would also be appreciated, I need to learn good algorithms.. I am envisioning 3 situations. If the value is zero or single digit, if it is of odd length, and if it is of even length. I was planning to write for loops...
PS: The problem is: Find the highest value product of two 3 digit integers that is also a palindrome.

- 1 Related: stackoverflow.com/a/7460573/846892 – Ashwini Chaudhary Commented Jun 26, 2013 at 22:10
- 2 I believe this is ProjectEuler #4. You should be able to find some solutions out there that could introduce you to python. But from the looks of it, your implementation is not a terrible one. Your isPalindrome can be much simpler. You may also want to store all palindromes you find in a list and then sort it to find the highest value. If you just break , you are not guaranteed the highest value palindrome. – wflynny Commented Jun 26, 2013 at 22:10
- 1 All these answers are good, although bear in mind that, as stated, your word/phrase has to be an exact palindrome for them to work, including capitalization, spaces, and punctuation. You'll want to look at methods like .lower() and .translate() to make the case uniform and remove the spaces and punctuation if you want to match cases like "Do geese see God?" – Crowman Commented Jun 26, 2013 at 23:23
- @PaulGriffiths Thank you, in this specific program I am dealing with numbers, but I have seen the .lower() and .upper() functions, .translate() I will look into. Thanks a lot! – DrOnline Commented Jun 27, 2013 at 1:31
- Just to clarify for future visitors to this question. The C style of checking for palindrome would involve a for loop like the following: for(int i=0; i<len(str)/2; i++) if str[i] != str[len(str)-i-1]: return False – Ghos3t Commented Mar 10, 2019 at 8:18
37 Answers 37
A pythonic way to determine if a given value is a palindrome:
Explanation:
- We're checking if the string representation of n equals the reversed string representation of n
- The [::-1] slice takes care of reversing the string
- After that, we compare for equality using ==
- Wow.. can you explain to me what this means? How can that line contain the logic to compare the leftmost value with the rightmost..? – DrOnline Commented Jun 26, 2013 at 22:09
- 8 It doesn't. It simply check that the word is equal to itself reversed. An advantage of python is that it allows you to work at an higher level of abstraction leading to cleaner and more elegant solutions – mariosangiorgio Commented Jun 26, 2013 at 22:11
- 1 @DrOnline I updated my answer. This is the pythonic way to do write the solution, by manipulating the very flexible list data structure provided by the language – Óscar López Commented Jun 26, 2013 at 22:12
- 3 @DrOnline the :: part is called a slice , read all about it in here – Óscar López Commented Jun 26, 2013 at 22:14
- 17 [::-1] is advanced slicing. [a:b:c] means slice from a (inclusive) to b (exclusive) with step size c . – wflynny Commented Jun 26, 2013 at 22:15
An alternative to the rather unintuitive [::-1] syntax is this:
The reversed function returns a reversed sequence of the characters in test .
''.join() joins those characters together again with nothing in between.
- 5 @RichieHindle: I find ''.join(reversed(test)) to be just as unintuitive as [::-1] . The really intuitive behaviour would be if you could write test == reversed(test) . (I'm not the downvoter.) – Benjamin Hodgson Commented Jun 26, 2013 at 23:00
- 6 You could do list(test) == list(reversed(test)) . – Rob Commented Apr 15, 2016 at 7:08
Just for the record, and for the ones looking for a more algorithmic way to validate if a given string is palindrome, two ways to achieve the same (using while and for loops):
And....the second one:
The awesome part of python is the things you can do with it. You don't have to use indexes for strings.
The following will work (using slices)
What it does is simply reverses n, and checks if they are equal. n[::-1] reverses n (the -1 means to decrement)
"2) My for loop (in in range (999, 100, -1), is this a bad way to do it in Python?"
Regarding the above, you want to use xrange instead of range (because range will create an actual list, while xrange is a fast generator)
My opinions on question 3
I learned C before Python, and I just read the docs, and played around with it using the console. (and by doing Project Euler problems as well :)
- 1 Note that xrange is only needed (and only exists) in Python 2. In Python 3, the regular range behaves much like xrange used to (with a few extra new features, like slicing to get a different range object added). – Blckknght Commented Jun 26, 2013 at 22:20
- 1 No problem! If you want to get some indexes, use xrange . If you want a list and manipulate that list for something else, use range – jh314 Commented Jun 26, 2013 at 22:21
- Thanks Blckknght, I got errors for xrange, was wondering if I had to include a library or something. Good to know! Kinda like raw input and input was merged to input, I see that was a Python 3 change. – DrOnline Commented Jun 26, 2013 at 22:34
Below the code will print 0 if it is Palindrome else it will print -1
Optimized Code
Explaination:
when searching the string the value that is returned is the value of the location that the string starts at.
So when you do word.find(word[::-1]) it finds nepalapen at location 0 and [::-1] reverses nepalapen and it still is nepalapen at location 0 so 0 is returned.
Now when we search for nepalapend and then reverse nepalapend to dnepalapen it renders a FALSE statement nepalapend was reversed to dnepalapen causing the search to fail to find nepalapend resulting in a value of -1 which indicates string not found.
Another method print true if palindrome else print false
output: TRUE
There is also a functional way:

- 3 This has a minor error: "mannam" will give IndexError: string index out of range , as the most inner call is on the null string (as will giving it a null string). if len(word) <= 1: return True solves this issue, although it will consider null strings as palindromes. – TemporalWolf Commented Nov 15, 2016 at 21:42
I know that this question was answered a while ago and i appologize for the intrusion. However,I was working on a way of doing this in python as well and i just thought that i would share the way that i did it in is as follows,
The most pythonic way to do this is indeed using the slicing notation to reverse the string as mentioned already:
In some other occasions though (like technical interviews), you may have to write a "proper" algorithm to find the palindrome. In this case, the following should do the trick:
- Set pointers to the start and end of the string
- Iterate while end exceeds start
- If the character in end and start indices don't match then this is not a palindrome, otherwise keep comparing
- Increase start pointer by 1
- Decrease end pointer by 1
Test Cases:

Here a case insensitive function since all those solutions above are case sensitive.
This function will return a boolean value.
- 3 In Python3.3+, use str.casefold instead – Adam Smith Commented Jan 27, 2015 at 20:36
doing the Watterloo course for python, the same questions is raised as a "Lesseon" find the info here:
http://cscircles.cemc.uwaterloo.ca/13-lists/
being a novice i solved the problem the following way:
The function is called isPalindrome(S) and requires a string "S" . The return value is by default TRUE , to have the initial check on the first if statement.
After that, the for loop runs half the string length to check if the character from string "S" at the position "i" is the same at from the front and from the back. If once this is not the case, the function stops, prints out FALSE and returns false.
If the string has an uppercase or non-alphabetic character then the function converts all characters to lowercase and removes all non-alphabetic characters using regex finally it applies palindrome check recursively:
the output is True for the input above.
There is much easier way I just found. It's only 1 line.

- You could make it a boolean, ie: is_palindrome = word.find(word[::-1]) == 0 . Still +1 – t.m.adam Commented Jul 8, 2017 at 7:06
- Answer using .find was already posted. – gre_gor Commented Dec 7, 2023 at 7:02
You are asking palindrome in python. palindrome can be performed on strings, numbers and lists. However, I just posted a simple code to check palindrome of a string.
Assuming a string 's'
You can use Deques in python to check palindrome
def palindrome(a_string): ch_dequeu = Deque() for ch in a_string: ch_dequeu.add_rear(ch) still_ok = True while ch_dequeu.size() > 1 and still_ok: first = ch_dequeu.remove_front() last = ch_dequeu.remove_rear() if first != last: still_ok = False return still_ok
class Deque: def __init__(self): self.items = [] def is_empty(self): return self.items == [] def add_rear(self, item): self.items.insert(0, item) def add_front(self, item): self.items.append(item) def size(self): return len(self.items) def remove_front(self): return self.items.pop() def remove_rear(self): return self.items.pop(0)
the "algorithmic" way:
There is another way by using functions, if you don't want to use reverse
It looks prettier with recursion!
This is the typical way of writing single line code
I tried using this:
and it worked for a number but I don't know if a string

You could use this one-liner that returns a bool value:
str(x)==str(x)[::-1]
This works both for words and numbers thanks to the type casting...
- This is exactly the same as the accepted answer posted 10 years before. – gre_gor Commented Dec 7, 2023 at 6:28
This method can solve the issue:

maybe you can try this one:
- 1 Welcome to StackOverflow. Your answer appears to be similar to several others that have already been posted, including the accepted response. If you feel your answer is different, please edit it to add detail. – TomG Commented Mar 21, 2016 at 2:59
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python string palindrome or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Why does flow separation cause an increase in pressure drag?
- Can the SLS's mobile launch platform be rotated at the launch complex to keep the rocket on the leeward side of the tower in case of high winds?
- Is the ILIKE Operator in QGIS not working correctly?
- Is the spectrum of Hawking radiation identical to that of thermal radiation?
- Background for the Elkies-Klagsbrun curve of rank 29
- Monte Carlo Simulation for PSK modulation
- Which hash algorithms support binary input of arbitrary bit length?
- Historical U.S. political party "realignments"?
- How specific does the GDPR require you to be when providing personal information to the police?
- Is this screw inside a 2-prong receptacle a possible ground?
- Parody of Fables About Authenticity
- Is there a difference between these two layouts?
- Stuck on Sokoban
- ESTA is not letting me pay
- Has the US said why electing judges is bad in Mexico but good in the US?
- I would like to add 9 months onto a field table that is a date and have it populate with the forecast date 9 months later
- What does "seeing from one end of the world to the other" mean?
- My supervisor wants me to switch to another software/programming language that I am not proficient in. What to do?
- Are there any polls on the opinion about Hamas in the broader Arab or Muslim world?
- Is there a phrase for someone who's really bad at cooking?
- What's the average flight distance on a typical single engine aircraft?
- What's the meaning of "running us off an embankment"?
- In Top, *how* do conjugate homorphisms of groups induce homotopies of classifying maps?
- Does Vexing Bauble counter taxed 0 mana spells?
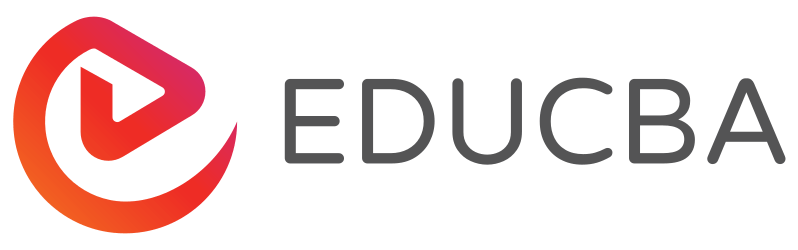
Palindrome in Python
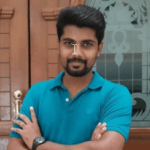
Updated April 17, 2023
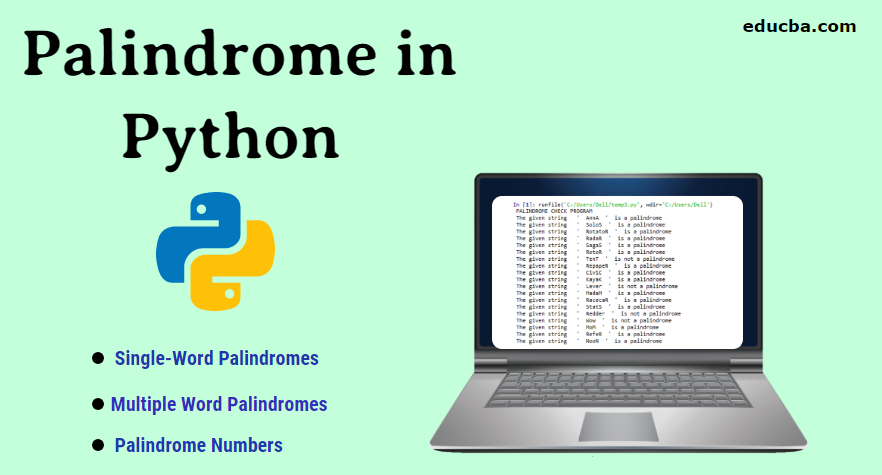
Introduction to Python in Palindrome
A Palindrome is a series of letters, as a word, or a series of numbers, which says the same word when being read both in forward and backward directions. Python Palindrome allows symbols, characters, punctuations and even spaces in the Palindrome words. It functions as a comparison between the actual word, and the reverse of the word that results in an exact match, resulting in the matched value is True. In Python, the palindrome methodology is of three different types: the single word palindrome, the multiple words palindrome, and the number palindrome.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
some among the single word palindromes are listed below,
Types and Techniques Python in Palindrome
Below mentioned are the Types of Python Palindrome.
1. Single-Word Palindromes: Anna, Solos, Rotator, Radar, Sagas, Rotor, Tenet, Repaper, Civic, Kayak, Level, Madam, Racecar, Stats, Redder, Wow, Mom, Refer, Noon
2. Multiple Word Palindromes: Don’t nod, I did, did I?, My gym
3. Palindrome Numbers : 11,66,77,767,454,36763
Technique #1
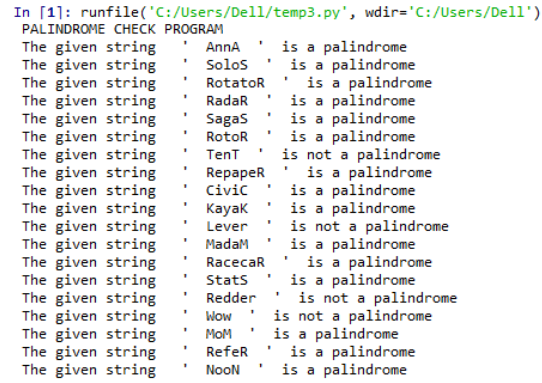
Explanation: This program is implied to check whether the given string is a palindrome or not. Since the input is a string, this check is achieved through the python reverse function . The process flow in the ispalindrome function is as below,
Functions of Python in Palindrome
1) The reverse of the argument of the function is determined and stored in a separate variable. here the reverse is determined using the length technique. The variable’s length is determined, and a manual reverse on top of the length is applied.
2) Then, the variable with reverse stored and the actual variable is compared to check whether they hold the same value.
3) If both are matched, then the value true is returned from the function. In the case both the values don’t make a match, then the value false is returned to the function.
4) So when the value is true, then the message stating “The given string is a palindrome” is printed, instead of when it’s false, then the message stating “the given string is not a palindrome is printed.”
Technique #2

Explanation: As verified for string, the palindrome can also be checked on the numeric values. A palindrome in numeric values also means that the value and it is reverse is the same. Here based on the keyed number, the reverse of the number is generated from the pattern ” str(Number)[::-1], “. and this generated output is compared with the actual value. when the generated value is an exact reverse of the given string, then the output is printed as ” ‘ The given number is PALINDROME ‘ “. In the other case, the output is printed as ” ‘ The given number is NOT a PALINDROME ‘ “.
Technique #3
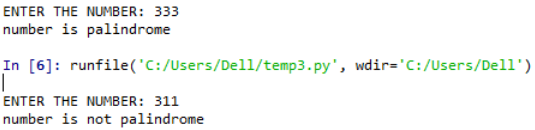
Explanation: This is also a palindrome check program on a numeric value presented. This technique involves reversing the given number using a mathematical formula, and the formula is like below,
Passing the input value to this formula successfully reverses the given integer, and this generated output is compared with the actual value. when the generated value is an exact reverse of the given string, then the output is printed as ” ‘ number is palindrome ‘ “. In the other case, the output is printed as ” ‘ The given number is ” number is not palindrome ‘ “.
These programs are implied to check whether the given string is a palindrome or not. Using the above programs, any given string or a numeric value can be successfully evaluated whether they are a palindrome or not.
Recommended Article
We hope that this EDUCBA information on “Palindrome in Python” was beneficial to you. You can view EDUCBA’s recommended articles for more information.
- Palindrome in C Program
- Palindrome in C++
- Palindrome in PHP
- Palindrome in Java

*Please provide your correct email id. Login details for this Free course will be emailed to you
By signing up, you agree to our Terms of Use and Privacy Policy .
Forgot Password?
This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy
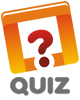
Explore 1000+ varieties of Mock tests View more
Submit Next Question
Early-Bird Offer: ENROLL NOW
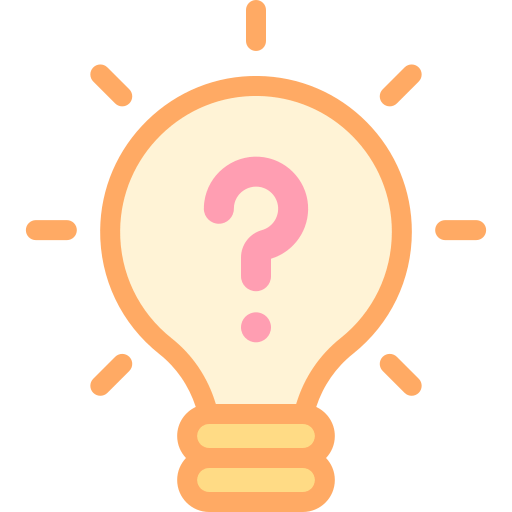
Python Palindromes One-Liner
This one-liner introduces another basic computer science term: palindromes . Similar to anagrams , palindromes are a popular coding interview question.
First things first:
What is a Palindrome?
“A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as madam or racecar or the number 10201.“ [source]
Here are a few fun examples:
- “Mr Owl ate my metal worm”
- “Was it a car or a cat I saw?”
- “Go hang a salami, I’m a lasagna hog”
- “Rats live on no evil star”
Is there a short and concise one-liner solution in Python? (There is!)
But before you and I move on, I’m excited to present you my brand-new Python book Python One-Liners .
If you like one-liners , you’ll LOVE the book. It’ll teach you everything there is to know about a single line of Python code. But it’s also an introduction to computer science , data science, machine learning, and algorithms. The universe in a single line of Python!
More With Less: Buy The Python One-Liner Book
The book is released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Problem Formulation
The problem is the following: Given a sequence of characters (string) – is the reverse sequence of characters the same as the original sequence (that is – is the string a palindrome)?
- Whitespace matters, i.e., 'ann a' is not considered a palindrome, but 'anna' is.
- Capitalization matters, i.e., 'Anna' is not considered a palindrome, but 'anna' is.
Python Palindrome Checker in One Line
Listing: One-liner solution to check whether a phrase is a palindrome.
If you don’t like one-liners and lambda functions, you can also define an explicit function:
Let’s check how this works next.
How the Python Palindrome Checker Works
The one-liner solution does not need any external library, it’s simple and straightforward. We define a lambda function that takes a single argument phrase – the string to be tested – and returns a Boolean whether the sequence of characters remains unchanged when reversed. To reverse the string, we use slicing .
Let’s quickly recap slicing:
💡 Slicing is a Python-specific concept for carving out a range of values from sequence types such as lists or strings . Slicing is based on the concise notation [start:stop:step] to carve out a sequence starting in index “ start ” (inclusive) and ending in index “ end ” (exclusive). The third parameter “ step ” allows you to define the step size, i.e., how many characters from the original sequence your slice will skip before taking the next character (e.g. step=2 means that your slice will consist of only every other character). When using a negative step size, the string is traversed in reverse order.
Thus, the result of the one-liner code snippet is the following:
Python Palindrome Checker Ignoring Whitespace and Capitalization
To check whether two strings are palindromes when ignoring capitalization and arbitrary whitespaces, first bring the string into a canonical form using ''.join (phrase. split() ) and string.lower() to convert it to a lowercase string without whitespaces.
Then use the same expression string == string[::-1] to compare the string with its reverse representation using slicing with negative step size.
Here’s the code example:
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
2 thoughts on “Python Palindromes One-Liner”
“Mr Owl ate my metal worm” If you count blanks as chars too, the above is not a palindrome!
Yeah, you’re right. It’s just an approximate fun palindrome… 🙂 Here’s how you could fix it:
>>> s = “Mr Owl ate my metal worm”.lower().replace(” “,””) >>> print(s == s[::-1]) True
Comments are closed.
- DSA Tutorial
- Data Structures
- Linked List
- Dynamic Programming
- Binary Tree
- Binary Search Tree
- Divide & Conquer
- Mathematical
- Backtracking
- Branch and Bound
- Pattern Searching
Make a palindromic string from given string
Given a string S consisting of lower-case English alphabets only, we have two players playing the game. The rules are as follows:
- The player can remove any character from the given string S and write it on paper on any side(left or right) of an empty string.
- The player wins the game, if at any move he can get a palindromic string first of length > 1.
- If a palindromic string cannot be formed, Player-2 is declared the winner.
Both play optimally with player-1 starting the game. The task is to find the winner of the game.
Examples:
Input: S = “abc” Output: Player-2 Explanation: There are all unique characters due to which there is no way to form a palindromic string of length > 1 Input: S = “abccab” Output: Player-2 Explanation: Initially, newString = “” is empty. Let Player-1 choose character ‘a’ and write it on paper. Then, S = “bccab” and newString = “a”. Now Player-2 chooses character ‘a’ from S and writes it on the left side of newString. Thus, S = “bccb” and newString = “aa”. Now, newString = “aa” is a palindrome of length 2. Hence, Player-2 wins.
Approach: The idea is to formulate a condition in which Player-1 is always going to be the winner. If the condition fails, then Player-2 will win the game.
- If there is only one unique character occurring once in the given string, and the rest of the characters occurring more than 1, then Player-1 is going to be the winner, else Player-2 will always win.
- If we have all characters that are occurring more than once in the given string, then Player-2 can always copy the Player-1 move in his first turn and wins.
- Also, if we have more than one character in the string occurring one time only, then a palindrome string can never be formed(in the optimal case). Hence, again, Player-2 wins.
Below is the implementation of the above approach:
Time Complexity: O(N), where N is the length of the given string. Auxiliary Space: O(1), no extra space is required, so it is a constant.
Please Login to comment...
Similar reads.
- Game Theory
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- Python 3.13 Releases | Enhanced REPL for Developers
- IPTV Anbieter in Deutschland - Top IPTV Anbieter Abonnements
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- How it works
- Homework answers
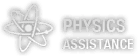
Answer to Question #217495 in Python for sai
Largest Palindrome
You are given an integer
N. Find the largest palindrome which is less than N.Input
The input contains a single integer
The output should be a single integer which is the largest palindrome that is less than the given input.
Explanation
N = 45.44 is the largest number which is a palindrome that is less than 45.
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Write a program to count Vowels and Consonants in string.
- 2. Given a string, write a program to return the sum and average of the digits of all numbers that appe
- 3. Given an integer N as a starting number and K as input, write a python program to print a number pyr
- 4. Build an algorithm to parse and process the dynamic expression based on the dictionaryprovided. The
- 5. Numbers To Words ProblemProgram to convert a given number to Dollar formatWrite code to convert a gi
- 6. Numbers To Words Problem Program to convert a given number to Dollar format Write code to convert
- 7. Trapezium OrderYou are given an integer N. Print N rows starting from 1 in the trapezium order as sh
- Programming
- Engineering
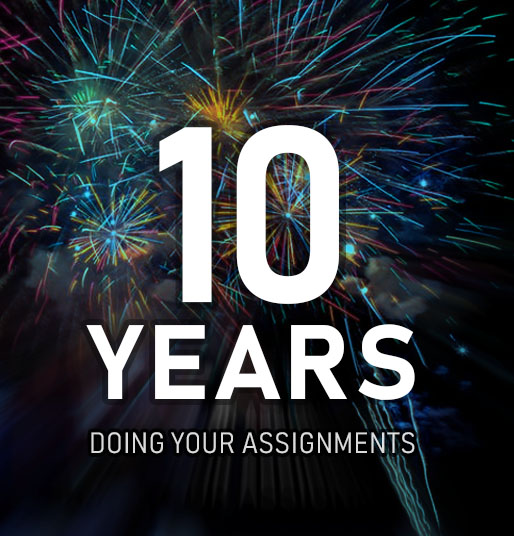
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
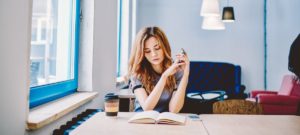
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
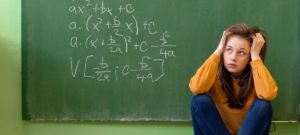
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…

IMAGES
VIDEO
COMMENTS
Answer to Question #231606 in Python for kaavya. You are given a string, write a program to find whether the string is palindrome or not. Note: Treat uppercase letters and lowercase letters as same when comparing letters. Ignore spaces and quotes within the string.Input. The first line of input is a string.
Your physics assignments can be a real challenge, and the due date can be really close — feel free to use our assistance and get the desired result. Physics Be sure that math assignments completed by our experts will be error-free and done according to your instructions specified in the submitted order form.
This tutorial shows you how to reorder a string into a palindrome in Python 3.Be sure to like, comment, and subscribe!0:00 Explaining the algorithm4:17 Imple...
Python. Question #217920. Palindrome Pairs Given a list of unique words, write a program to print all the pairs of the distinct indices (i, j) in the given list, so that the concatenation of two words at indices i and j is a palindrome. Input The input will be a single line containing a sentence. Output The output should be printing each ...
1. Palindrome Numbers. To check if a number is a Palindrome number or not, we first take the input of the number and create a copy of the number taken as an input. We then create a new variable to store the reversed number and initialize it with 0. Traverse through the number using mod 10 and division by 10 operations and in each loop make sure ...
The easiest way to check if a number is a Python palindrome is to convert the number to a string and apply any of the methods mentioned above. Let's see how we can do this using the string indexing method: # Use String Indexing in Python to check if a Number is a Palindrome. a_number = 123454321 def palindrome ( number ):
The concept of a palindrome is used in finding a similar sequence in DNA and RNA. Understanding Palindrome String Palindrome Program In Python. Now you understand palindromes. Let's create Python programs that tell whether a given string is a palindrome or not. There can be many different methods to check if a string is a palindrome.
How to write a Python program to check whether a string is palindrome or not using a stack? Here's a Python program that checks whether a string is a palindrome using a stack: def is_palindrome(s): stack = [] # Push all characters to the stack for char in s: stack.append(char) # Pop characters from the stack and compare with the original string
string = input ("Enter string: ") print (isPalindrome (string)) Enter string: dad. The string is a palindrome. The isPalindrome () method is first declared in the code above, and the string argument is passed in. Then, using the slice operator string [::-1], we get the input string's reverse.
We can also write a recursive palindrome checker function in Python. The recursive approach is: Base case - Empty string or one character string is palindrome. Recursion - Check first and last char, if equal call function on substring with first and last char removed. Build string back up recursively.
207. A pythonic way to determine if a given value is a palindrome: str(n) == str(n)[::-1] Explanation: We're checking if the string representation of n equals the reversed string representation of n. The [::-1] slice takes care of reversing the string. After that, we compare for equality using ==.
Python. Question #231605. Palindrome - 2. You are given a string, write a program to find whether the string is palindrome or not. Note: Treat uppercase letters and lowercase letters as same when comparing letters.Input. The first line of input is a string. Output. The output should be the string. True or False.Explanation.
2) Then, the variable with reverse stored and the actual variable is compared to check whether they hold the same value. 3) If both are matched, then the value true is returned from the function. In the case both the values don't make a match, then the value false is returned to the function. 4) So when the value is true, then the message stating "The given string is a palindrome" is ...
Python One-Liners will teach you how to read and write "one-liners": concise statements of useful functionality packed into a single line of code. You'll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
Answers >. Programming & Computer Science >. Python. Question #194339. Palindrome Pairs. Given a list of unique words, write a program to print all the pairs of the distinct indices (i, j) in the given list, so that the concatenation of two words at indices i and j is a palindrome.
Explanation: There are all unique characters due to which there. is no way to form a palindromic string of length > 1. Input: S = "abccab". Output: Player-2. Explanation: Initially, newString = "" is empty. Let Player-1 choose character 'a' and write it on paper. Then, S = "bccab" and newString = "a".
Palindrome Pairs Sample input Was it car or a cat i saw ... Be sure that math assignments completed by our experts will be error-free and done according to your instructions specified in the submitted order form. ... Programming. Answer to Question #310281 in Python for Palindrome Pairs 2022-03-12T04:56:30-05:00. Answers > Programming ...
Largest Palindrome. You are given an integer . N. Find the largest palindrome which is less than N.Input. The input contains a single integer . N.Output. The output should be a single integer which is the largest palindrome that is less than the given input. Explanation. Given . N = 45.44 is the largest number which is a palindrome that is less ...