Assignation
Global variables.
As in Go lang, you must initially declare your global variable using the := assignment operator and subsequent overwrite use the = operator.
Razor expression | Go Template | Note |
---|---|---|
Global declare and assign of string | ||
Global declare and assign of integer | ||
Global declare and assign of floating point | ||
Global declare and assign of large scientific notation number | ||
Global declare and assign of small scientific notation number | ||
Global declare and assign of hexadecimal number | ||
Global declare and assign of mathematic expression | ||
Global declare and assign of generic expression | ||
Global replacement of previously declared global variable |

Local variables
First declaration of local variable must use the := assignment operator and subsequent assignation must use only = .
There are many form used to declare local variable using razor syntax:
- @{variable} := <value or expression>
- @{variable := <value or expression>}
- @$variable := <value or expression>
Razor expression | Go Template | Note |
---|---|---|
Local declare and assign of string | ||
Local declare and assign of integer | ||
Local declare and assign of floating point | ||
Local declare and assign of large scientific number | ||
Local declare and assign of small scientific number | ||
Local declare and assign of hexadecimal number | ||
Local declare and assign of mathematic expression | ||
Local declare and assign of generic expression | ||
Local replacement of previously declared local variable |
Assignment operators
Using the Razor syntax, it is possible to use assignment operators such as += , /= … The operators that are supported are:
Operator | Assignment | Note |
---|---|---|
Addition | ||
Subtraction | ||
Multiplication | ||
, | , | Division |
Modulo | ||
Bitwise AND | ||
` | ` | ` |
Bitwise XOR | ||
Bit clear | ||
, | , | Left shift |
, | , | Right shift |
Razor expression | Go Template | Note |
---|---|---|
Add assignment operator on global | ||
Add assignment operator on global | ||
Local assignation | ||
Add assignment operator on local | ||
Multiply assignment operator on local | ||
Divide assignment operator on local |
Razor expression | Go Template | Note |
---|---|---|
`@{invalid} := print “hello” “world” | upper` | `{{- $invalid := print }} “hello” “world” |
`@{valid := print “hello” “world” | upper}` | `{{- $valid := print “hello” “world” |
`@($valid := print “hello” “world” | upper)` | `{{- $valid := print “hello” “world” |
Assignation within expression
This package is not in the latest version of its module.
Documentation ¶
- Text and spaces
- Associated templates
- Nested template definitions
Package template implements data-driven templates for generating textual output.
To generate HTML output, see html/template , which has the same interface as this package but automatically secures HTML output against certain attacks.
Templates are executed by applying them to a data structure. Annotations in the template refer to elements of the data structure (typically a field of a struct or a key in a map) to control execution and derive values to be displayed. Execution of the template walks the structure and sets the cursor, represented by a period '.' and called "dot", to the value at the current location in the structure as execution proceeds.
The input text for a template is UTF-8-encoded text in any format. "Actions"--data evaluations or control structures--are delimited by "{{" and "}}"; all text outside actions is copied to the output unchanged.
Once parsed, a template may be executed safely in parallel, although if parallel executions share a Writer the output may be interleaved.
Here is a trivial example that prints "17 items are made of wool".
More intricate examples appear below.
Text and spaces ¶
By default, all text between actions is copied verbatim when the template is executed. For example, the string " items are made of " in the example above appears on standard output when the program is run.
However, to aid in formatting template source code, if an action's left delimiter (by default "{{") is followed immediately by a minus sign and white space, all trailing white space is trimmed from the immediately preceding text. Similarly, if the right delimiter ("}}") is preceded by white space and a minus sign, all leading white space is trimmed from the immediately following text. In these trim markers, the white space must be present: "{{- 3}}" is like "{{3}}" but trims the immediately preceding text, while "{{-3}}" parses as an action containing the number -3.
For instance, when executing the template whose source is
the generated output would be
For this trimming, the definition of white space characters is the same as in Go: space, horizontal tab, carriage return, and newline.
Here is the list of actions. "Arguments" and "pipelines" are evaluations of data, defined in detail in the corresponding sections that follow.
Arguments ¶
An argument is a simple value, denoted by one of the following.
- A boolean, string, character, integer, floating-point, imaginary or complex constant in Go syntax. These behave like Go's untyped constants. Note that, as in Go, whether a large integer constant overflows when assigned or passed to a function can depend on whether the host machine's ints are 32 or 64 bits.
- The keyword nil, representing an untyped Go nil.
- The character '.' (period): . The result is the value of dot.
- A variable name, which is a (possibly empty) alphanumeric string preceded by a dollar sign, such as $piOver2 or $ The result is the value of the variable. Variables are described below.
- The name of a field of the data, which must be a struct, preceded by a period, such as .Field The result is the value of the field. Field invocations may be chained: .Field1.Field2 Fields can also be evaluated on variables, including chaining: $x.Field1.Field2
- The name of a key of the data, which must be a map, preceded by a period, such as .Key The result is the map element value indexed by the key. Key invocations may be chained and combined with fields to any depth: .Field1.Key1.Field2.Key2 Although the key must be an alphanumeric identifier, unlike with field names they do not need to start with an upper case letter. Keys can also be evaluated on variables, including chaining: $x.key1.key2
- The name of a niladic method of the data, preceded by a period, such as .Method The result is the value of invoking the method with dot as the receiver, dot.Method(). Such a method must have one return value (of any type) or two return values, the second of which is an error. If it has two and the returned error is non-nil, execution terminates and an error is returned to the caller as the value of Execute. Method invocations may be chained and combined with fields and keys to any depth: .Field1.Key1.Method1.Field2.Key2.Method2 Methods can also be evaluated on variables, including chaining: $x.Method1.Field
- The name of a niladic function, such as fun The result is the value of invoking the function, fun(). The return types and values behave as in methods. Functions and function names are described below.
- A parenthesized instance of one the above, for grouping. The result may be accessed by a field or map key invocation. print (.F1 arg1) (.F2 arg2) (.StructValuedMethod "arg").Field
Arguments may evaluate to any type; if they are pointers the implementation automatically indirects to the base type when required. If an evaluation yields a function value, such as a function-valued field of a struct, the function is not invoked automatically, but it can be used as a truth value for an if action and the like. To invoke it, use the call function, defined below.
Pipelines ¶
A pipeline is a possibly chained sequence of "commands". A command is a simple value (argument) or a function or method call, possibly with multiple arguments:
A pipeline may be "chained" by separating a sequence of commands with pipeline characters '|'. In a chained pipeline, the result of each command is passed as the last argument of the following command. The output of the final command in the pipeline is the value of the pipeline.
The output of a command will be either one value or two values, the second of which has type error. If that second value is present and evaluates to non-nil, execution terminates and the error is returned to the caller of Execute.
Variables ¶
A pipeline inside an action may initialize a variable to capture the result. The initialization has syntax
where $variable is the name of the variable. An action that declares a variable produces no output.
Variables previously declared can also be assigned, using the syntax
If a "range" action initializes a variable, the variable is set to the successive elements of the iteration. Also, a "range" may declare two variables, separated by a comma:
in which case $index and $element are set to the successive values of the array/slice index or map key and element, respectively. Note that if there is only one variable, it is assigned the element; this is opposite to the convention in Go range clauses.
A variable's scope extends to the "end" action of the control structure ("if", "with", or "range") in which it is declared, or to the end of the template if there is no such control structure. A template invocation does not inherit variables from the point of its invocation.
When execution begins, $ is set to the data argument passed to Execute, that is, to the starting value of dot.
Here are some example one-line templates demonstrating pipelines and variables. All produce the quoted word "output":
Functions ¶
During execution functions are found in two function maps: first in the template, then in the global function map. By default, no functions are defined in the template but the Funcs method can be used to add them.
Predefined global functions are named as follows.
The boolean functions take any zero value to be false and a non-zero value to be true.
There is also a set of binary comparison operators defined as functions:
For simpler multi-way equality tests, eq (only) accepts two or more arguments and compares the second and subsequent to the first, returning in effect
(Unlike with || in Go, however, eq is a function call and all the arguments will be evaluated.)
The comparison functions work on any values whose type Go defines as comparable. For basic types such as integers, the rules are relaxed: size and exact type are ignored, so any integer value, signed or unsigned, may be compared with any other integer value. (The arithmetic value is compared, not the bit pattern, so all negative integers are less than all unsigned integers.) However, as usual, one may not compare an int with a float32 and so on.
Associated templates ¶
Each template is named by a string specified when it is created. Also, each template is associated with zero or more other templates that it may invoke by name; such associations are transitive and form a name space of templates.
A template may use a template invocation to instantiate another associated template; see the explanation of the "template" action above. The name must be that of a template associated with the template that contains the invocation.
Nested template definitions ¶
When parsing a template, another template may be defined and associated with the template being parsed. Template definitions must appear at the top level of the template, much like global variables in a Go program.
The syntax of such definitions is to surround each template declaration with a "define" and "end" action.
The define action names the template being created by providing a string constant. Here is a simple example:
This defines two templates, T1 and T2, and a third T3 that invokes the other two when it is executed. Finally it invokes T3. If executed this template will produce the text
By construction, a template may reside in only one association. If it's necessary to have a template addressable from multiple associations, the template definition must be parsed multiple times to create distinct *Template values, or must be copied with Template.Clone or Template.AddParseTree .
Parse may be called multiple times to assemble the various associated templates; see ParseFiles , ParseGlob , Template.ParseFiles and Template.ParseGlob for simple ways to parse related templates stored in files.
A template may be executed directly or through Template.ExecuteTemplate , which executes an associated template identified by name. To invoke our example above, we might write,
or to invoke a particular template explicitly by name,
- func HTMLEscape(w io.Writer, b []byte)
- func HTMLEscapeString(s string) string
- func HTMLEscaper(args ...any) string
- func IsTrue(val any) (truth, ok bool)
- func JSEscape(w io.Writer, b []byte)
- func JSEscapeString(s string) string
- func JSEscaper(args ...any) string
- func URLQueryEscaper(args ...any) string
- type ExecError
- func (e ExecError) Error() string
- func (e ExecError) Unwrap() error
- type FuncMap
- type Template
- func Must(t *Template, err error) *Template
- func New(name string) *Template
- func ParseFS(fsys fs.FS, patterns ...string) (*Template, error)
- func ParseFiles(filenames ...string) (*Template, error)
- func ParseGlob(pattern string) (*Template, error)
- func (t *Template) AddParseTree(name string, tree *parse.Tree) (*Template, error)
- func (t *Template) Clone() (*Template, error)
- func (t *Template) DefinedTemplates() string
- func (t *Template) Delims(left, right string) *Template
- func (t *Template) Execute(wr io.Writer, data any) error
- func (t *Template) ExecuteTemplate(wr io.Writer, name string, data any) error
- func (t *Template) Funcs(funcMap FuncMap) *Template
- func (t *Template) Lookup(name string) *Template
- func (t *Template) Name() string
- func (t *Template) New(name string) *Template
- func (t *Template) Option(opt ...string) *Template
- func (t *Template) Parse(text string) (*Template, error)
- func (t *Template) ParseFS(fsys fs.FS, patterns ...string) (*Template, error)
- func (t *Template) ParseFiles(filenames ...string) (*Template, error)
- func (t *Template) ParseGlob(pattern string) (*Template, error)
- func (t *Template) Templates() []*Template
- Template (Block)
- Template (Func)
- Template (Glob)
- Template (Helpers)
- Template (Share)
Constants ¶
This section is empty.
func HTMLEscape ¶
HTMLEscape writes to w the escaped HTML equivalent of the plain text data b.
func HTMLEscapeString ¶
HTMLEscapeString returns the escaped HTML equivalent of the plain text data s.
func HTMLEscaper ¶
HTMLEscaper returns the escaped HTML equivalent of the textual representation of its arguments.
func IsTrue ¶ added in go1.6
IsTrue reports whether the value is 'true', in the sense of not the zero of its type, and whether the value has a meaningful truth value. This is the definition of truth used by if and other such actions.
func JSEscape ¶
JSEscape writes to w the escaped JavaScript equivalent of the plain text data b.
func JSEscapeString ¶
JSEscapeString returns the escaped JavaScript equivalent of the plain text data s.
func JSEscaper ¶
JSEscaper returns the escaped JavaScript equivalent of the textual representation of its arguments.
func URLQueryEscaper ¶
URLQueryEscaper returns the escaped value of the textual representation of its arguments in a form suitable for embedding in a URL query.
type ExecError ¶ added in go1.6
ExecError is the custom error type returned when Execute has an error evaluating its template. (If a write error occurs, the actual error is returned; it will not be of type ExecError.)
func (ExecError) Error ¶ added in go1.6
Func (execerror) unwrap ¶ added in go1.13, type funcmap ¶.
FuncMap is the type of the map defining the mapping from names to functions. Each function must have either a single return value, or two return values of which the second has type error. In that case, if the second (error) return value evaluates to non-nil during execution, execution terminates and Execute returns that error.
Errors returned by Execute wrap the underlying error; call errors.As to unwrap them.
When template execution invokes a function with an argument list, that list must be assignable to the function's parameter types. Functions meant to apply to arguments of arbitrary type can use parameters of type interface{} or of type reflect.Value . Similarly, functions meant to return a result of arbitrary type can return interface{} or reflect.Value .
type Template ¶
Template is the representation of a parsed template. The *parse.Tree field is exported only for use by html/template and should be treated as unexported by all other clients.
This example demonstrates a custom function to process template text. It installs the strings.Title function and uses it to Make Title Text Look Good In Our Template's Output.
Here we demonstrate loading a set of templates from a directory.
This example demonstrates one way to share some templates and use them in different contexts. In this variant we add multiple driver templates by hand to an existing bundle of templates.
This example demonstrates how to use one group of driver templates with distinct sets of helper templates.
func Must ¶
Must is a helper that wraps a call to a function returning ( *Template , error) and panics if the error is non-nil. It is intended for use in variable initializations such as
New allocates a new, undefined template with the given name.
func ParseFS ¶ added in go1.16
ParseFS is like Template.ParseFiles or Template.ParseGlob but reads from the file system fsys instead of the host operating system's file system. It accepts a list of glob patterns (see path.Match ). (Note that most file names serve as glob patterns matching only themselves.)
func ParseFiles ¶
ParseFiles creates a new Template and parses the template definitions from the named files. The returned template's name will have the base name and parsed contents of the first file. There must be at least one file. If an error occurs, parsing stops and the returned *Template is nil.
When parsing multiple files with the same name in different directories, the last one mentioned will be the one that results. For instance, ParseFiles("a/foo", "b/foo") stores "b/foo" as the template named "foo", while "a/foo" is unavailable.
func ParseGlob ¶
ParseGlob creates a new Template and parses the template definitions from the files identified by the pattern. The files are matched according to the semantics of filepath.Match , and the pattern must match at least one file. The returned template will have the filepath.Base name and (parsed) contents of the first file matched by the pattern. ParseGlob is equivalent to calling ParseFiles with the list of files matched by the pattern.
When parsing multiple files with the same name in different directories, the last one mentioned will be the one that results.
func (*Template) AddParseTree ¶
AddParseTree associates the argument parse tree with the template t, giving it the specified name. If the template has not been defined, this tree becomes its definition. If it has been defined and already has that name, the existing definition is replaced; otherwise a new template is created, defined, and returned.
func (*Template) Clone ¶
Clone returns a duplicate of the template, including all associated templates. The actual representation is not copied, but the name space of associated templates is, so further calls to Template.Parse in the copy will add templates to the copy but not to the original. Clone can be used to prepare common templates and use them with variant definitions for other templates by adding the variants after the clone is made.
func (*Template) DefinedTemplates ¶ added in go1.5
DefinedTemplates returns a string listing the defined templates, prefixed by the string "; defined templates are: ". If there are none, it returns the empty string. For generating an error message here and in html/template .
func (*Template) Delims ¶
Delims sets the action delimiters to the specified strings, to be used in subsequent calls to Template.Parse , Template.ParseFiles , or Template.ParseGlob . Nested template definitions will inherit the settings. An empty delimiter stands for the corresponding default: {{ or }}. The return value is the template, so calls can be chained.
func (*Template) Execute ¶
Execute applies a parsed template to the specified data object, and writes the output to wr. If an error occurs executing the template or writing its output, execution stops, but partial results may already have been written to the output writer. A template may be executed safely in parallel, although if parallel executions share a Writer the output may be interleaved.
If data is a reflect.Value , the template applies to the concrete value that the reflect.Value holds, as in fmt.Print .
func (*Template) ExecuteTemplate ¶
ExecuteTemplate applies the template associated with t that has the given name to the specified data object and writes the output to wr. If an error occurs executing the template or writing its output, execution stops, but partial results may already have been written to the output writer. A template may be executed safely in parallel, although if parallel executions share a Writer the output may be interleaved.
func (*Template) Funcs ¶
Funcs adds the elements of the argument map to the template's function map. It must be called before the template is parsed. It panics if a value in the map is not a function with appropriate return type or if the name cannot be used syntactically as a function in a template. It is legal to overwrite elements of the map. The return value is the template, so calls can be chained.
func (*Template) Lookup ¶
Lookup returns the template with the given name that is associated with t. It returns nil if there is no such template or the template has no definition.
func (*Template) Name ¶
Name returns the name of the template.
func (*Template) New ¶
New allocates a new, undefined template associated with the given one and with the same delimiters. The association, which is transitive, allows one template to invoke another with a {{template}} action.
Because associated templates share underlying data, template construction cannot be done safely in parallel. Once the templates are constructed, they can be executed in parallel.
func (*Template) Option ¶ added in go1.5
Option sets options for the template. Options are described by strings, either a simple string or "key=value". There can be at most one equals sign in an option string. If the option string is unrecognized or otherwise invalid, Option panics.
Known options:
missingkey: Control the behavior during execution if a map is indexed with a key that is not present in the map.
func (*Template) Parse ¶
Parse parses text as a template body for t. Named template definitions ({{define ...}} or {{block ...}} statements) in text define additional templates associated with t and are removed from the definition of t itself.
Templates can be redefined in successive calls to Parse. A template definition with a body containing only white space and comments is considered empty and will not replace an existing template's body. This allows using Parse to add new named template definitions without overwriting the main template body.
func (*Template) ParseFS ¶ added in go1.16
Func (*template) parsefiles ¶.
ParseFiles parses the named files and associates the resulting templates with t. If an error occurs, parsing stops and the returned template is nil; otherwise it is t. There must be at least one file. Since the templates created by ParseFiles are named by the base (see filepath.Base ) names of the argument files, t should usually have the name of one of the (base) names of the files. If it does not, depending on t's contents before calling ParseFiles, t.Execute may fail. In that case use t.ExecuteTemplate to execute a valid template.
func (*Template) ParseGlob ¶
ParseGlob parses the template definitions in the files identified by the pattern and associates the resulting templates with t. The files are matched according to the semantics of filepath.Match , and the pattern must match at least one file. ParseGlob is equivalent to calling Template.ParseFiles with the list of files matched by the pattern.
func (*Template) Templates ¶
Templates returns a slice of defined templates associated with t.
Source Files ¶
- template.go
Directories ¶
Path | Synopsis |
---|---|
Package parse builds parse trees for templates as defined by text/template and html/template. |
Keyboard shortcuts
: This menu | |
: Search site | |
or | : Jump to |
or | : Canonical URL |
Golang Templates Cheatsheet
The Go standard library provides a set of packages to generate output. The text/template package implements templates for generating text output, while the html/template package implements templates for generating HTML output that is safe against certain attacks. Both packages use the same interface but the following examples of the core features are directed towards HTML applications.
Table of Contents
- Parsing and Creating Templates
- Executing Templates
- Template Encoding and HTML
- Template Variables
- Template Actions
- Template Functions
- Template Comparison Functions
- Nested Templates and Layouts
- Templates Calling Functions
Parsing and Creating Templates
Naming templates .
There is no defined file extension for Go templates. One of the most popular is .tmpl supported by vim-go and referenced in the text/template godocs . The extension .gohtml supports syntax highlighting in both Atom and GoSublime editors. Finally analysis of large Go codebases finds that .tpl is often used by developers. While the extension is not important it is still good to be consistent within a project for clarity.
Creating a Template
tpl, err := template.Parse(filename) will get the template at filename and store it in tpl. tpl can then be executed to show the template.
Parsing Multiple Templates
template.ParseFiles(filenames) takes a list of filenames and stores all templates. template.ParseGlob(pattern) will find all templates matching the pattern and store the templates.
Executing Templates
Execute a single template .
Once a template has been parsed there are two options to execute them. A single template tpl can be executed using tpl.Execute(io.Writer, data) . The content of tpl will be written to the io.Writer. Data is an interface passed to the template that will be useable in the template.
Executing a Named Template
tpl.ExecuteTemplate(io.Writer, name, data) works the same as execute but allows for a string name of the template the user wants to execute.
Template Encoding and HTML
Contextual encoding .
Go ’s html/template package does encoding based on the context of the code. As a result, html/template encodes any characters that need encoding to be rendered correctly.
For example the < and > in "<h1>A header!</h1>" will be encoded as <h1>A header!</h1> .
Type template.HTML can be used to skip encoding by telling Go the string is safe. template.HTML("<h1>A Safe header</h1>") will then be <h1>A Safe header</h1> . Using this type with user input is dangerous and leaves the application vulnerable.
The go html/template package is aware of attributes within the template and will encode values differently based on the attribute.
Go templates can also be used with javascript. Structs and maps will be expanded into JSON objects and quotes will be added to strings for use in function parameters and as variable values.
Safe Strings and HTML Comments
The html/template package will remove any comments from a template by default. This can cause issues when comments are necessary such as detecting internet explorer.
We can use the Custom Functions method (Globally) to create a function that returns html preserving comments. Define a function htmlSafe in the FuncMap of the template.
This function takes a string and produces the unaltered HTML code. This function can be used in a template like so to preserve the comments <!--[if IE 6]> and <![endif]--> :
Template Variables
The dot character (.) .
A template variable can be a boolean, string, character, integer, floating-point, imaginary, or complex constant in Go syntax. Data passed to the template can be accessed using dot {{ . }} .
If the data is a complex type then it’s fields can be accessed using the dot with the field name {{ .FieldName }} .
Dots can be chained together if the data contains multiple complex structures. {{ .Struct.StructTwo.Field }}
Variables in Templates
Data passed to the template can be saved in a variable and used throughout the template. {{$number := .}} We use the $number to create a variable then initialize it with the value passed to the template. To use the variable we call it in the template with {{$number}} .
In this example we pass 23 to the template and stored in the $number variable which can be used anywhere in the template
Template Actions
If/else statements .
Go templates support if/else statements like many programming languages. We can use the if statement to check for values, if it doesn’t exist we can use an else value. The empty values are false, 0, any nil pointer or interface value, and any array, slice, map, or string of length zero.
If .Name exists then Hello, Name will be printed (replaced with the name value) otherwise it will print Hello, Anonymous .
Templates also provide the else if statment {{else if .Name2 }} which can be used to evaluate other options after an if.
Removing Whitespace
Adding different values to a template can add various amounts of whitespace. We can either change our template to better handle it, by ignoring or minimizing effects, or we can use the minus sign - within out template.
<h1>Hello, {{if .Name}} {{.Name}} {{- else}} Anonymous {{- end}}!</h1>
Here we are telling the template to remove all spaces between the Name variable and whatever comes after it. We are doing the same with the end keyword. This allows us to have whitespace within the template for easier reading but remove it in production.
Range Blocks
Go templates have a range keyword to iterate over all objects in a structure. Suppose we had the Go structures:
We have an Item, with a name and price, then a ViewData which is the structure sent to the template. Consider the template containing the following:
For each Item in the range of Items (in the ViewData structure) get the Name and Price of that item and create html for each Item automatically. Within a range each Item becomes the {{.}} and the item properties therefore become {{.Name}} or {{.Price}} in this example.
Template Functions
The template package provides a list of predefined global functions. Below are some of the most used.
Indexing structures in Templates
If the data passed to the template is a map, slice, or array it can be indexed from the template. We use {{index x number}} where index is the keyword, x is the data and number is a integer for the index value. If we had {{index names 2}} it is equivalent to names[2] . We can add more integers to index deeper into data. {{index names 2 3 4}} is equivalent to names[2][3][4] .
This code example passes a person structure and gets the 3rd favourite number from the FavNums slice.
The and Function
The and function returns the boolean AND of its arguments by returning the first empty argument or the last argument. and x y behaves logically as if x then y else x . Consider the following go code
Pass a ViewData with a User that has Admin set true to the following template
The result will be You are an admin user! . However if the ViewData did not include a *User object or Admin was set as false then the result will be Access denied! .
The or Function
The or function operates similarly to the and function however will stop at the first true. or x y is equivalent to if x then x else y so y will never be evaluated if x is not empty.
The not Function
The not function returns the boolean negation of the argument.
Template Comparison Functions
Comparisons .
The html/template package provides a variety of functions to do comparisons between operators. The operators may only be basic types or named basic types such as type Temp float32 Remember that template functions take the form {{ function arg1 arg2 }} .
- eq Returns the result of arg1 == arg2
- ne Returns the result of arg1 != arg2
- lt Returns the result of arg1 < arg2
- le Returns the result of arg1 <= arg2
- gt Returns the result of arg1 > arg2
- ge Returns the result of arg1 >= arg2
Of special note eq can be used with two or more arguments by comparing all arguments to the first. {{ eq arg1 arg2 arg3 arg4}} will result in the following logical expression:
arg1==arg2 || arg1==arg3 || arg1==arg4
Nested Templates and Layouts
Nesting templates .
Nested templates can be used for parts of code frequently used across templates, a footer or header for example. Rather than updating each template separately we can use a nested template that all other templates can use. You can define a template as follows:
A template named “footer” is defined which can be used in other templates like so to add the footer template content into the other template:
Passing Variables between Templates
The template action used to include nested templates also allows a second parameter to pass data to the nested template.
We use the same range to loop through Items as before but we pass the name to the header template each time in this simple example.
Creating Layouts
Glob patterns specify sets of filenames with wildcard characters. The template.ParseGlob(pattern string) function will parse all templates that match the string pattern. template.ParseFiles(files...) can also be used with a list of file names.
The templates are named by default based on the base names of the argument files. This mean views/layouts/hello.gohtml will have the name hello.gohtml . If the template has a {{define “templateName”}} within it then that name will be usable.
A specific template can be executed using t.ExecuteTemplate(w, "templateName", nil) . t is an object of type Template, w is type io.Writer such as an http.ResponseWriter , Then there is the name of the template to execute, and finally passing any data to the template, in this case a nil value.
Example main.go file
All .gohtml files are parsed in main. When route / is reached the template defined as bootstrap is executed using the handler function.
Example views/layouts/bootstrap.gohtml file
Templates Calling Functions
Function variables (calling struct methods) .
We can use templates to call the methods of objects in the template to return data. Consider the User struct with the following method.
When a type User has been passed to the template we can then call this method from the template.
The template checks if the User HasPermission for the feature and renders depending on the result.
Function Variables (call)
If the Method HasPermission has to change at times then the Function Variables (Methods) implementation may not fit the design. Instead a HasPermission func(string) bool attribute can be added on the User type. This can then have a function assigned to it at creation.
We need to tell the Go template that we want to call this function so we must change the template from the Function Variables (Methods) implementation to do this. We use the call keyword supplied by the go html/template package. Changing the previous template to use call results in:
Custom Functions
Another way to call functions is to create custom functions with template.FuncMap . This method creates global methods that can be used throughout the entire application. FuncMap has type map[string]interface{} mapping a string, the function name, to a function. The mapped functions must have either a single return value, or two return values where the second has type error.
Here the function to check if a user has permission for a feature is mapped to the string "hasPermission" and stored in the FuncMap. Note that the custom functions must be created before calling ParseFiles()
The function could be executed in the template as follows:
The .User and string "feature-a" are both passed to hasPermission as arguments.
Custom Functions (Globally)
The previous two methods of custom functions rely on .User being passed to the template. This works in many cases but in a large application passing too many objects to a template can become difficult to maintain across many templates. We can change the implementation of the custom function to work without the .User being passed.
Using a similar feature example as the other 2 sections first you would have to create a default hasPermission function and define it in the template’s function map.
This function could be placed in main() or somewhere that ensures the default hasPermission is created in the hello.gohtml function map. The default function just returns false but it defines the function and implementation that doesn’t require User .
Next a closure could be used to redefine the hasPermission function. It would use the User data available when it is created in a handler rather than having User data passed to it. Within the handler for the template you can redefine any functions to use the information available.
In this handler a User is created with ID and Email, Then a ViewData is created without passing the user to it. The hasPermission function is redefined using user.ID which is available when the function is created. {{if hasPermission "feature-a"}} can be used in a template without having to pass a User to the template as the User object in the handler is used instead.
- Table of Contents
- An Introduction to Templates in Go :
- Previous Article
- Next Article
Template Actions and Nested Templates in Go
This article is part of a series
This is the second part in a four part series where we are learning about creating dynamic HTML and text files with Go’s template packages.
If you haven’t already, I suggest you check out the first part of the series (linked above - look for “Previous Article”). It isn’t absolutely necessary to understand the article, but we created a few source files in that article that will be helpful if you intend to code along.
You can also check out the series overview here: An Introduction to Templates in Go .
And if you are enjoying this series, consider signing up for my mailing list to get notified when I release new articles like it. I promise I don’t spam.
Setting up a Web Server
Up until now we have been outputting templates to the terminal, but as we start to dive into more HTML this starts to make less sense. Instead we want to visualize the HTML being generated in a web browser. To do this we first need to set up a web server that will render our HTML templates.
Open up main.go and update the code to match the code below.
And if you don’t already have it created from the previous article, create a file named hello.gohtml and add the following to it.
Now start the server by typing go run main.go in your terminal. The program should remain running and be listening to web requests on port 3000, so you can view the rendered HTML at localhost:3000 .
if…else Blocks
Our current template is pretty boring as it only prints out a persons name. But what happens if no name is provided? Lets try it out. Open up your main.go file and remove the code inside of your handler() fucntion that creates an instance of ViewData and instead provide nil to the testTemplate.Execute method. Your handler() function should look like this when you are done:
Now restart your server (or let fresh do it) and visit the page in your browser - localhost:3000 . You should see a page that looks something like this.
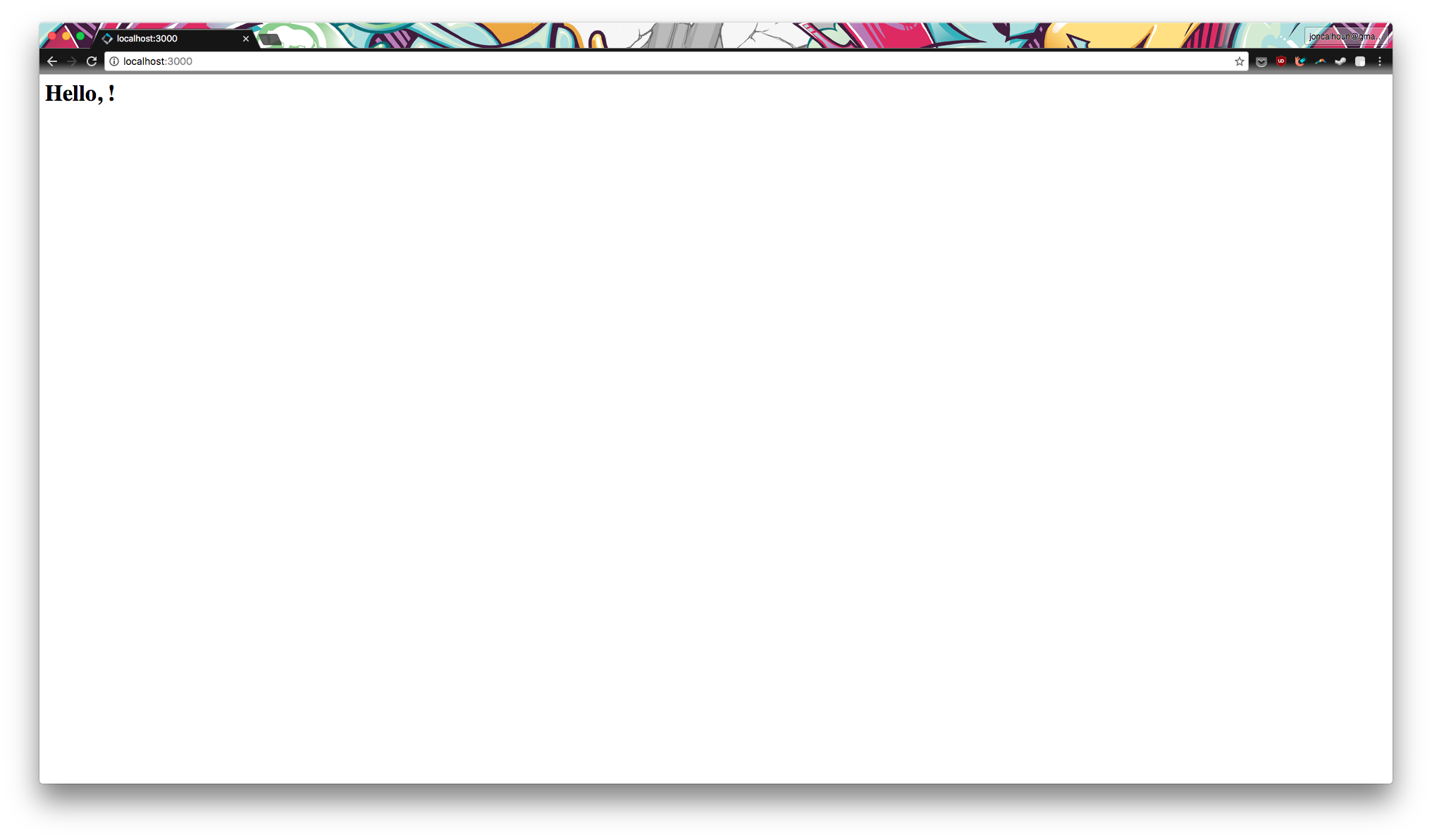
When we don’t provide a name the template renders with an empty string in place of the value. Instead we would rather our page show a more generic string, such as “Hello, there!”. Lets go ahead and update the template to use our first action, an if/else block. Update hello.gohtml like so.
If you check out the page in your browser you should see that this updated your template to show “Hello, there !” like we wanted, but unfortunately this added in an extra space between the word “there” and the exclamation point. This doesn’t matter most of the time, but when dealing with text this can sometimes be annoying. In the next section we will look at two options for cleaning this up a bit.
Trimming Whitespace
To get rid of the extra whitespace we have a couple options:
- Remove them from our template.
- Use the minus sign ( - ) to tell the template package to trim extra whitespace.
The first option is pretty simple. We would just update our hello.gohtml file to and remove extra whitespaces.
In this example this works fine because it is a very short piece of text, but imagine instead that we were generating python code where spacing matters - this could get very annoying very quickly. Luckily the templates package also provide a way to trim unwanted whitespace using the minus character.
In this code snippet we are telling the template package that we don’t want all spaces between the Name variable and whatever comes after it by putting the minus character at the front of the else keyword, and we are also doing the same with the end keyword on the second to last line. Reload your page and you should see that the space is no longer there.
For the rest of this tutorial I am going to opt to use the first example here for my hello.gohtml file.

Range Blocks
Now lets imagine that you wanted to display all of your widgets on your website, along with their price. This is the type of task that dynamic web applications were made for, as nobody wants to manually create HTML for each item you sell and maintain that. Instead we want to use the same HTML for each item. In Go you achieve this using a range block inside of your template.
Update hello.gohtml so that it has the following code.
Then update main.go to have the following code.
If you restart your server (or let fresh do it) and reload the page at localhost:3000 you should now see an HTML page that shows three widgets, each with a title and a price. If we added more widgets to our array we would see more here, and if we instead left it as an empty array we wouldn’t see any widgets here.
The most common source of confusion with the range action is that we are accessing individual attributes of a widget without needing to use an index or any other accessor inside of the .Widgets value. This is because the range action will set the value of each object in the collection to the dot ( . ) inside of the range block. For example, if you were to render {{.}} inside of the range block you would see the same output as if you used fmt.Println() on a Widget object.
Experiment with the templates
Play around with the range block for a bit. Add a line of code like <pre>{{.}}</pre> - what is assigned to the dot attribute? Is it an instance of the Widget struct each pass? What happens if you need the index of each item in the slice as well?
For more on that last bit - getting slice index values - check out the Variables section of the Go template docs and see if you can make their example work with our code.
Nested Templates
As your templates start to grow you will quickly find that you need to reuse components in different places. This is where nested templates come to save the day. With Go’s template package you can declare multiple uniquely named templates, and then when you need to use another template inside of your code you simply reference it using the template keyword. For example, lets say you wanted to declare a footer for your website that you could include on multiple pages and multiple layouts. Add the following footer template to your hello.gohtml file. Where you put it isn’t important, but I prefer to put mine at the top of the file.
Then insert the following line after your range block for the widgets.
Your hello.gohtml file should look like this:
Now if you check out localhost:3000 you will see that the page is using the footer template that you defined. When you define a template you can use it inside of any other template and can even use it multiple times. Try including the footer template twice to see what I mean.
In a later post I will go over how to include multiple files in a template so that you can separate and reuse your templates more easily. For now we are going to go ahead and just put all of the template code in a single file.
Template Variables
Our last example was great, but what happens when you need to include some data in your nested template? Luckily, the template action allows you to pass in a second argument that will be assigned to the dot ( . ) argument inside of the template. For example, lets say we wanted to write a template for the name header portion of a widget, we could do so with the following code.
In this case the .Name attribute is being assigned to the dot ( . ) attribute inside of the widget-header template.
Nested templates with template variables even allow for you to go multiple layers deep, meaning it is possible to call a template from inside of a template.
The end result of this code is the same, but now that we have a widget template we could easily reuse this on other pages of our web application without having to rewrite the code.
Up Next…
With your newly learned templating skills you should be on your way to creating reusable and dynamic templates. In the next article we will go over how to use built-in template functions like and , eq , index , and after that we will look at how to add our own custom functions. I had originally intended to include those hear, but this article had a lot to cover with actions and I didn’t want to sell either short.
Following the post on functions we will go over how to use templates to create the view layer of a web application. This will include creating a shared layout, defining default templates that can be overridden, and including the same templates across various pages without putting all of the code into a single file.
If you are feeling ambitious or curious you can also check out the template docs for text/template and html/template . Go ahead and explore some other actions on your own 👍
This article is part of the series, An Introduction to Templates in Go .
Want to see how templates work in the bigger picture?
In my course - Web Development with Go - we use the html/template package to build out an entire view layer for a realistic application. If you have ever wondered how all of these pieces fit together in the scope of a complete web application, I suggest you check out the course.
If you sign up for my mailing list ( down there ↓ over there → ) I'll send you a FREE sample so you can see if it is for you. The sample includes over 2.5 hours of screencasts and the first few chapters from the book.
You will also receive notifications when I release new articles, updates on upcoming courses (including FREE ones), and I'll let you know when my paid courses are on sale.
Jon Calhoun is a full stack web developer who teaches about Go, web development, algorithms, and anything programming. If you haven't already, you should totally check out his Go courses .
Previously, Jon worked at several statups including co-founding EasyPost, a shipping API used by several fortune 500 companies. Prior to that Jon worked at Google, competed at world finals in programming competitions, and has been programming since he was a child.
More in this series
This post is part of the series, An Introduction to Templates in Go .
Spread the word
Did you find this page helpful? Let others know about it!
Sharing helps me continue to create both free and premium Go resources.
Want to discuss the article?
See something that is wrong, think this article could be improved, or just want to say thanks? I'd love to hear what you have to say!
You can reach me via email or via twitter .
©2018 Jonathan Calhoun. All rights reserved.
Primer on Working with Go Templates

Table of Contents
Introduction.
hugo templates are based on the go language, but are derived from the go template library. It is simple and relies on only basic logic. go templates are html documents whose variables and functions are accessible via {{-}} . For purposes of the blog, the templates are contained in the layout folder.
Curly Brackets
Templates are usually bound to a data structure which is then used to populate the fields within the template. (An old fashioned mail label merge comes to mind, back when we sent mail). The code {{ .FieldName }} denotes the placeholder that the data will replace.
Capital Letters
A name is only exported if it begins with a capital letter. For example, fmt.Println(math.Pi) must have the capital “P” or it will not export 3.14. This is the case when working with templates as well.
Dot Notation
You are never going to understand go without understanding the {{ . }} first. It’s about the scoping rules in go and it allows you to abbreviate how you cite a variable depending on the context. Great article here .
Dollar Notation
Another important symbol is $ . The root context of a template file is stored in $ so the variable can be referenced no matter the depth of the loop or function.
Assignments
Variables are assigned values or declared through the use of := .
Global, pages, parameters, configured or set.
Arrays (slice)
An array, known as a slice in go , is declared as follows:
Associative arrays (maps)
Associative arrays are called “maps”.
Subsetting or Indexing
Iterates over a map, array, or slice. Go and Hugo templates make heavy use of range to iterate over a map, array or slice.
Filters an array to only the elements containing a matching value for a given field.
Loops through any array, slice, or map and returns a string of all the values separated by a delimiter.
Incorporation
Templates in hugo are always in the /layouts folder.
A partial is by definition only part of a template. It must have a context for it to be relevant. In other words, Don’t forget the “{{ . }}" .
_index.md adds front matter and content to list templates. You can keep one _index.md for your homepage and one in each of your content sections, taxonomies, and taxonomy terms.
Lookup Order for templates
Hugo searches for the layout to use for a given page in a well defined order, starting from the most specific.
- declared in frontmatter: layout: "single"
- type in frontmatter: type: "post"
It has a special role in Hugo. It allows you to add front matter and content to your list templates.
A cross-platform Go IDE with extended support for JavaScript, TypeScript, and databases
- Twitter Twitter
- Youtube Youtube
- slack slack
Go templates made easy
Go ships with its own template engine, split into two packages, text/template and html/template . These packages are similar in functionality, with the difference that html/template allows a user to generate HTML code that is safe against code injection, making it suitable for use on web pages and emails. These packages are also used in other applications such as the configuration for Helm , the Kubernetes package manager, assisting with code generation and much more.
Let’s take a look at an example below. Create a project and place the following code in a main.go file:
Then, place the following template code in a new file named index.html :
Now let’s start to add some output to our page so that we can deliver the data to it. Normally you’d start typing something like “ <title>{{. ” and expect the IDE to be smart enough and give you completion options for the options after the dot.
This is where GoLand comes to help us. We can now specify the type beforehand by invoking the “ Specify dot type ” action via Alt + Enter and select the type from the list of types available in the project.
This doesn’t work only for structure fields as the “ Title ” of the page works, but it works for slices, slice elements, and even for elements that are part of a map and are a more complex type.
Besides completion options, once you specify the type of the dot in the template, other functionality such as Navigate to Declaration , Find Usages , or even Rename refactoring will work as the IDE has enough information to complete these actions.
That’s it for today. We learned how we can get better code assistance from the IDE when using the built-in Go template engine and work with it more effectively.
Please let us know your feedback in the comments section below, on Twitter , or on our issue tracker , and tell us what would you like to learn more about in future articles.
Subscribe to GoLang Blog updates
By submitting this form, I agree that JetBrains s.r.o. ("JetBrains") may use my name, email address, and location data to send me newsletters, including commercial communications, and to process my personal data for this purpose. I agree that JetBrains may process said data using third-party services for this purpose in accordance with the JetBrains Privacy Policy . I understand that I can revoke this consent at any time in my profile . In addition, an unsubscribe link is included in each email.
Thanks, we've got you!
Discover more
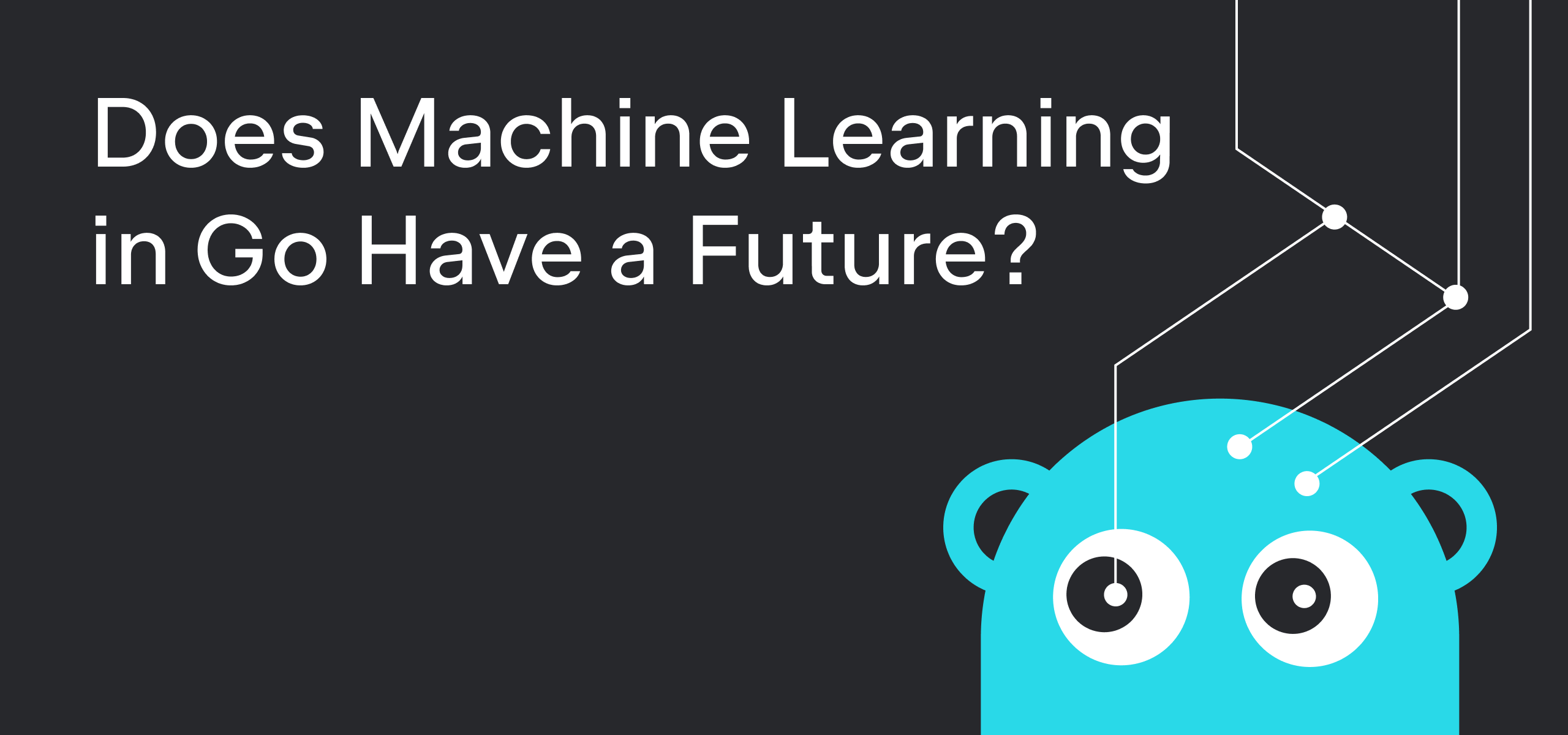
Does Machine Learning in Go Have a Future?
In this article, you'll explore the challenges of actively using Go for Machine Learning.

OS in Go? Why Not?
In this article, you'll learn why languages like C have a stronghold over OS development and whether writing an OS using Go is possible.
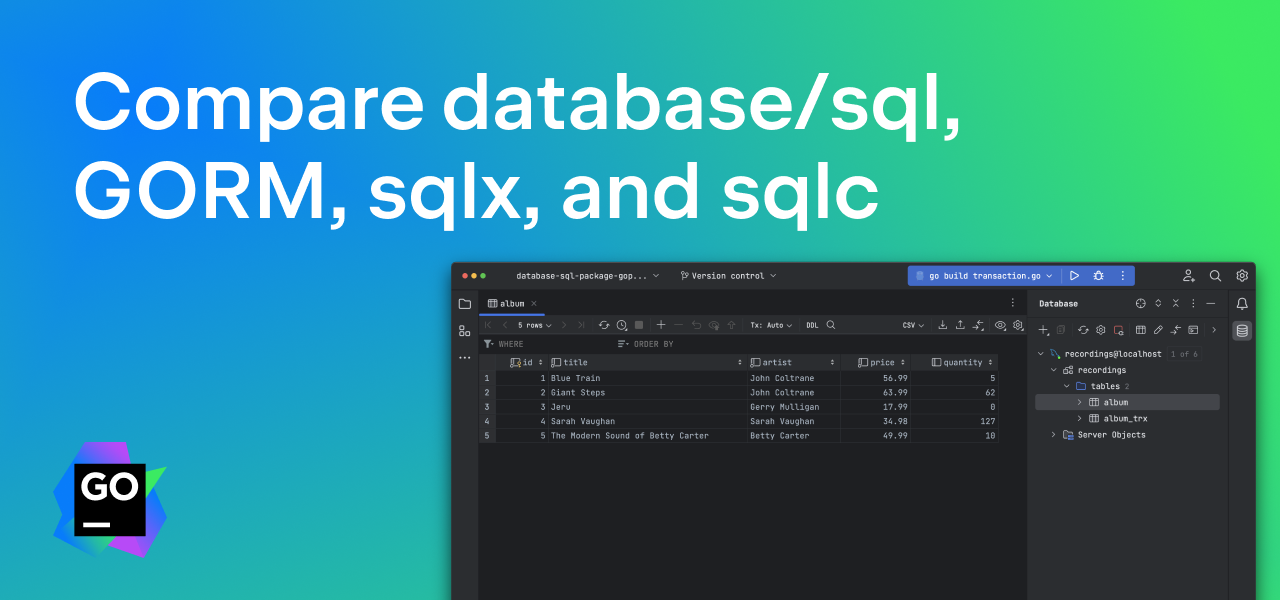
Comparing database/sql, GORM, sqlx, and sqlc
This article compares the database/sql package with 3 other Go packages, namely: sqlx, sqlc, and GORM. The comparison focuses on 3 key areas – features, ease of use, and performance.
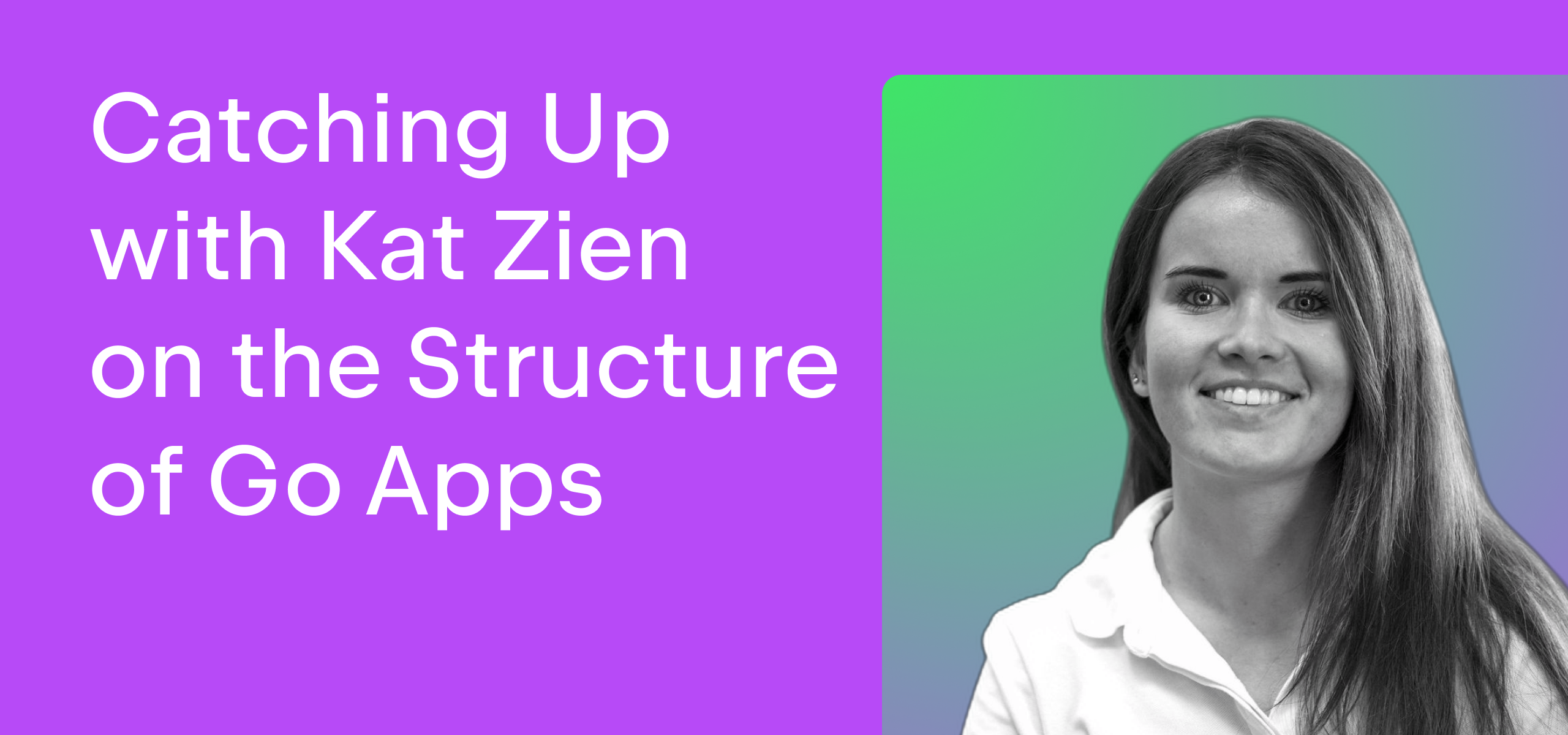
Catching Up With Kat Zien on the Structure of Go Apps in 2023
Let’s say I was asked to build a Go application for a website that hosts raffles.


Member-only story
How To Use Templates In Golang?
An overview of the standard template package.

Jes Fink-Jensen
Better Programming
In this article, I will explain the basics of the standard templating packages of the Go language (Golang). These basics include using variables, conditional statements, looping over variables, and applying functions to variables in the Golang templates.
Golang provides the text/template and html/template packages for handling templates straight out of the box.
The first package is the most general one — You can use it for creating templates for all kinds of text strings. The second package is more specific for HTML — it is handy for dealing with insecure variables in an HTML web environment.
These packages contain various functions that can load, parse, and evaluate template text or (HTML or text) files.
For example, you can use the following functions:
- Use Parse to parse text strings present inside your program.
- Use ParseFiles to load and parse template files.
- Use Execute to render a template to some output using specific data fields.
In the following, I will discuss the basic building blocks for creating powerful templates in Golang.
External (program) variables
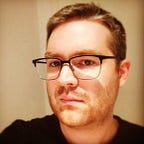
Written by Jes Fink-Jensen
Not your average geek…
Text to speech
francoposa.io
Golang templates, part 1: concepts and composition.
Understanding Golang Template Nesting and Hierarchy With Simple Text Templates
Franco Posa
Template Composition Concepts
Go takes a unique approach to defining, loading, and rendering text and HTML templates.
Common templating libraries such as Jinja, Django Templates, or Liquid have OOP-style template hierarchies where one template inherits from or extends another, forming a clear parent-child relationship. See Jinja2’s template inheritance docs for a basic HTML example.
While the Go template philosophy requires a different mental model, it is quite straightforward and flexible once we push past the initial learning curve.
To understand Go stdlib templates, we need to grasp two design decisions of the library:
- Any template can embed any other template
- The Template type is a recursive collection of Template instances
We will illustrate these concepts with some basic text examples, modified from the Go text/template documentation to provide more insight into the inner workings of the library.
Part 2 of this guide will extend these basics to more practical HTML examples.
The Template Data Structure
As mentioned, Go’s Template is a recursive data type. Each Template instance is itself a collection made up of one or more Template instances. This structure is represented internally by the parse.Tree type.
In this data structure, a child template can be embedded into multiple parent templates. The template libraries handle this internally by copying the child templates.
Introducing cycles into this structure (i.e. T1 embeds T2 , which in turn embeds T1 ), is forbidden and will result in errors when building the template collection.
In our following examples:
- T1 is a Template instance
- T2 is a Template instance
- T3 is a Template instance which embeds the T1 & T2 Template instances
- The top-level collection, named “tmplEx1”, is a Template instance that contains the T1, T2, and T3 Template instances
A rough representation of this template tree might look like this:
From this collection, we will be able to execute any of the individual templates defined in the collection, or the full collection (really the root of the template tree) at once.
Declaring and Invoking Templates
Using define and template.
In Go we declare templates with the define action and “invoke” or evaluate them with the template action.
The code for building and executing the template collections is contained in sections below. For now, we will just focus on understanding the outputs we can expect from given inputs.
In the first version of our example, we declare three templates:
- T1 , containing the word “ONE”
- T2 , containing the word “TWO”
- T3 , which invokes T1 and T2 with a space in between to say “ONE TWO”
Rendering these templates with some helpful print statements will give us the following output:
This looks good, except that executing the full template collection does not output anything. This is because nowhere in our template collection did we invoke a template outside of a template declaration.
We can correct this (if desired) by invoking the t3 template somewhere in our collection, outside of any other template definitions. We will pack the invocation {{template "T3"}} in tight at the end to avoid any unwanted whitespace in our template.
Now we will get the desired output:
Now executing the full template collection has an output.
Using block
We can clean up Example v1.1 with the block action, which combines the define and template actions, declaring the template and invoking it in-place.
The block action requires a pipeline, but this example does not pass in any meaningful data when executing our template - you will notice the calls to Execute just provide an empty string to fill the data parameter.
Pipelines provide ways to access and manipulate the Go data structures which can be passed into templates. Beyond this, pipelines are a topic in and of themselves which will put off in favor of grasping the basic usage of the template package.
We are not concerned with passing any data into the templates in this example. We will just use the standard “dot” ( . ) pipeline, which passes the provided data through to the template unmodified.
We will get the same output as above, without having to worry about tacking on a template invocation without adding whitespace:
Building a Template Collection
Recall that our template tree looks approximately like this:
When building the template collection, you do not need to worry about parsing the templates in reference order. Go’s template packages allow you to add template T3 to the collection before the others.
This is a flexible approach, as the necessary templates could theoretically be created and added to the collection at anytime in the application lifecycle. It also removes the burden from the user of building the collection by walking the tree in the correct order.
Templates are generally built on application startup, which is why we use template.Must to panic if there are any issues with parsing and building the template collection.
Executing Templates
Template collections can be executed as a whole from the root of the tree with the Execute method, or a specific template in the collection can be referenced by name with ExecuteTemplate .
This snippet will execute the templates built in the examples above to produce the expected output:

DEV Community

Posted on Nov 28, 2022
Passing multiple arguments to golang sub-templates
Have you ever wanted to pass multiple arguments to a go sub-template? If you google it you'll be convinced it's not possible. But bear with me.
In go templates you can pass a single "argument" (pipeline in go parlance) to a "sub-template" defined block. But by creating a simple helper function you can pass as many arguments as you want. Simply add this function to your FuncMap :
And you'll be able to create constructs such as:
I named arr my helper func, but you can call it whatever you want.
Top comments (8)
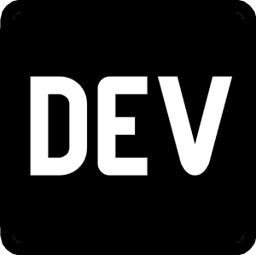
Templates let you quickly answer FAQs or store snippets for re-use.

- Location UK
- Joined Jul 16, 2019
With Go templates it's much more preferable to define a struct containing the data you wish to expose to the template, for example,
and in your template you would have something like,
this provides a more stricter mechanism by which to control what data is in a template, and more clarity too since you would be referring to the data via the field names. Furthermore, it provides some type checking beforehand via the struct's fields.

- Location Rio de Janeiro, BR
- Pronouns she / her
- Joined May 23, 2018
Agreed. But eventually in the rare case when you need a more dynamic approach now you know how.
In the case of having a more dynamic approach I would then prefer map[string]any .
Actually we're talking about different things. The example you showed is related to data sent from Go to the template. My post is about a template calling a sub-template. Imagine an html form builder, where each field is constructed by calling a sub template:
The data sent from Go to the template is a struct (MP3 info), field names have nothing to do with the MP3 data, they're a frontend issue.
In that case I would simply change your implementation to not use an arr function for grabbing arbitrary values and still use a map. See the playground link for an example as to what I mean: go.dev/play/p/5Hiajt4H2Z5
A map function is defined which takes the pairs of values and puts them into a map[string]any , these can then be accessed from the sub-template. This provides more clarity of the data being accessed since the values can be referred to by the index name.

- Joined Apr 2, 2023
This is very helpful thanks for that!

- Joined Jul 7, 2023
Nice! This was really helping me. However, it needs to be: {{ template "MyTemplate" (els "first" 123 .Some.Value) }} {{ template "MyTemplate" (els "second" 456 .Other.Value) }}
In the end I wrote: “I named arr my helper func, but you can call it whatever you want.”, els is the name of the arguments. The name of the function you define on your FuncMap:
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Unlock the Power of Real-Time UI: A Beginner's Guide to Streaming Data with React.js, gRPC, Envoy, and Golang
Dawn Zhao - Aug 8

A Comprehensive Guide to Type Casting and Conversions in Go
zakaria chahboun - Aug 18

Easily manage and install your private Go modules
goproxy.dev - Aug 19

range-over-func in Go
Pallat Anchaleechamaikorn - Aug 18
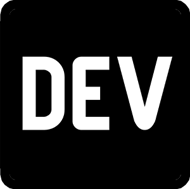
We're a place where coders share, stay up-to-date and grow their careers.
Introduction to templating
A template is a file in the layouts directory of a project, theme, or module. Templates use variables , functions , and methods to transform your content, resources, and data into a published page.
For example, this HTML template initializes the $v1 and $v2 variables, then displays them and their product within an HTML paragraph.
While HTML templates are the most common, you can create templates for any output format including CSV, JSON, RSS, and plain text.
The most important concept to understand before creating a template is context , the data passed into each template. The data may be a simple value, or more commonly objects and associated methods .
For example, a template for a single page receives a Page object, and the Page object provides methods to return values or perform actions.
Current context
Within a template, the dot ( . ) represents the current context.
In the example above the dot represents the Page object, and we call its Title method to return the title as defined in front matter .
The current context may change within a template. For example, at the top of a template the context might be a Page object, but we rebind the context to another value or object within range or with blocks.
In the example above, the context changes as we range through the slice of values. In the first iteration the context is “foo”, and in the second iteration the context is “bar”. Inside of the with block the context is “baz”. Hugo renders the above to:
Template context
Within a range or with block you can access the context passed into the template by prepending a dollar sign ( $ ) to the dot:
Hugo renders this to:
In the examples above the paired opening and closing braces represent the beginning and end of a template action, a data evaluation or control structure within a template.
A template action may contain literal values ( boolean , string , integer , and float ), variables, functions, and methods.
In the example above:
- $convertToLower is a variable
- true is a literal boolean value
- strings.ToLower is a function that converts all characters to lowercase
- Title is a method on a the Page object
Hugo renders the above to:
Notice the blank lines and indentation in the previous example? Although irrelevant in production when you typically minify the output, you can remove the adjacent whitespace by using template action delimiters with hyphens:
Whitespace includes spaces, horizontal tabs, carriage returns, and newlines.
Within a template action you may pipe a value to function or method. The piped value becomes the final argument to the function or method. For example, these are equivalent:
You can pipe the result of one function or method into another. For example, these are equivalent:
These are also equivalent:
Line splitting
You can split a template action over two or more lines. For example, these are equivalent:
You can also split raw string literals over two or more lines. For example, these are equivalent:
A variable is a user-defined identifier prepended with a dollar sign ( $ ), representing a value of any data type, initialized or assigned within a template action. For example, $foo and $bar are variables.
Variables may contain scalars , slices , maps , or objects .
Use := to initialize a variable, and use = to assign a value to a variable that has been previously initialized. For example:
Variables initialized inside of an if , range , or with block are scoped to the block. Variables initialized outside of these blocks are scoped to the template.
With variables that represent a slice or map, use the index function to return the desired value.
With variables that represent a map or object, chain identifiers to return the desired value or to access the desired method.
Used within a template action, a function takes one or more arguments and returns a value. Unlike methods, functions are not associated with an object.
Go’s text/template and html/template packages provide a small set of functions, operators, and statements for general use. See the go-templates section of the function documentation for details.
Hugo provides hundreds of custom functions categorized by namespace. For example, the strings namespace includes these and other functions:
Function | Alias |
---|---|
As shown above, frequently used functions have an alias. Use aliases in your templates to reduce code length.
When calling a function, separate the arguments from the function, and from each other, with a space. For example:
Used within a template action and associated with an object, a method takes zero or more arguments and either returns a value or performs an action.
The most commonly accessed objects are the Page and Site objects. This is a small sampling of the methods available to each object.
Object | Method | Description |
---|---|---|
Returns the date of the given page. | ||
Returns a map of custom parameters as defined in the front matter of the given page. | ||
Returns the title of the given page. | ||
Returns a data structure composed from the files in the data directory. | ||
Returns a map of custom parameters as defined in the site configuration. | ||
Returns the title as defined in the site configuration. |
Chain the method to its object with a dot ( . ) as shown below, remembering that the leading dot represents the current context .
The context passed into most templates is a Page object, so this is equivalent to the previous example:
Some methods take an argument. Separate the argument from the method with a space. For example:
Template comments are similar to template actions. Paired opening and closing braces represent the beginning and end of a comment. For example:
Code within a comment is not parsed, executed, or displayed. Comments may be inline, as shown above, or in block form:
You may not nest one comment inside of another.
To render an HTML comment, pass a string through the safeHTML template function. For example:
Use the template function to include one or more of Hugo’s embedded templates :
Use the partial or partialCached function to include one or more partial templates :
Create your partial templates in the layouts/partials directory.
This limited set of contrived examples demonstrates some of concepts described above. Please see the functions , methods , and templates documentation for specific examples.
Conditional blocks
See documentation for if , else , and end .
Logical operators
See documentation for and and or .
See documentation for range , else , and end .
Use the seq function to loop a specified number of times:
Rebind context
See documentation for with , else , and end .
To test multiple conditions:
Access site parameters
See documentation for the Params method on a Site object.
With this site configuration:
Access the custom site parameters by chaining the identifiers:
Access page parameters
See documentation for the Params method on a Page object.
With this front matter:
Access the custom page parameters by chaining the identifiers:
- Blockquote render hooks
- Code block render hooks
Last updated: August 14, 2024: Document the 'else with' construct introduced with Go 1.23 (41df91659)
Got any suggestions?
We want to hear from you! Send us a message and help improve Slidesgo
Top searches
Trending searches
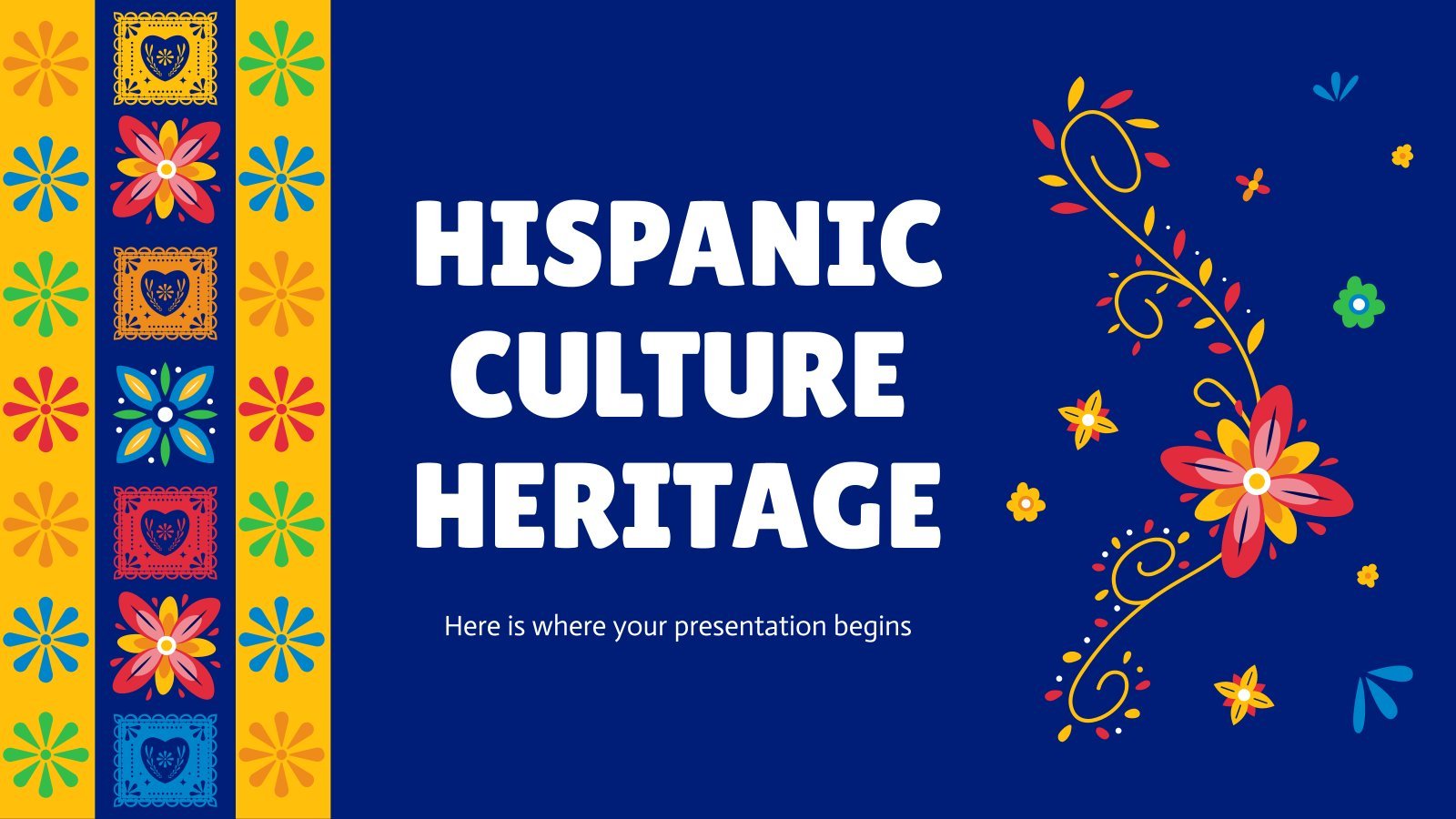
hispanic heritage month
21 templates
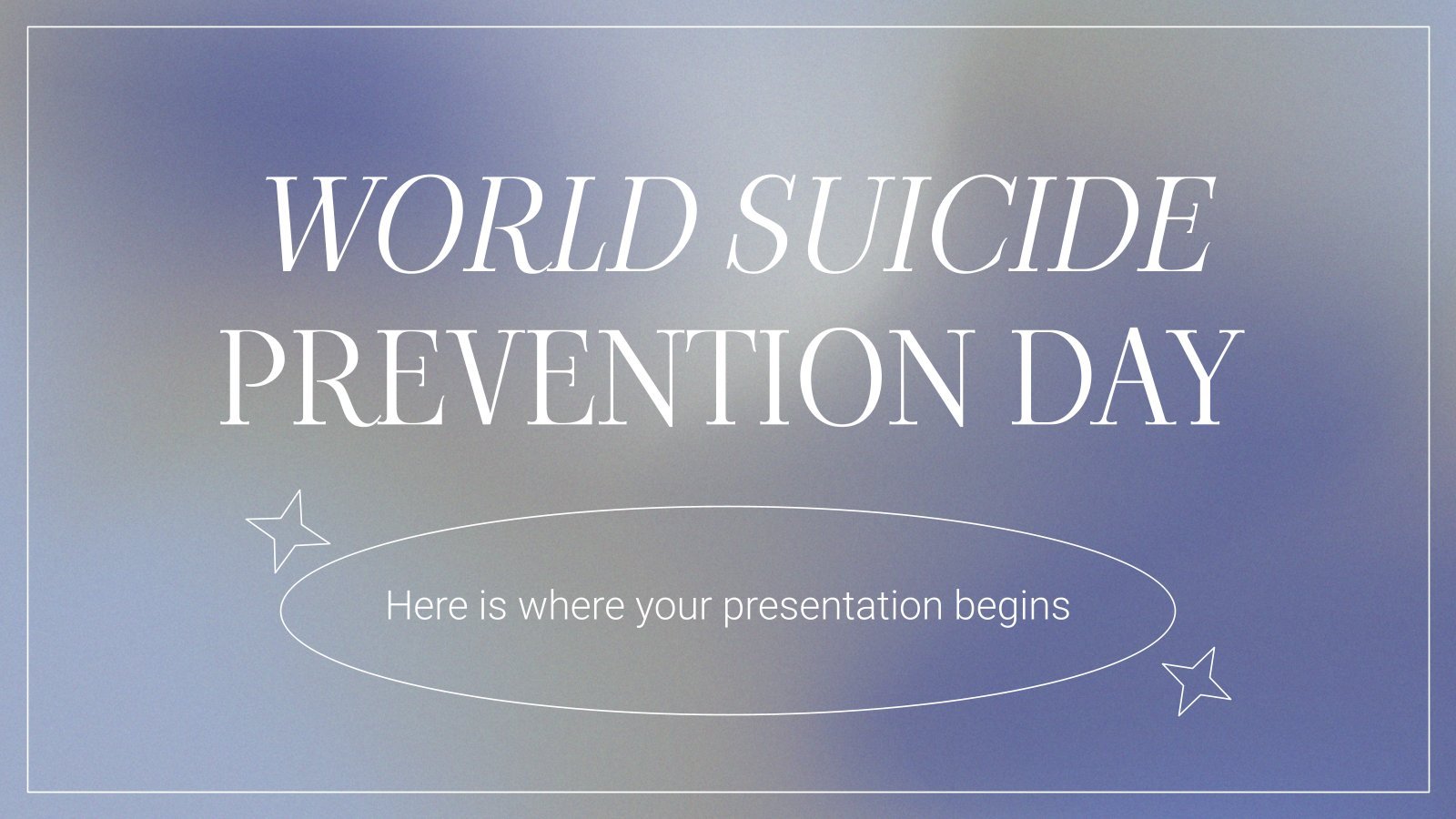
suicide prevention
9 templates
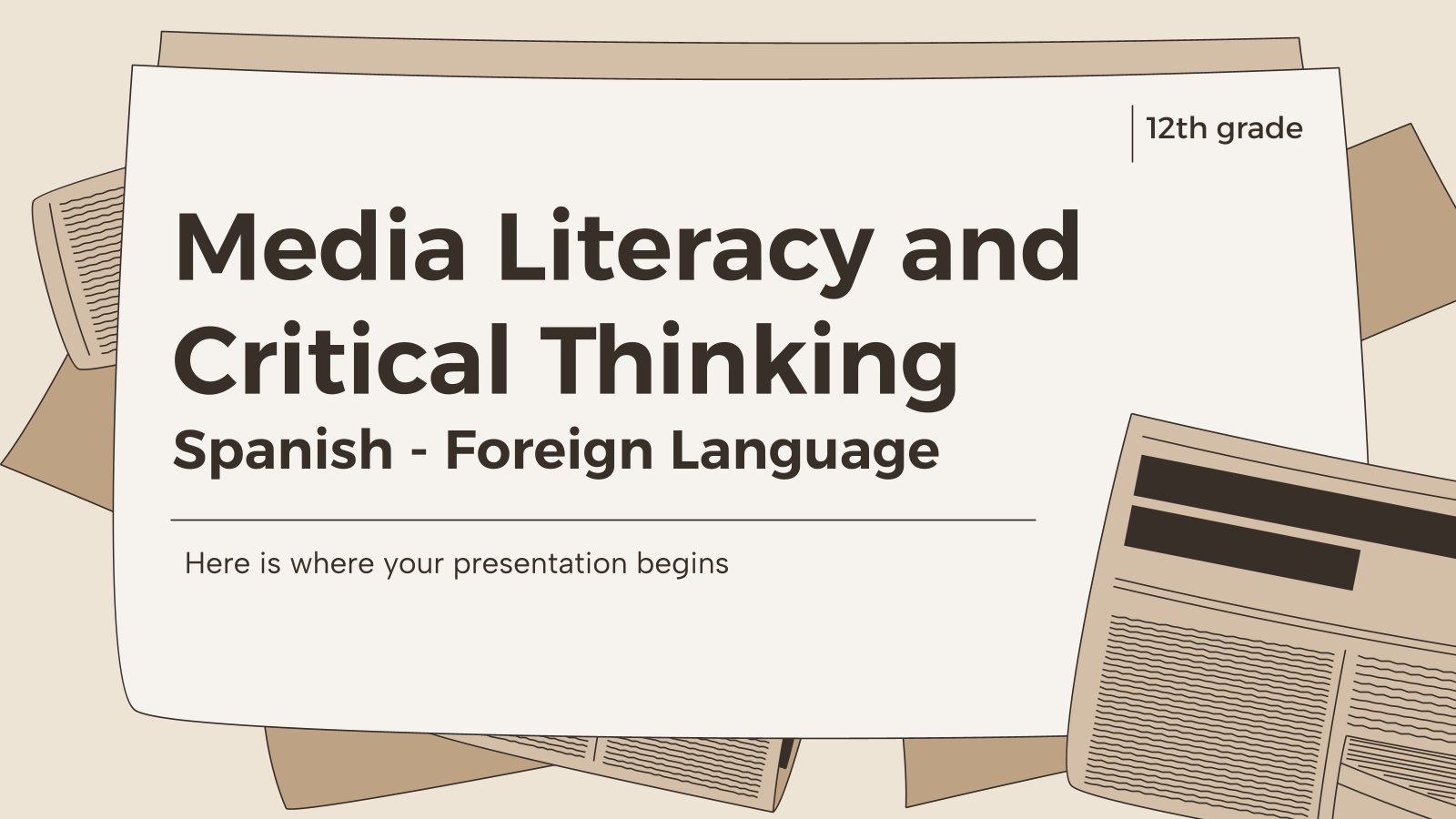
135 templates
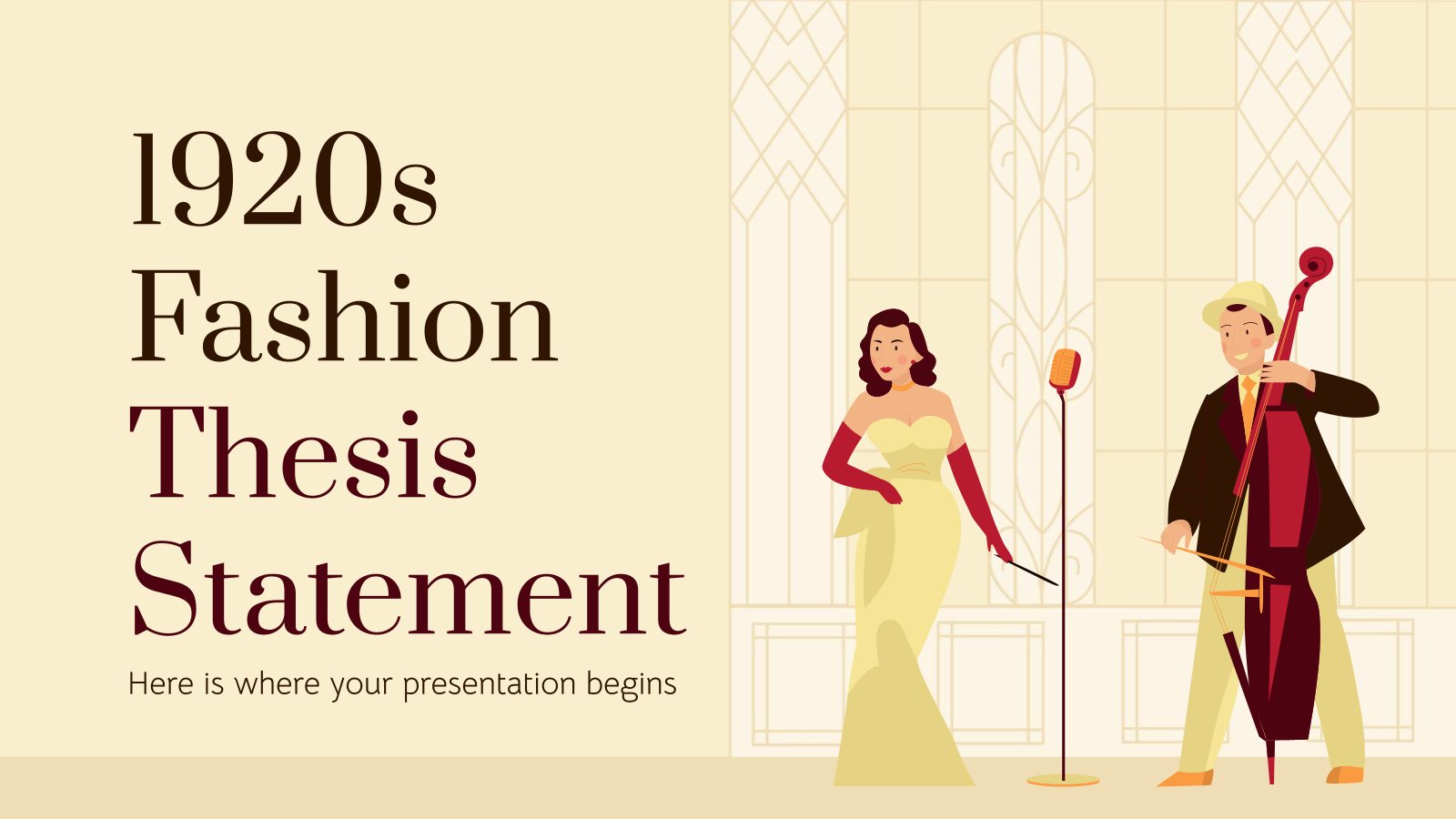
16 templates
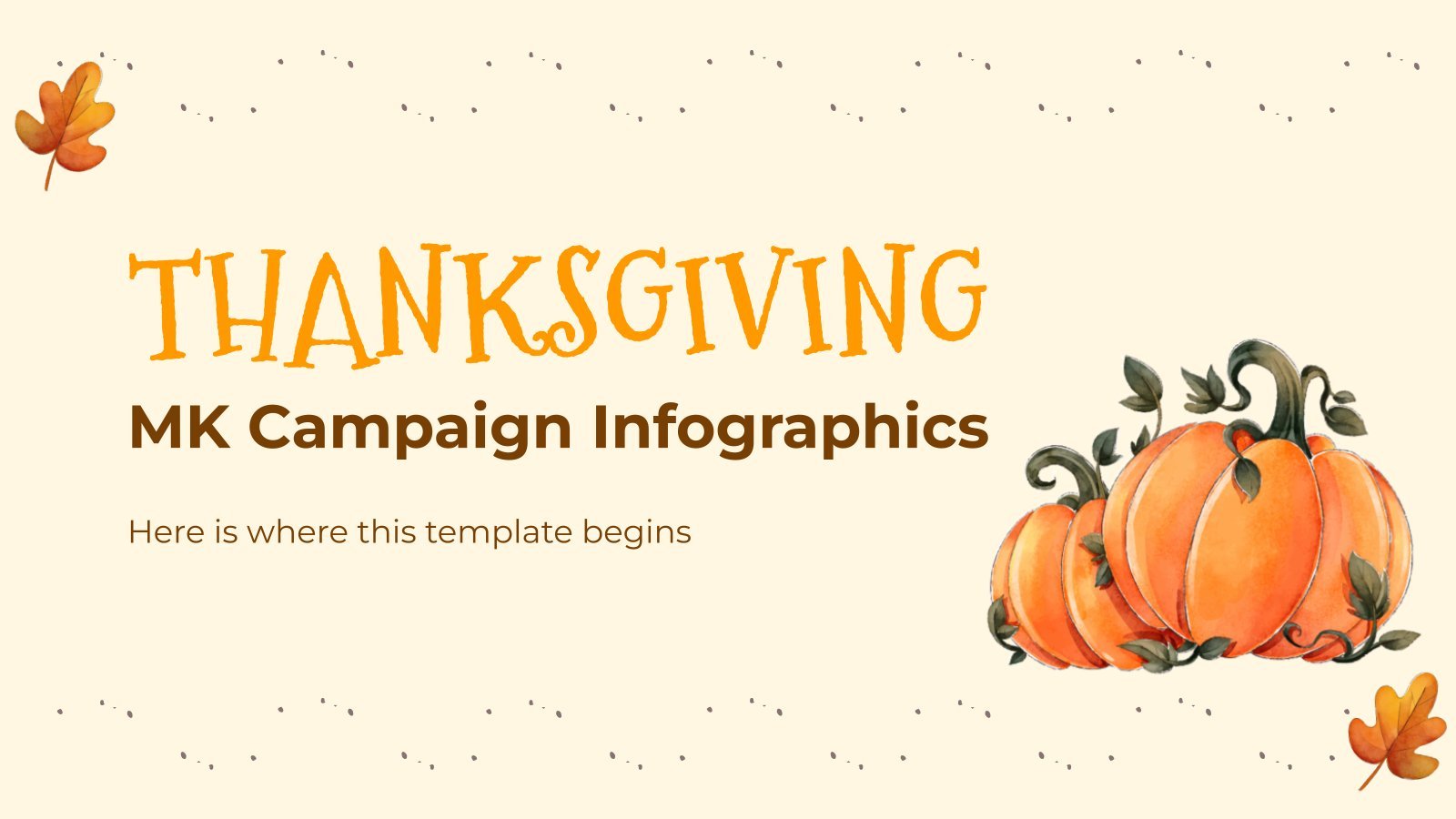
36 templates
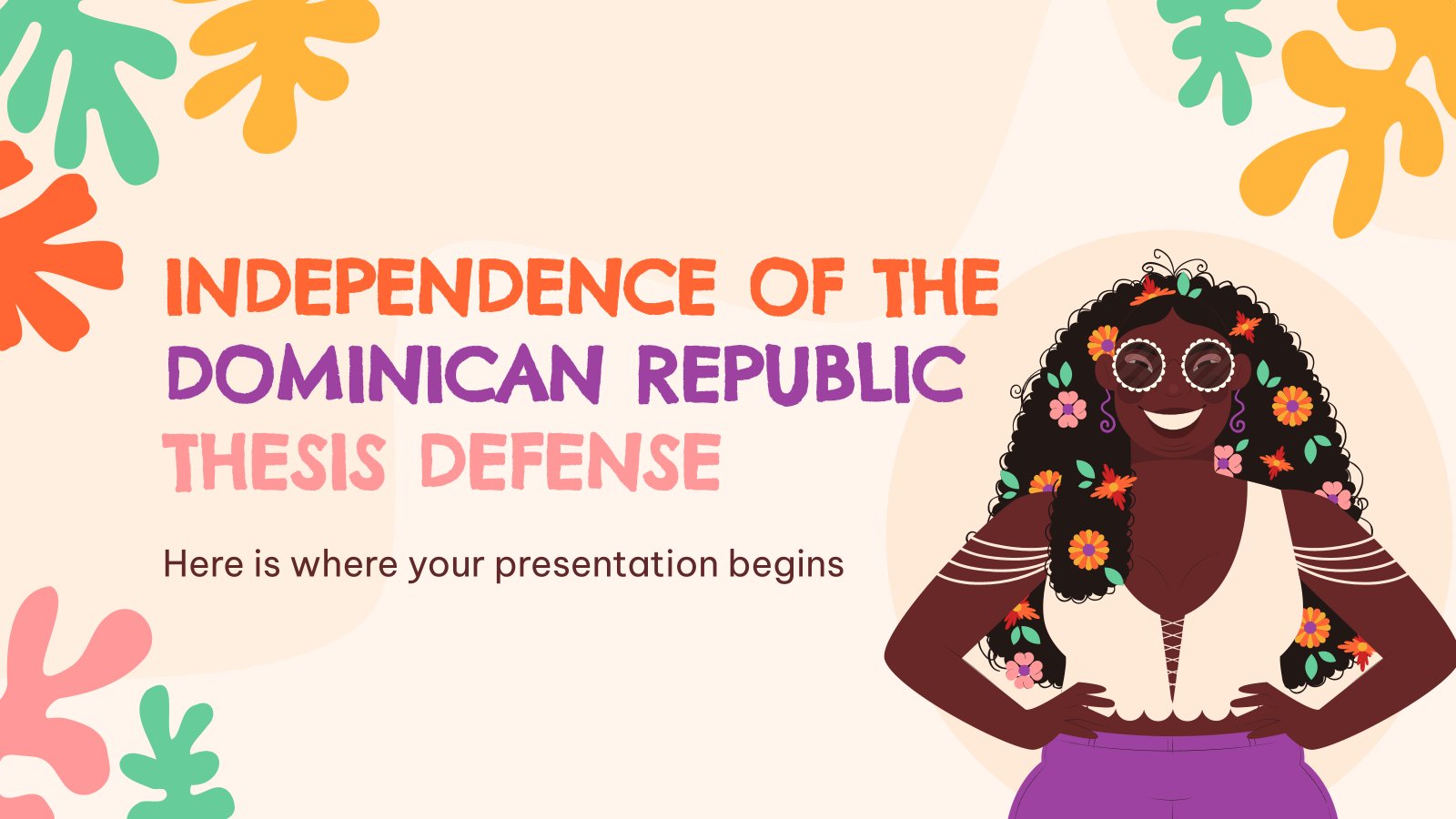
dominican republic
Teacher on special assignment (tosa) presentation templates, amid the vibrant world of education, our tosa-themed presentation templates celebrate the unique and impactful role of teachers on special assignment. these designs transform slides into engaging educational journeys, perfect for showcasing innovative strategies and inspiring the next generation of learners..
- Calendar & Weather
- Infographics
- Marketing Plan
- Project Proposal
- Social Media
- Thesis Defense
- Black & White
- Craft & Notebook
- Floral & Plants
- Illustration
- Interactive & Animated
- Professional
- Instagram Post
- Instagram Stories
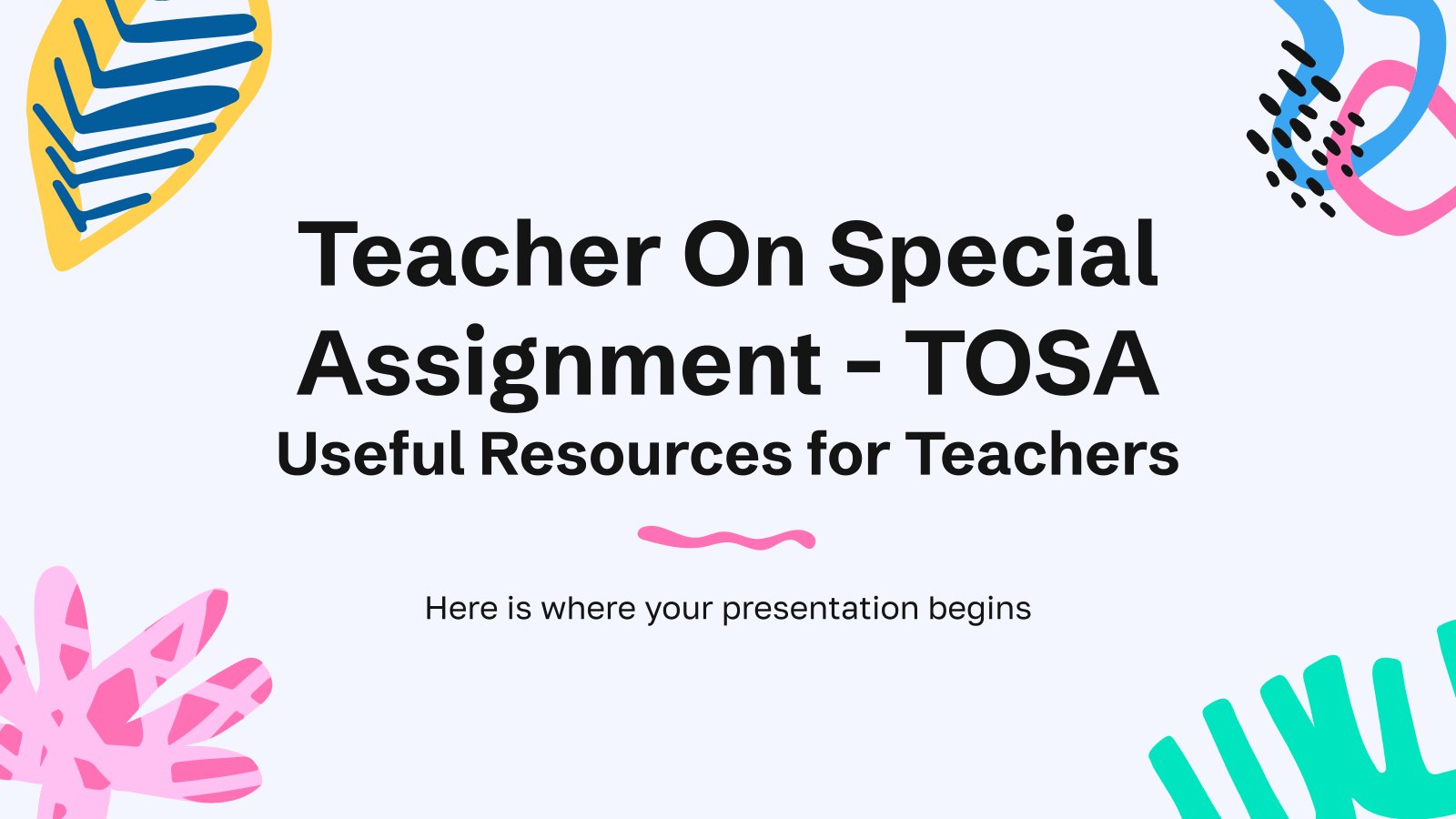
It seems that you like this template!
Premium template.
Unlock this template and gain unlimited access
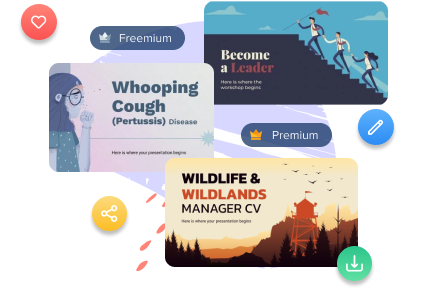
Register for free and start downloading now
Teacher on special assignment - tosa - useful resources for teachers.
Download the Teacher On Special Assignment - TOSA - Useful Resources for Teachers presentation for PowerPoint or Google Slides and start impressing your audience with a creative and original design. Slidesgo templates like this one here offer the possibility to convey a concept, idea or topic in a clear, concise...
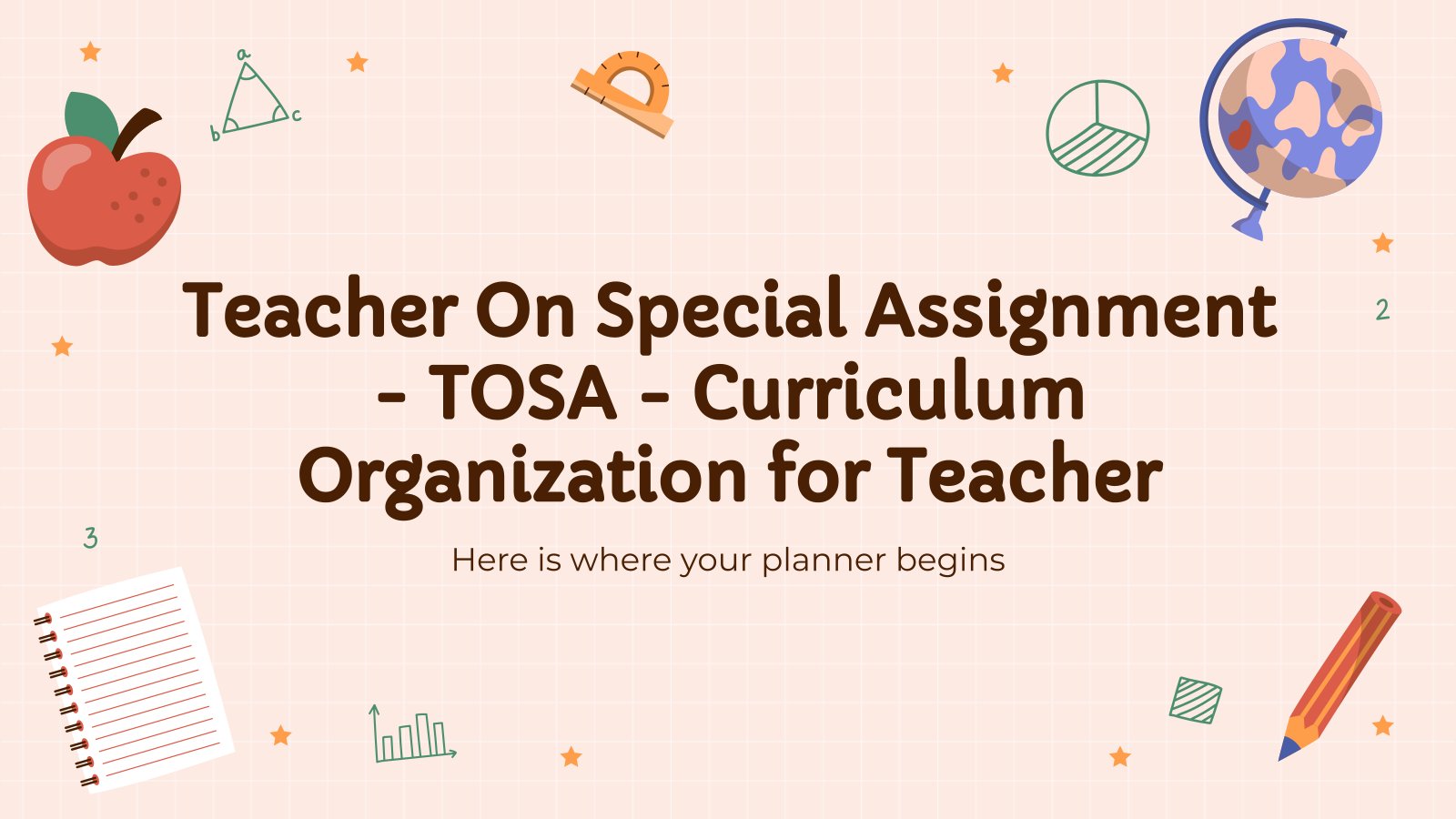
Teacher On Special Assignment - TOSA - Curriculum Organization for Teachers
Download the Teacher On Special Assignment - TOSA - Curriculum Organization for Teachers presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind. Offering the best resources, it allows educators or students...
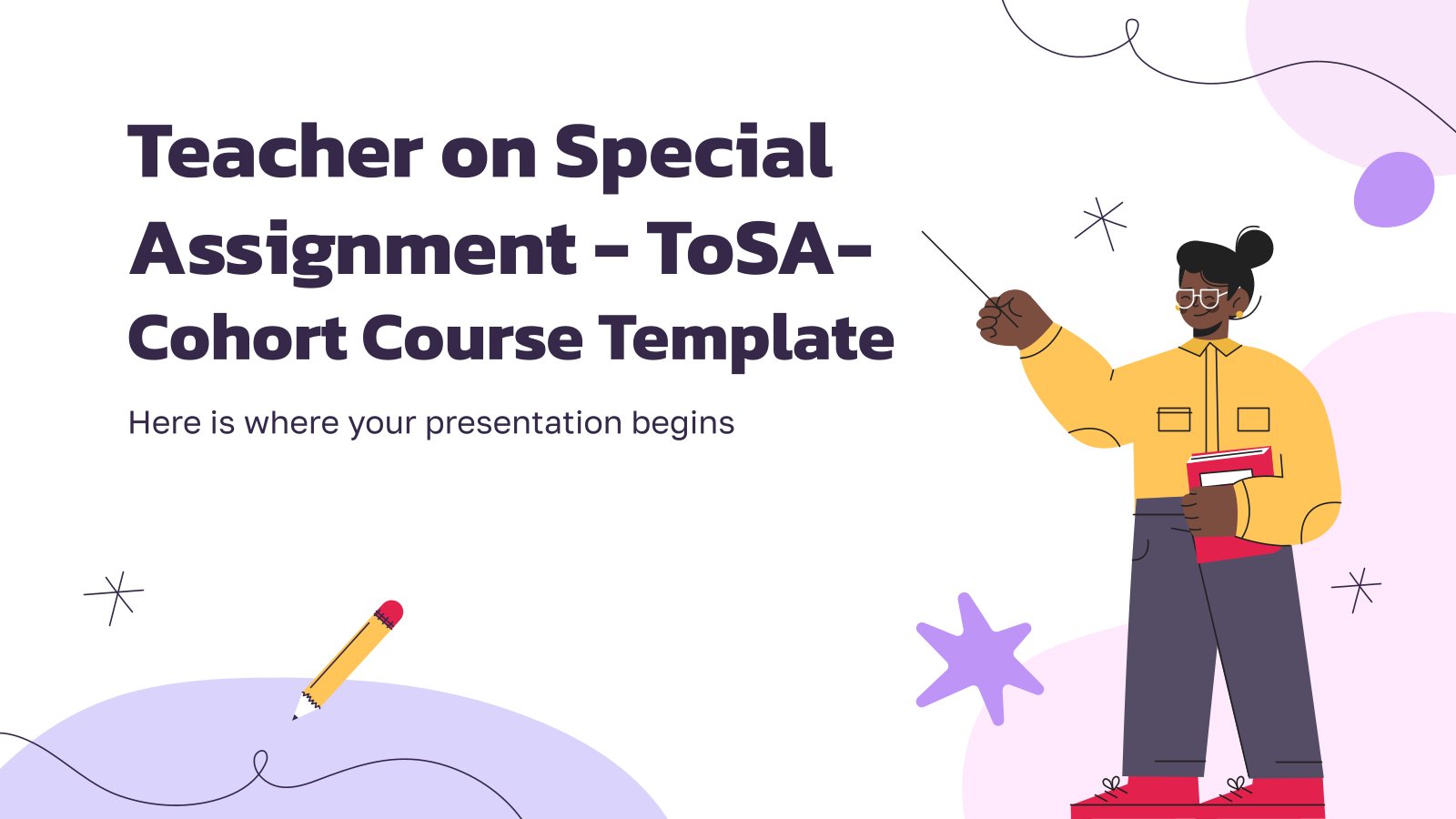
Teacher On Special Assignment - TOSA- Cohort Course Template
Download the Teacher On Special Assignment - TOSA- Cohort Course Template presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind. Offering the best resources, it allows educators or students to efficiently...
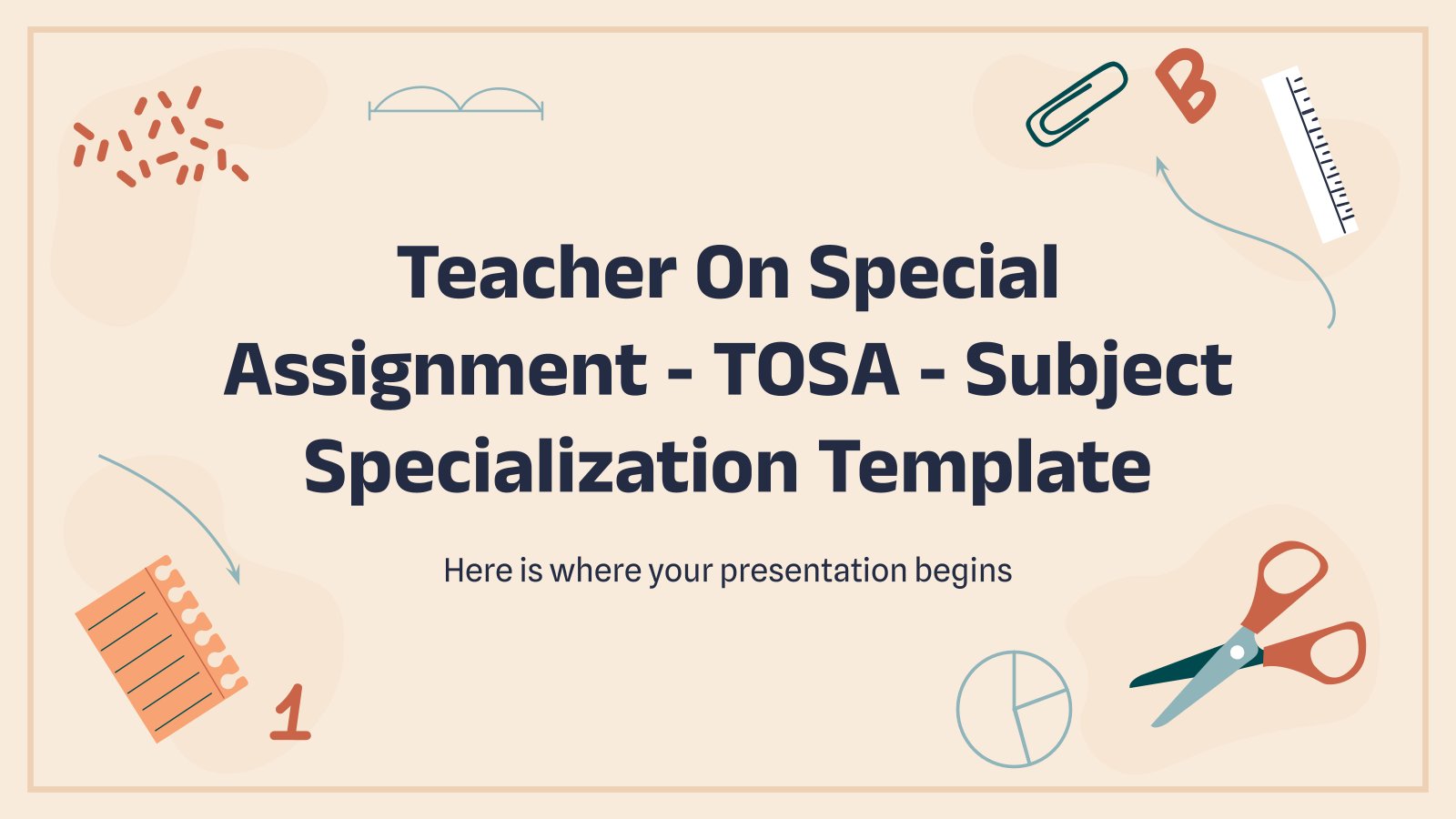
Teacher On Special Assignment - TOSA - Subject Specialization Template
Download the Teacher On Special Assignment - TOSA - Subject Specialization Template presentation for PowerPoint or Google Slides and start impressing your audience with a creative and original design. Slidesgo templates like this one here offer the possibility to convey a concept, idea or topic in a clear, concise and...
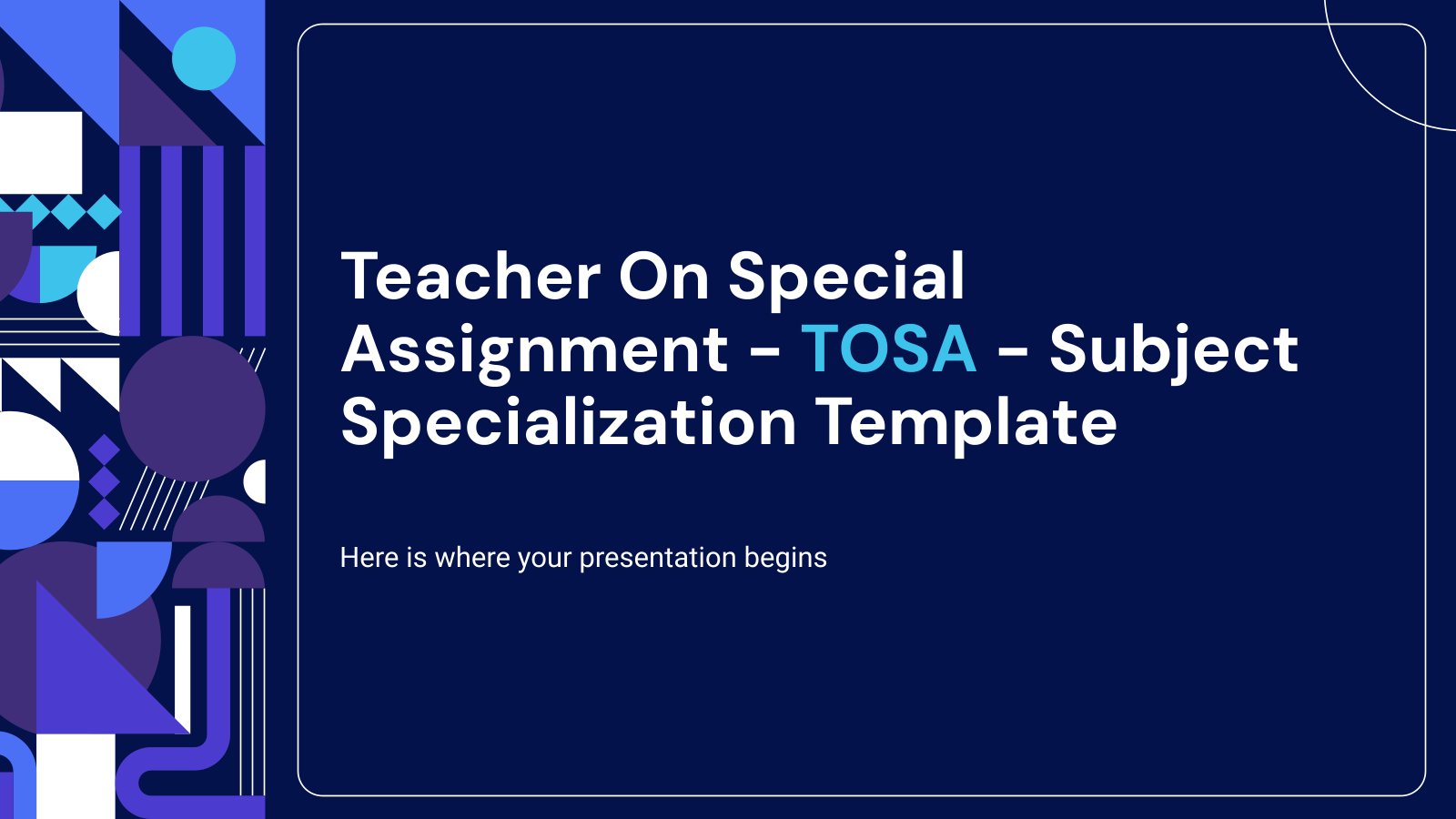
Download the Teacher On Special Assignment - TOSA - Subject Specialization Template presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind. Offering the best resources, it allows educators or students to...
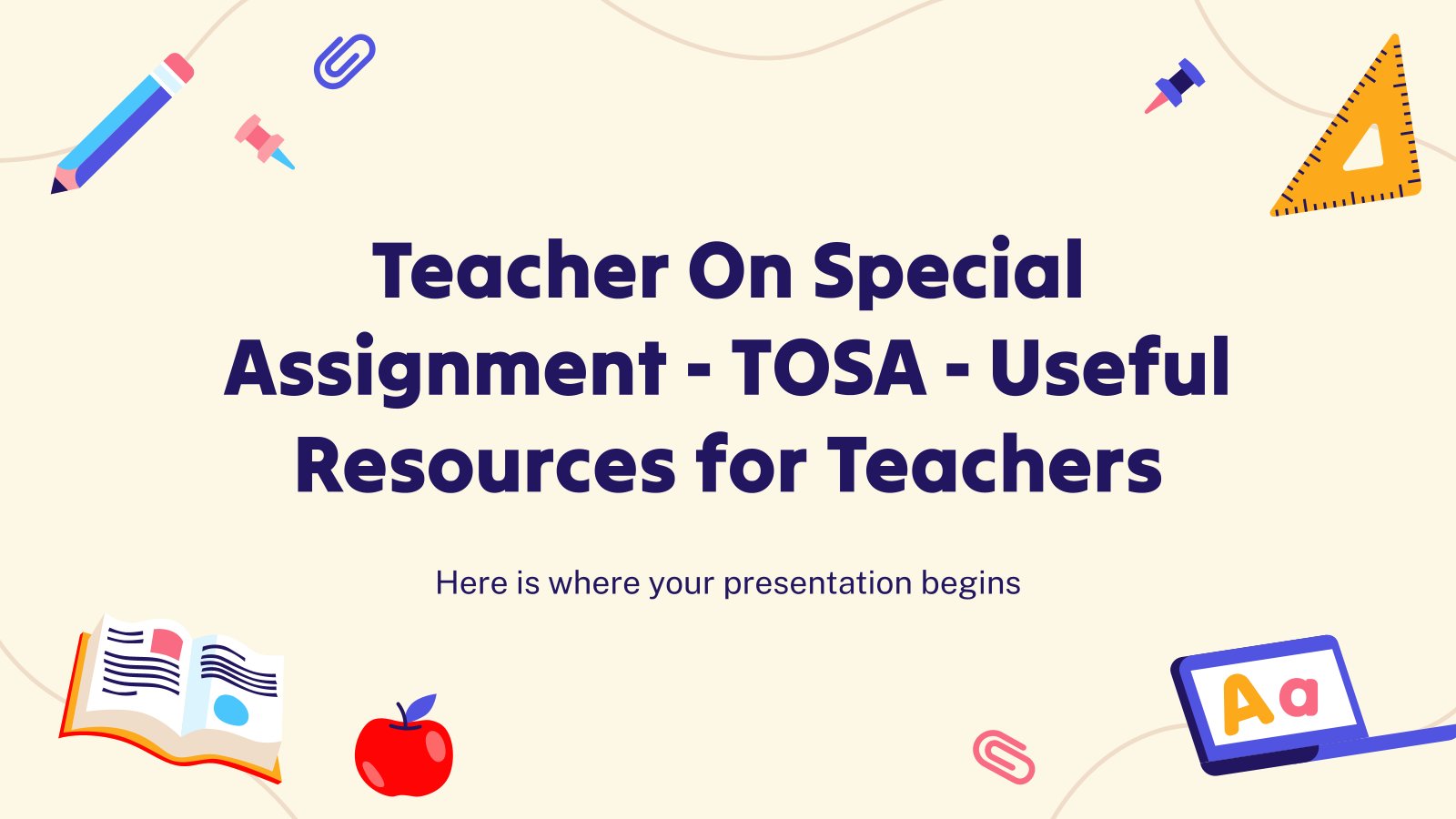
Create your presentation Create personalized presentation content
Writing tone, number of slides.
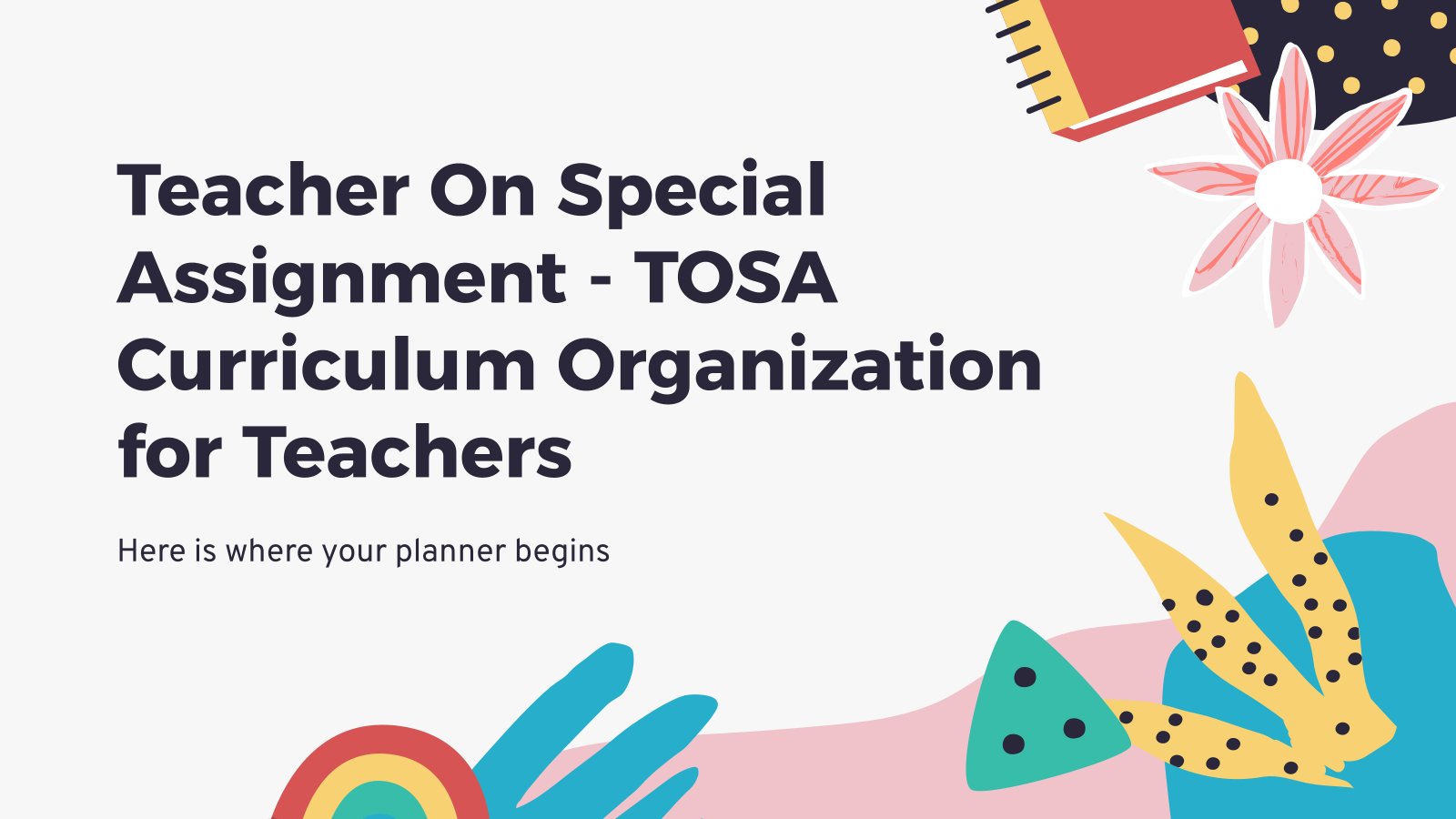
Download the Teacher On Special Assignment - TOSA - Curriculum Organization for Teachers presentation for PowerPoint or Google Slides and start impressing your audience with a creative and original design. Slidesgo templates like this one here offer the possibility to convey a concept, idea or topic in a clear, concise...
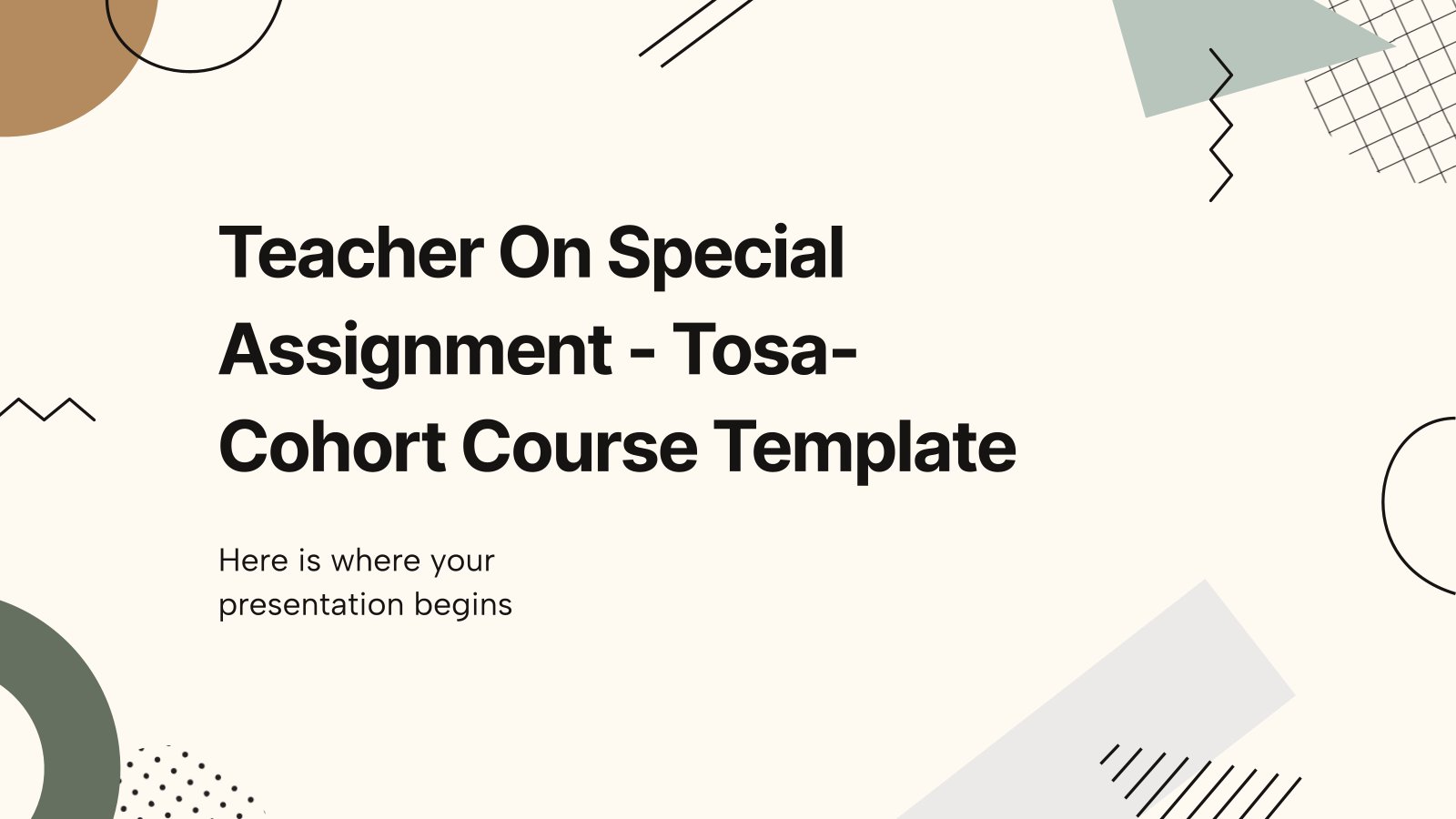
Teacher On Special Assignment - TOSA - Co-teaching Template
Download the Teacher On Special Assignment - TOSA - Co-teaching Template presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind. Offering the best resources, it allows educators or students to efficiently...
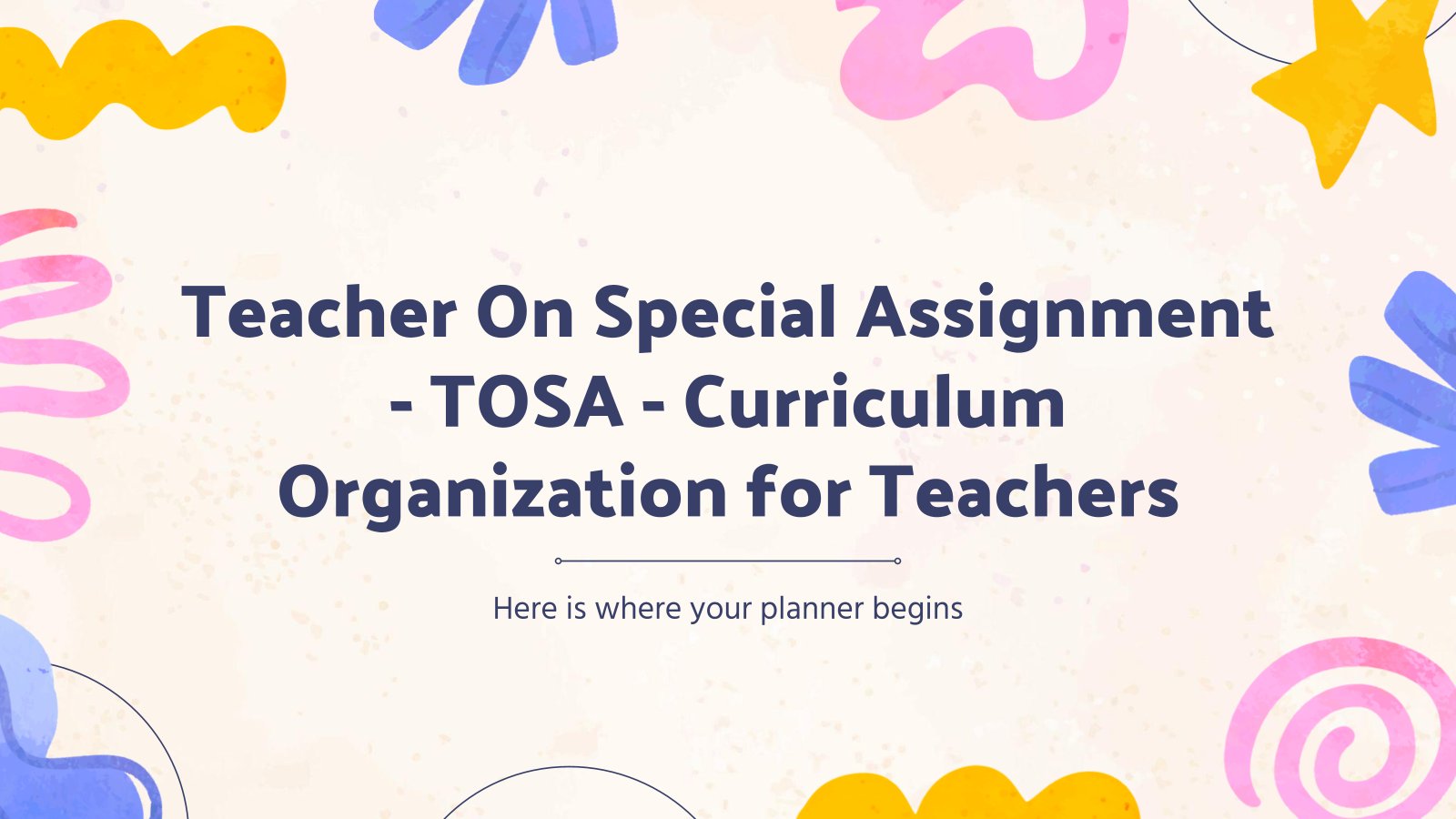
Teacher On Special Assignment - TOSA - Resume
Download the "Teacher On Special Assignment - TOSA - Resume" presentation for PowerPoint or Google Slides. Having a good CV can make all the difference in landing your dream job. It's not just a piece of paper, it's your chance to showcase your skills, experience, and personality. If you want...
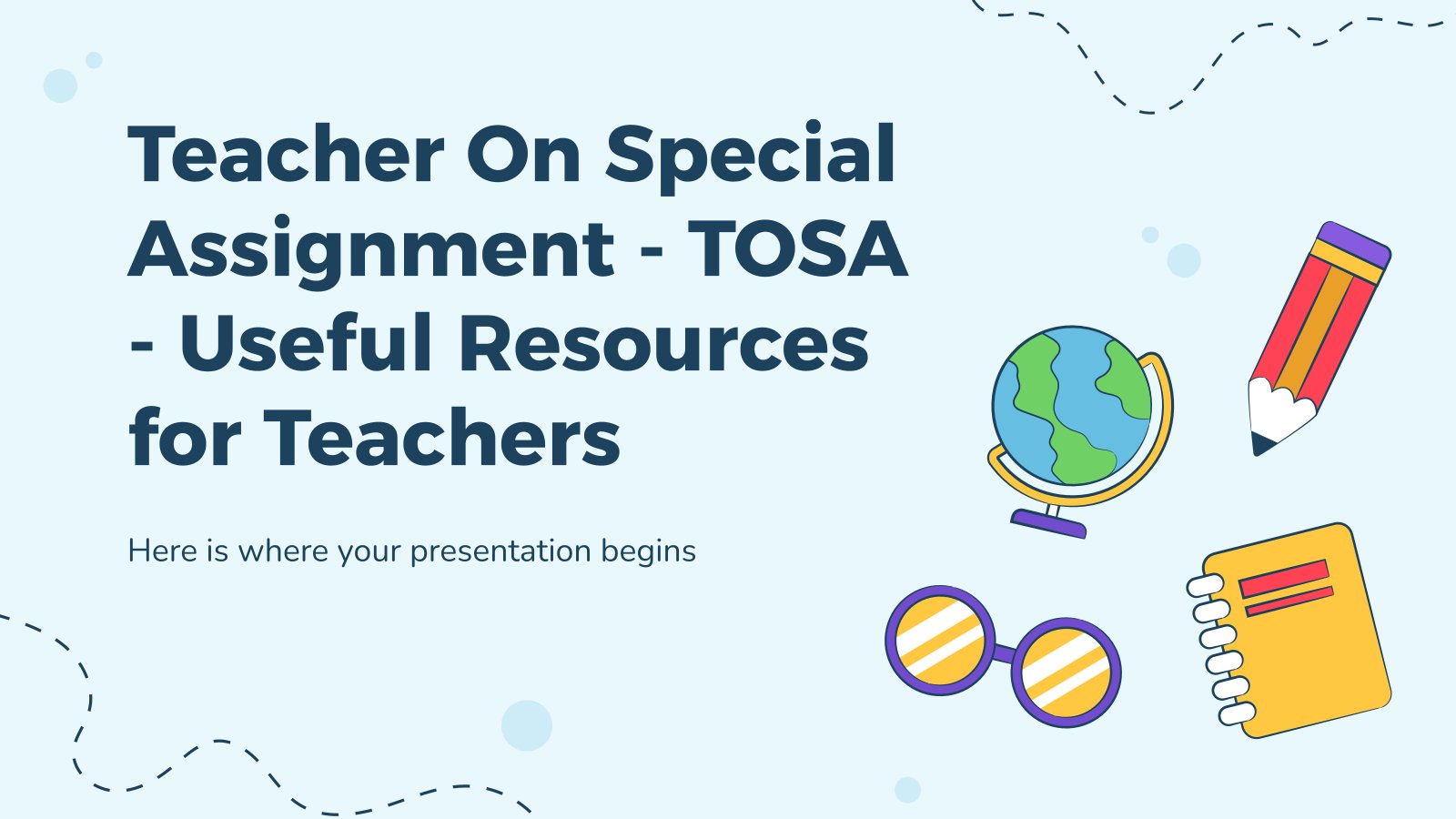
Download the Teacher On Special Assignment - TOSA - Useful Resources for Teachers presentation for PowerPoint or Google Slides. Whether you're an entrepreneur looking for funding or a sales professional trying to close a deal, a great pitch deck can be the difference-maker that sets you apart from the competition....
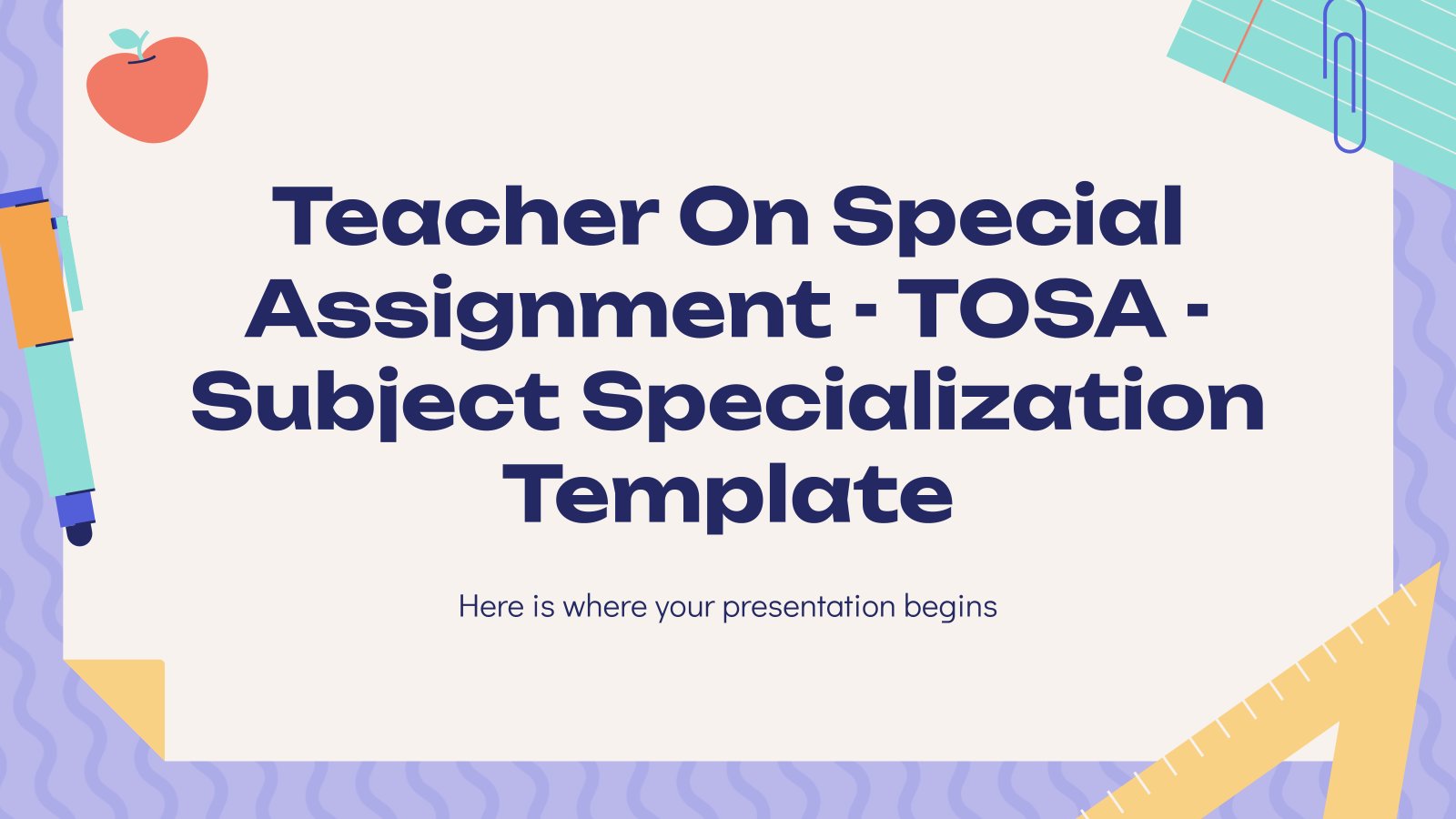
Teacher On Special Assignment Onboarding
Download the Teacher On Special Assignment Onboarding presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind. Offering the best resources, it allows educators or students to efficiently manage their presentations and...
- Page 1 of 3
Register for free and start editing online
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Sample Go Project Template (based on the layout from the Standard Project Layout repo)
golang-standards/project-template
Folders and files.
Name | Name | |||
---|---|---|---|---|
1 Commit | ||||
Repository files navigation
Go project template.
This is an opinionated Go project template you can use as a starting point for your project. It doesn't include any code generation, so you'll need to replace the placeholder variables/values/names with your own.
Clone the repository, keep what you need and delete everything else! Feel free to replace the parts that don't align with your use cases (e.g., you may prefer/use a different vendoring tool).
See Go Project Layout for a more generic and a less opinionated starting point for your project.
- -3 more templates
- No results for
Assignment Tracker templates
Streamline your academic workflow with our versatile assignment tracker templates, ideal for both individual tasks and collaborative group assignments. effortlessly monitor due dates, progress milestones, and peer contributions, ensuring nothing falls through the cracks. notion brings clarity and cohesion to every academic pursuit..
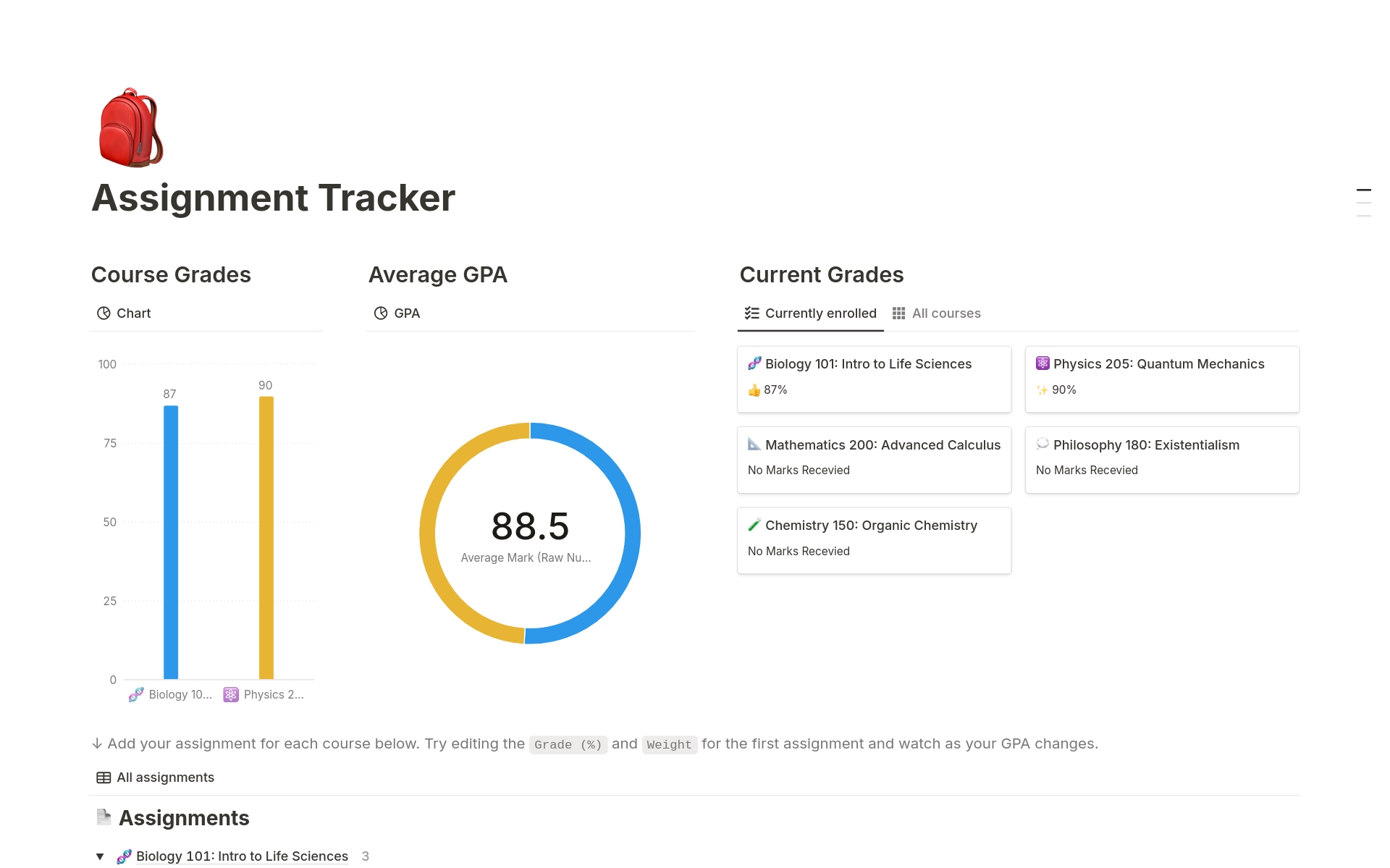
Assignment Tracker with Automations
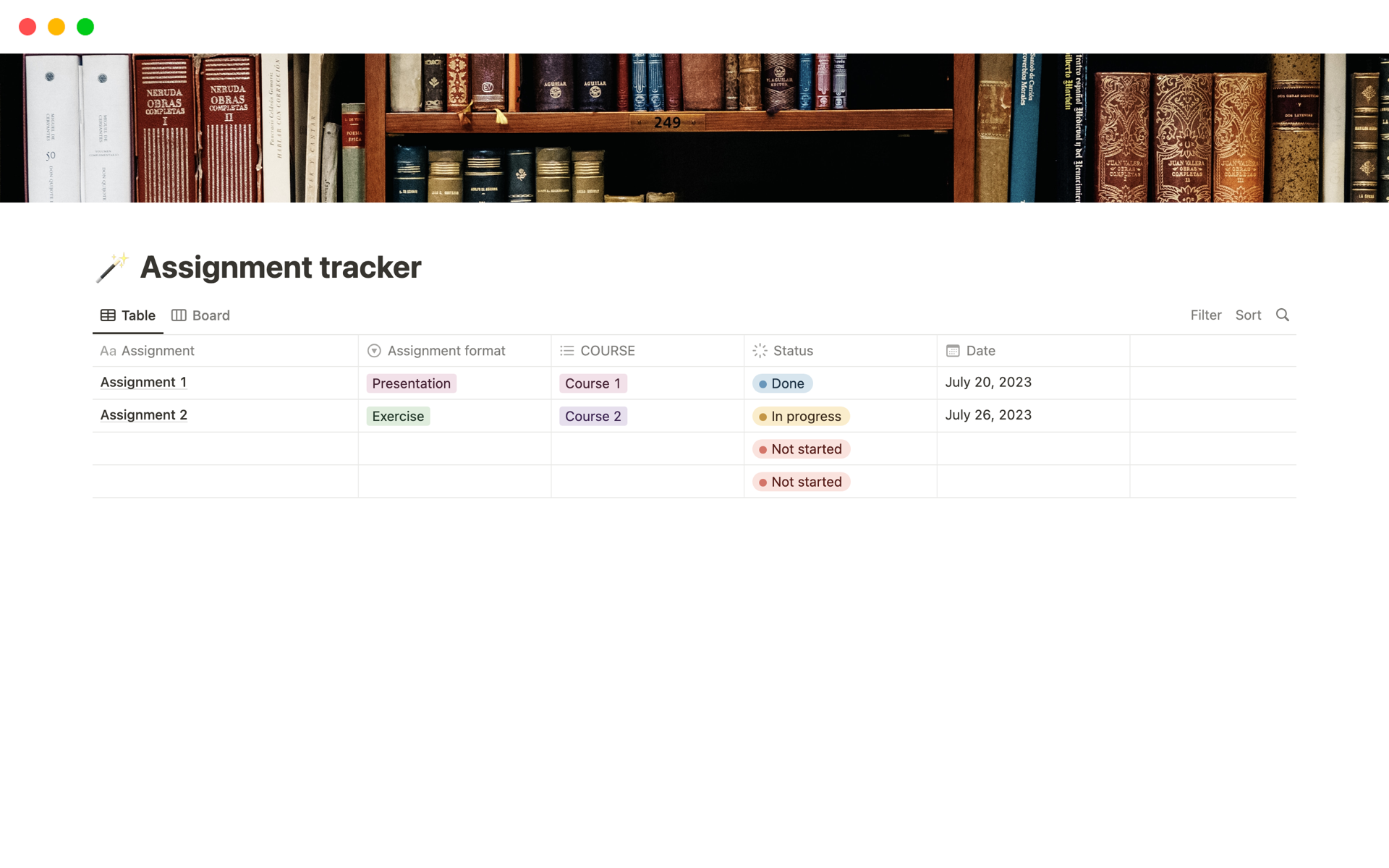
Assignment Tracker
Dzifianu Afi
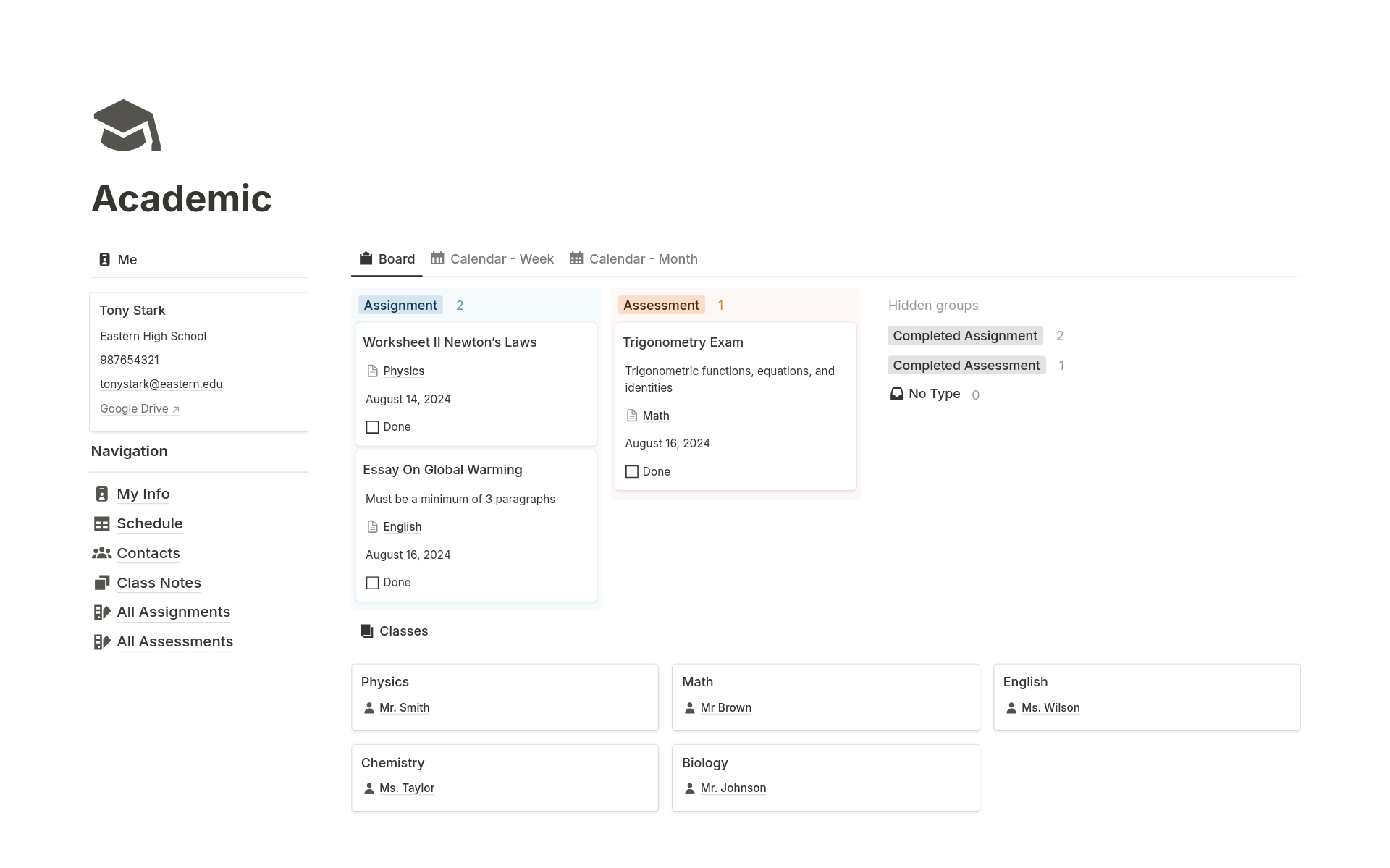
Academic Dashboard for Students
turbojellyfish
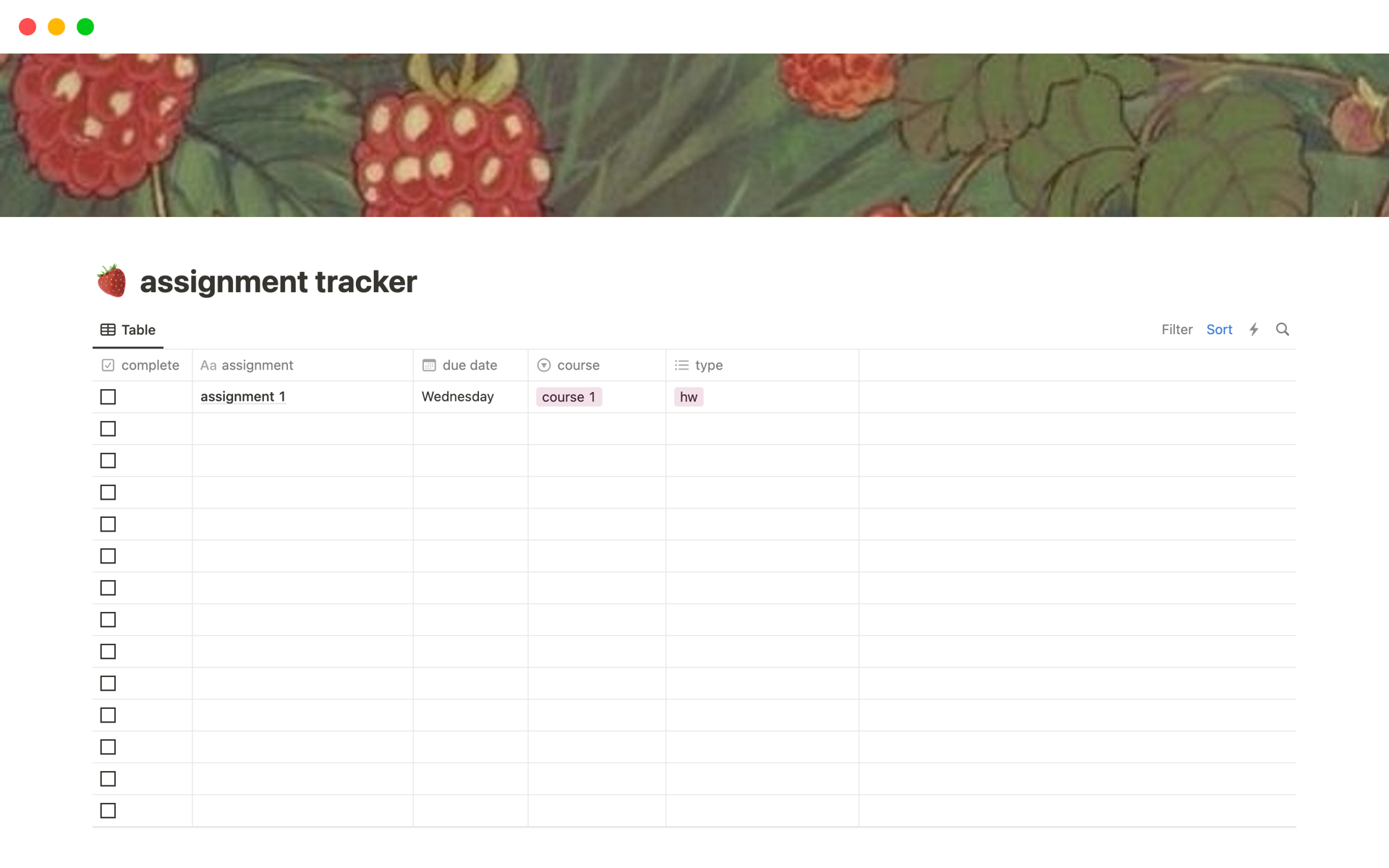
berry sweet assignment tracker
chaelin <3
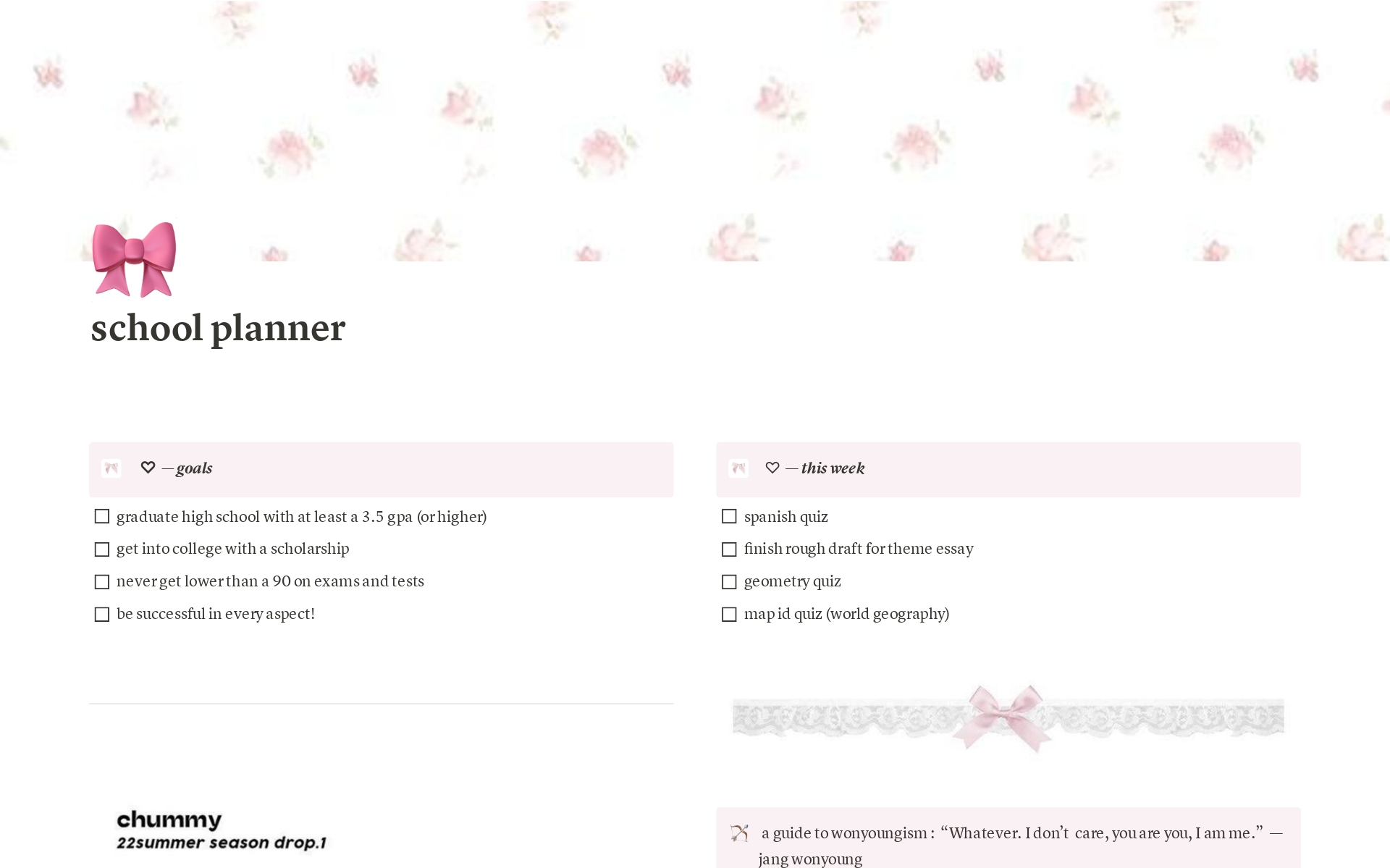
pink coquette school planner
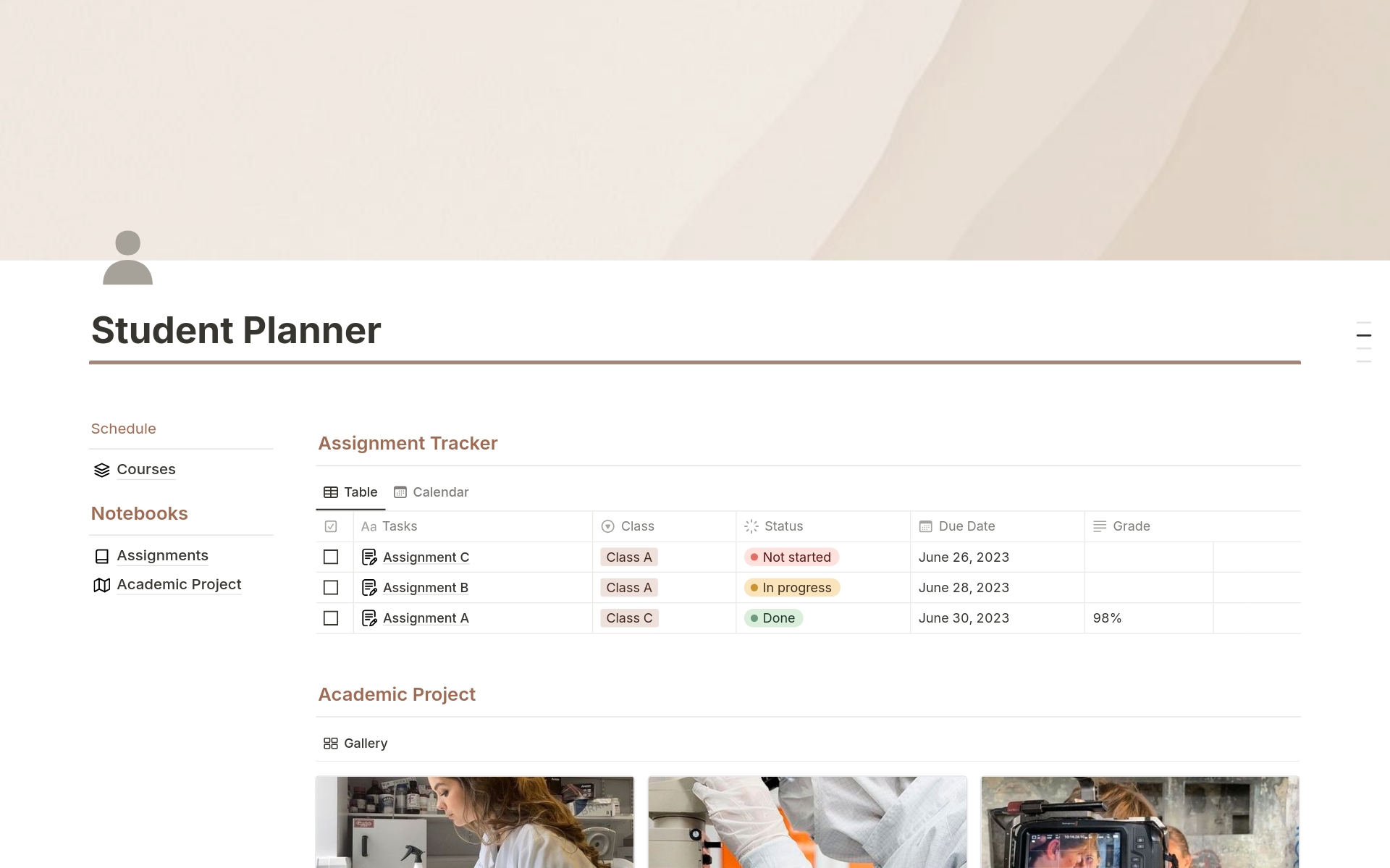
Student Planner and Assignment Tracker
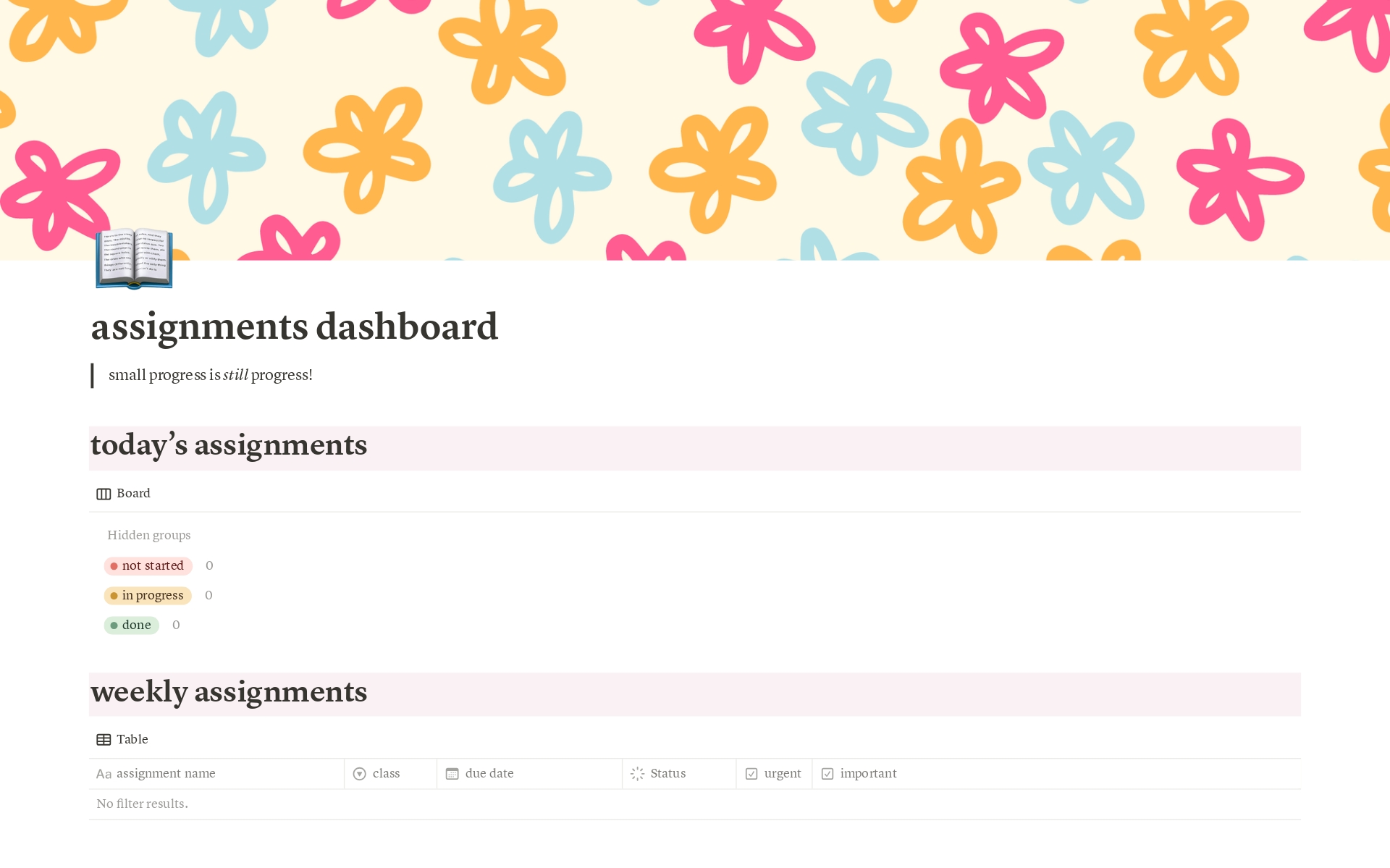
Caroline @ QUIRKLY
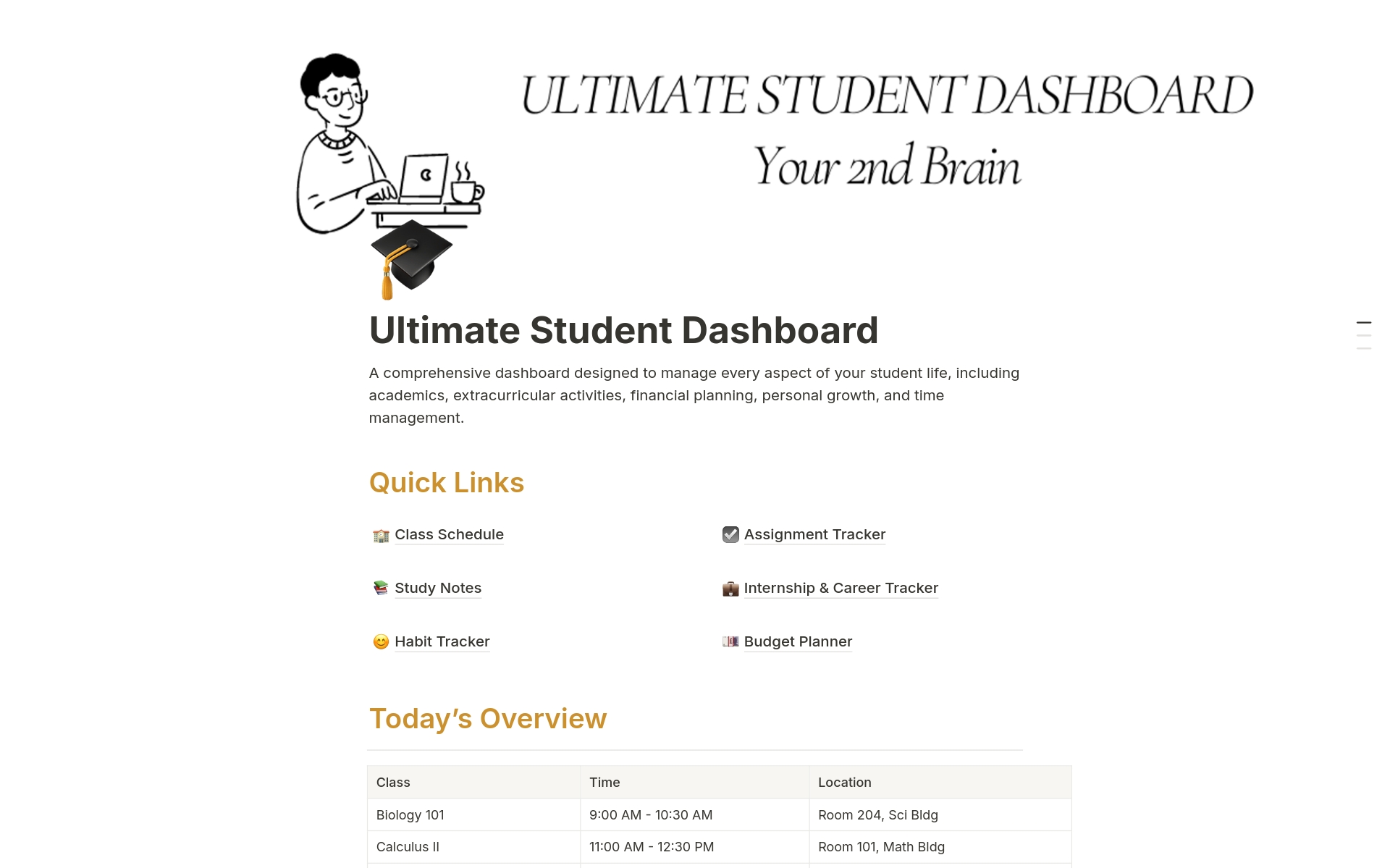
Ultimate Student Dashboard
Rohullah Hamid
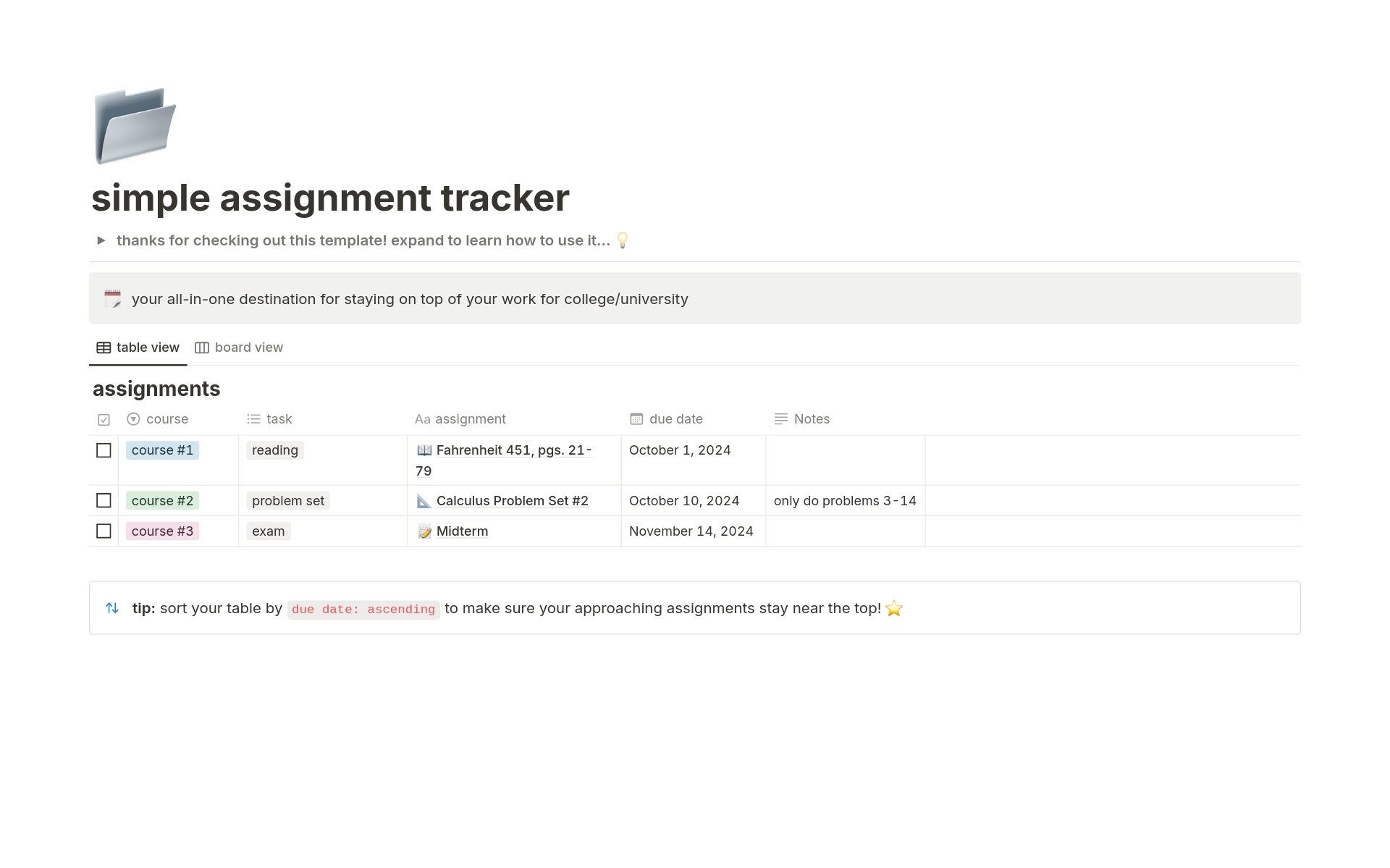
simple assignment tracker
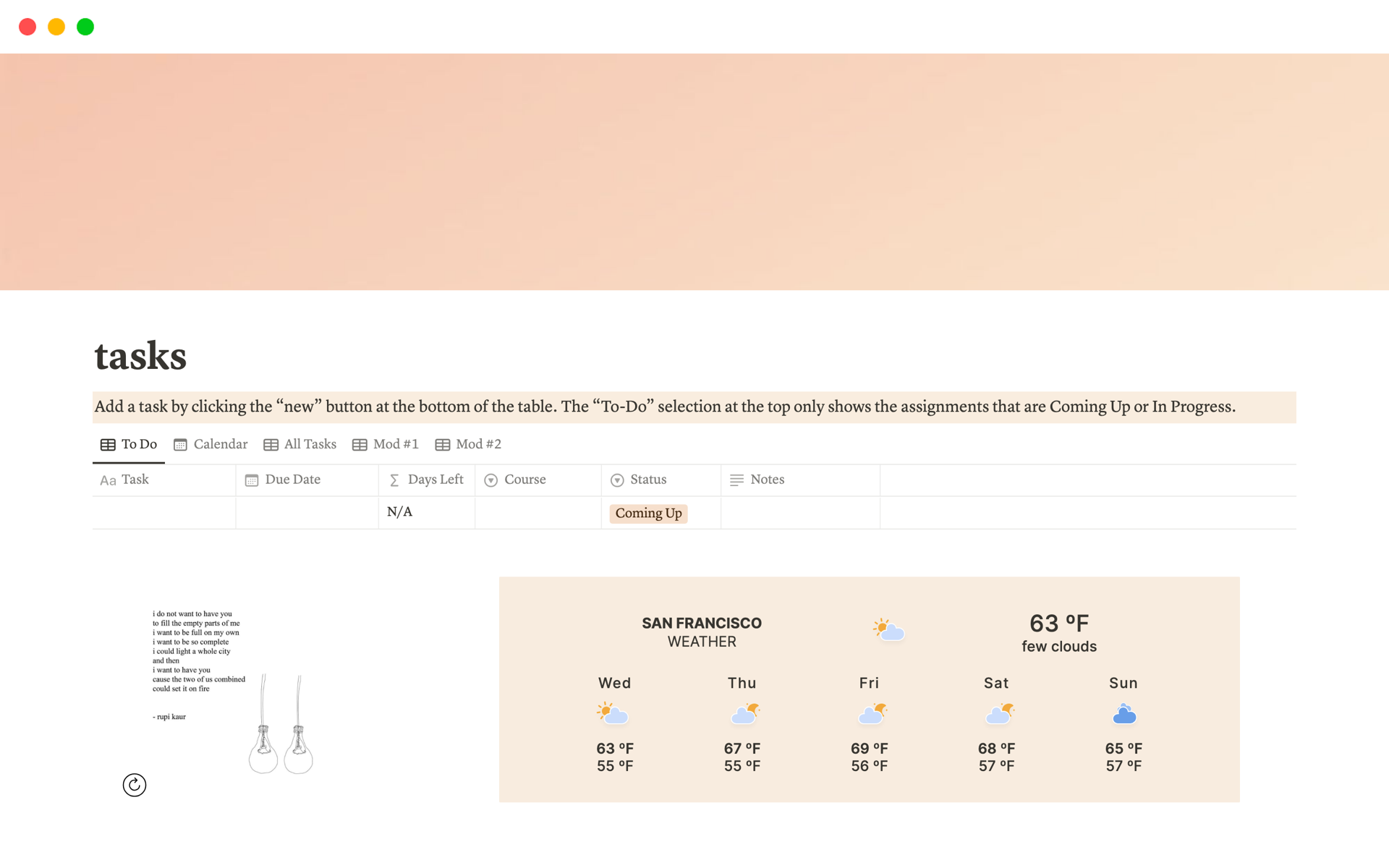
Cute and Simple Student Assignment Tracker
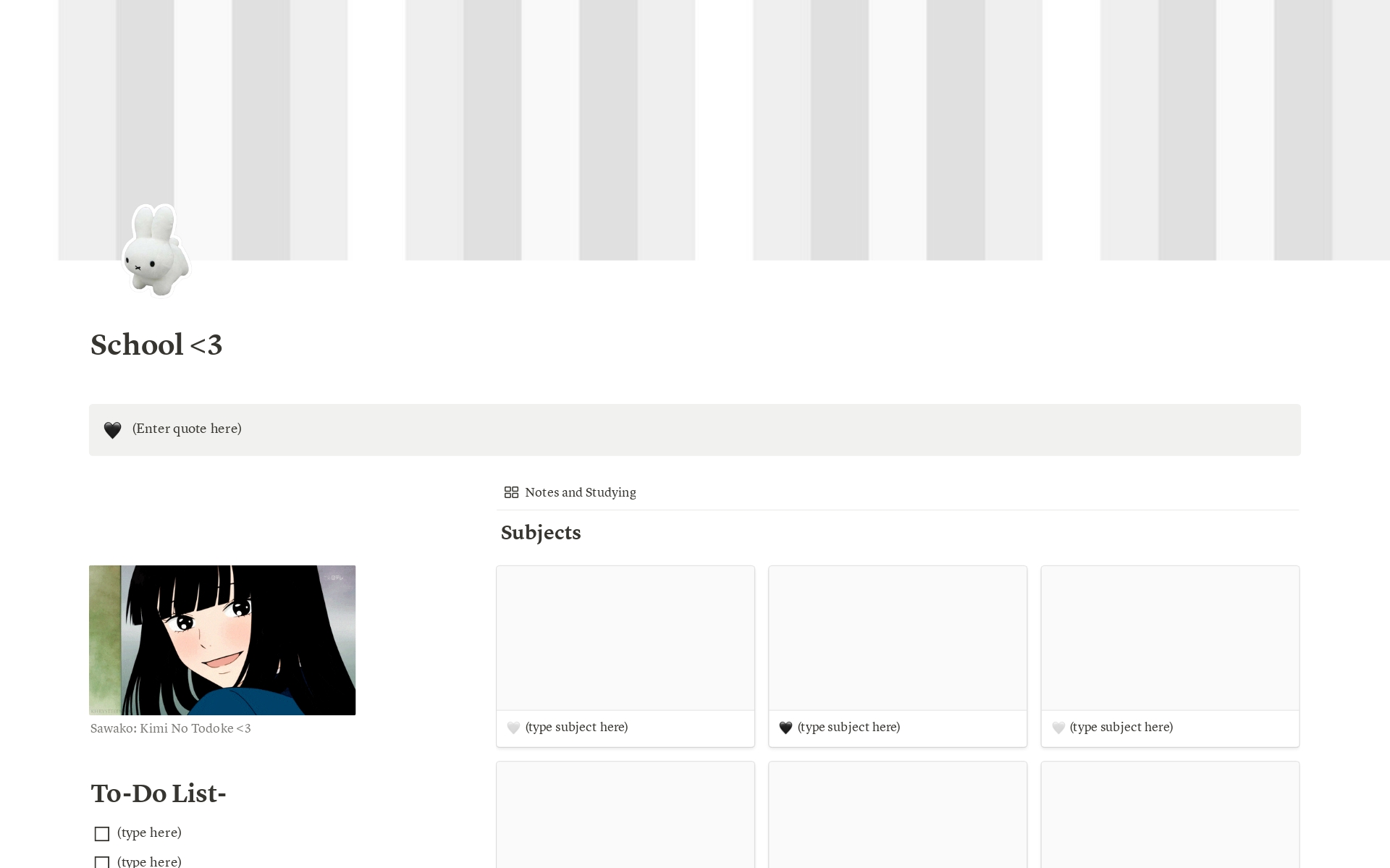
Aesthetic School Assignment Tracker/Notes <3
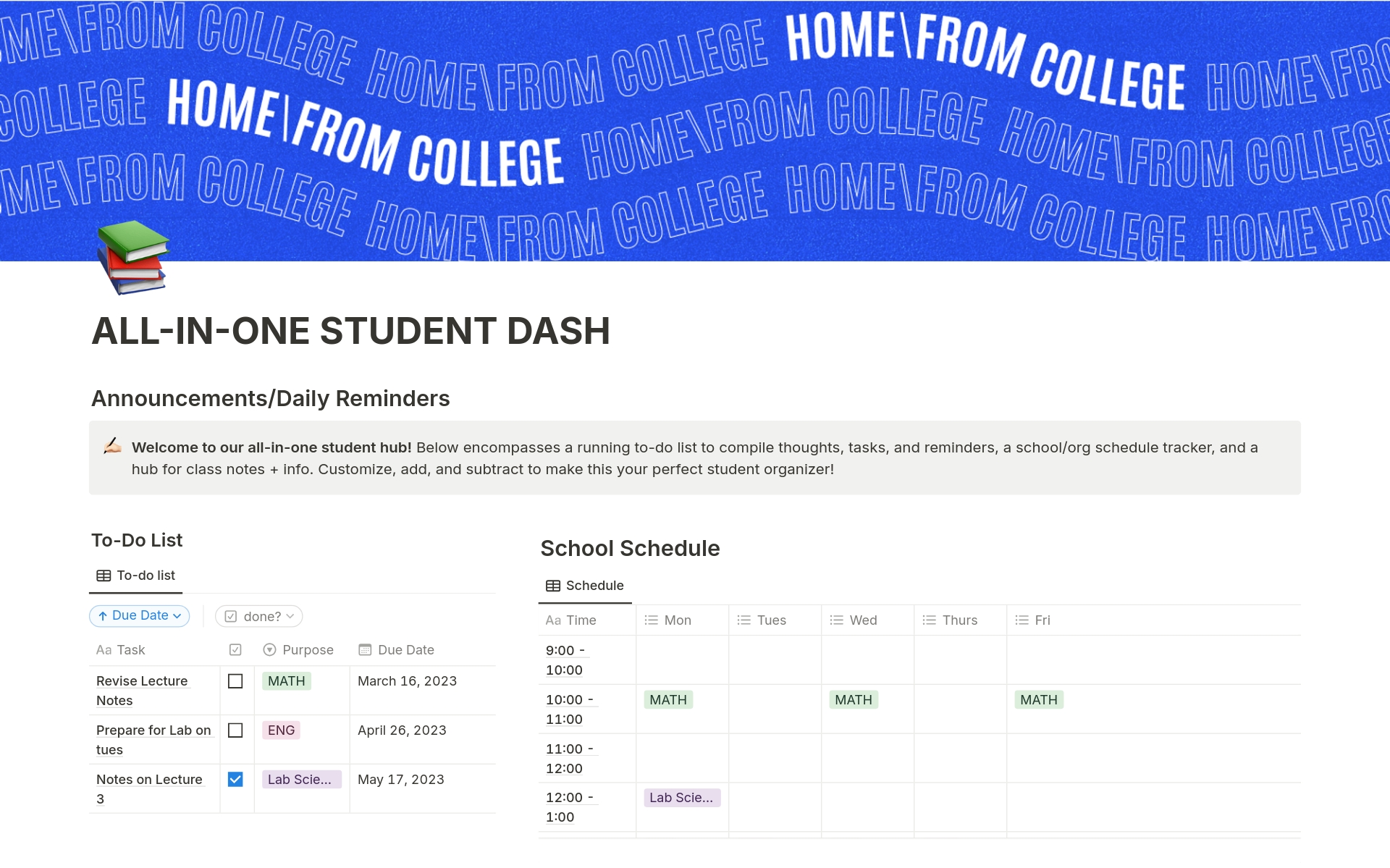
All-In-One Student Dashboard
Home From College
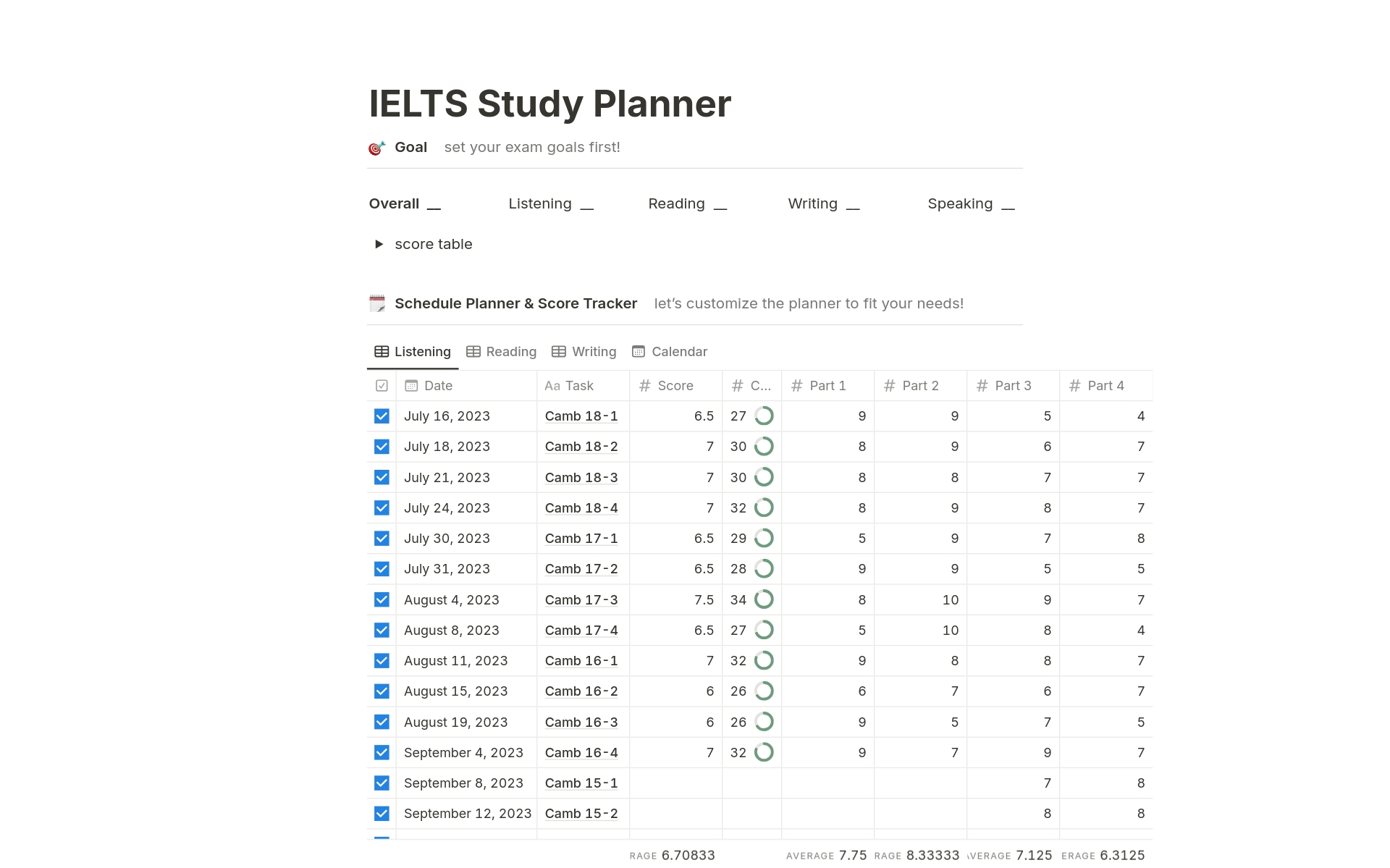
IELTS Study Planner
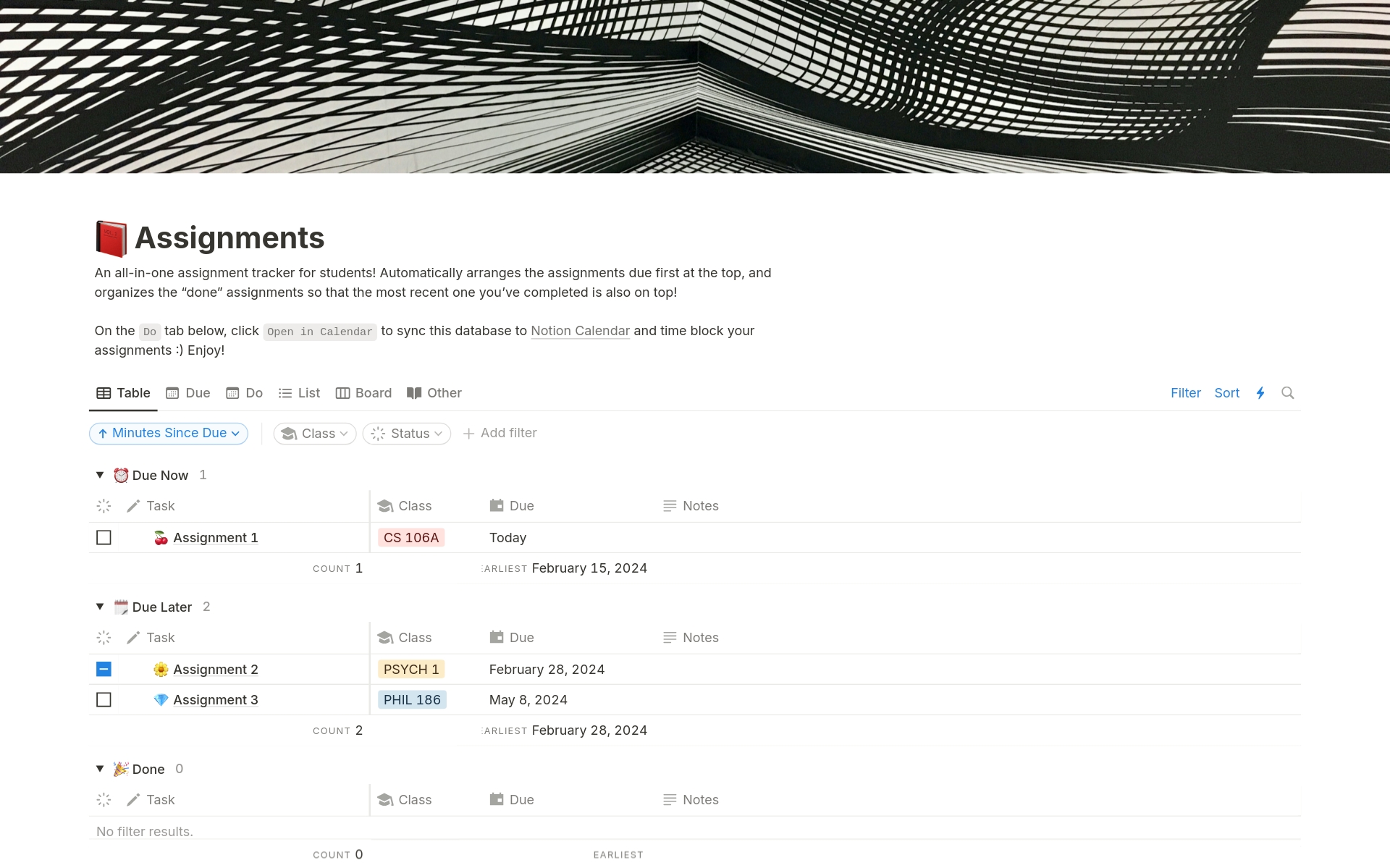
Simple Assignment Tracker
Sam Catania
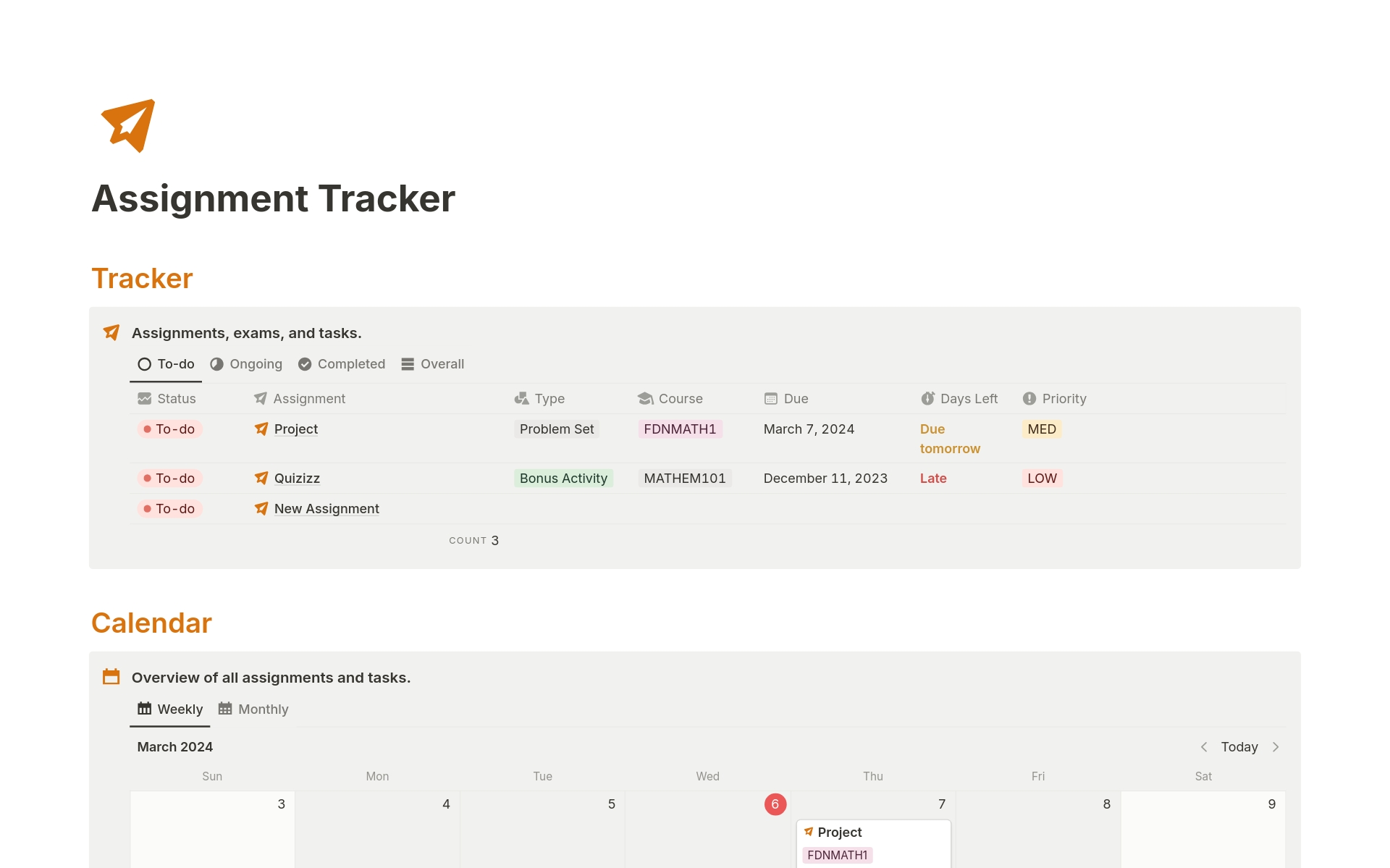
Basic school dashboard
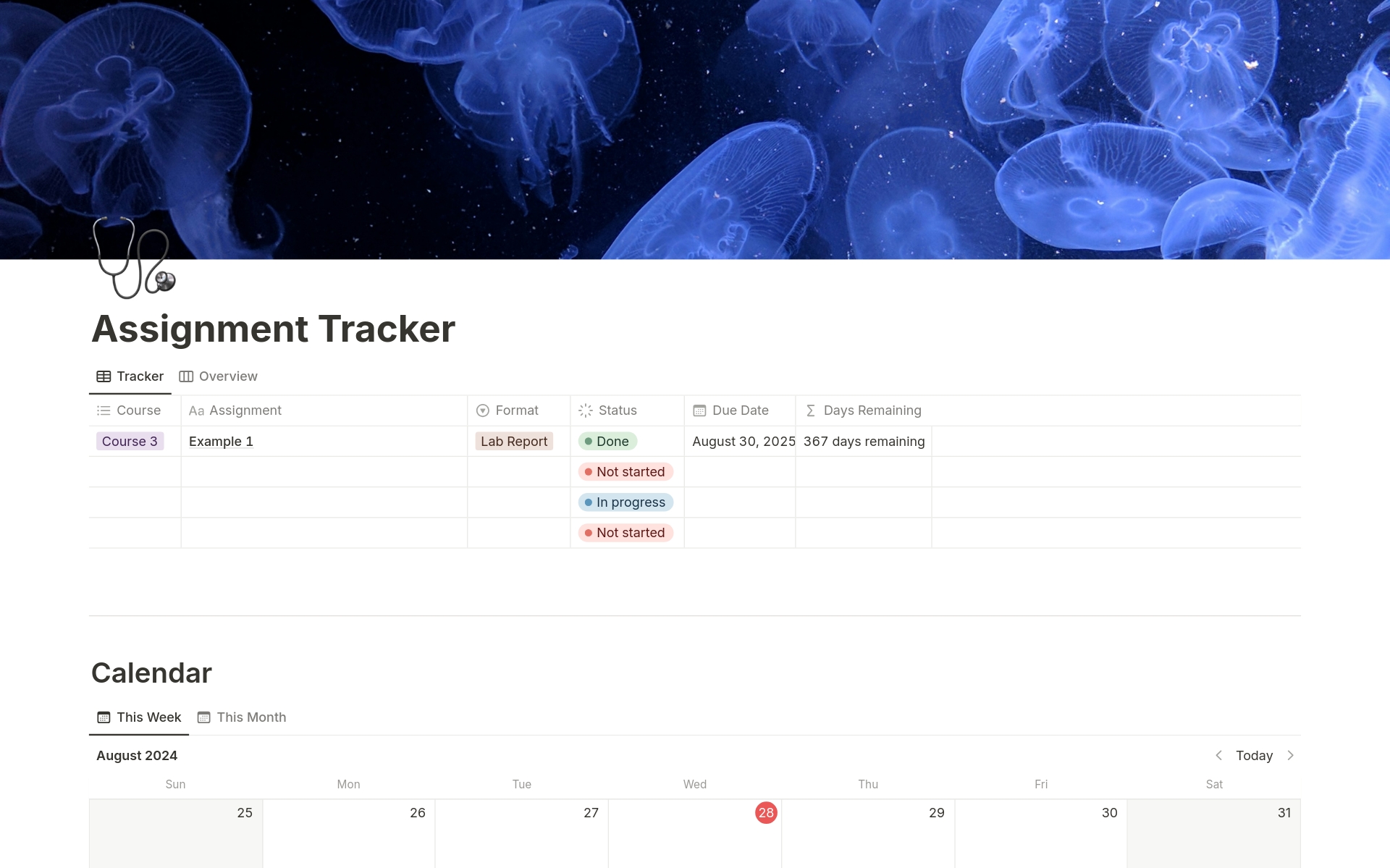
Minimalist Study Zone
Productivity horizon
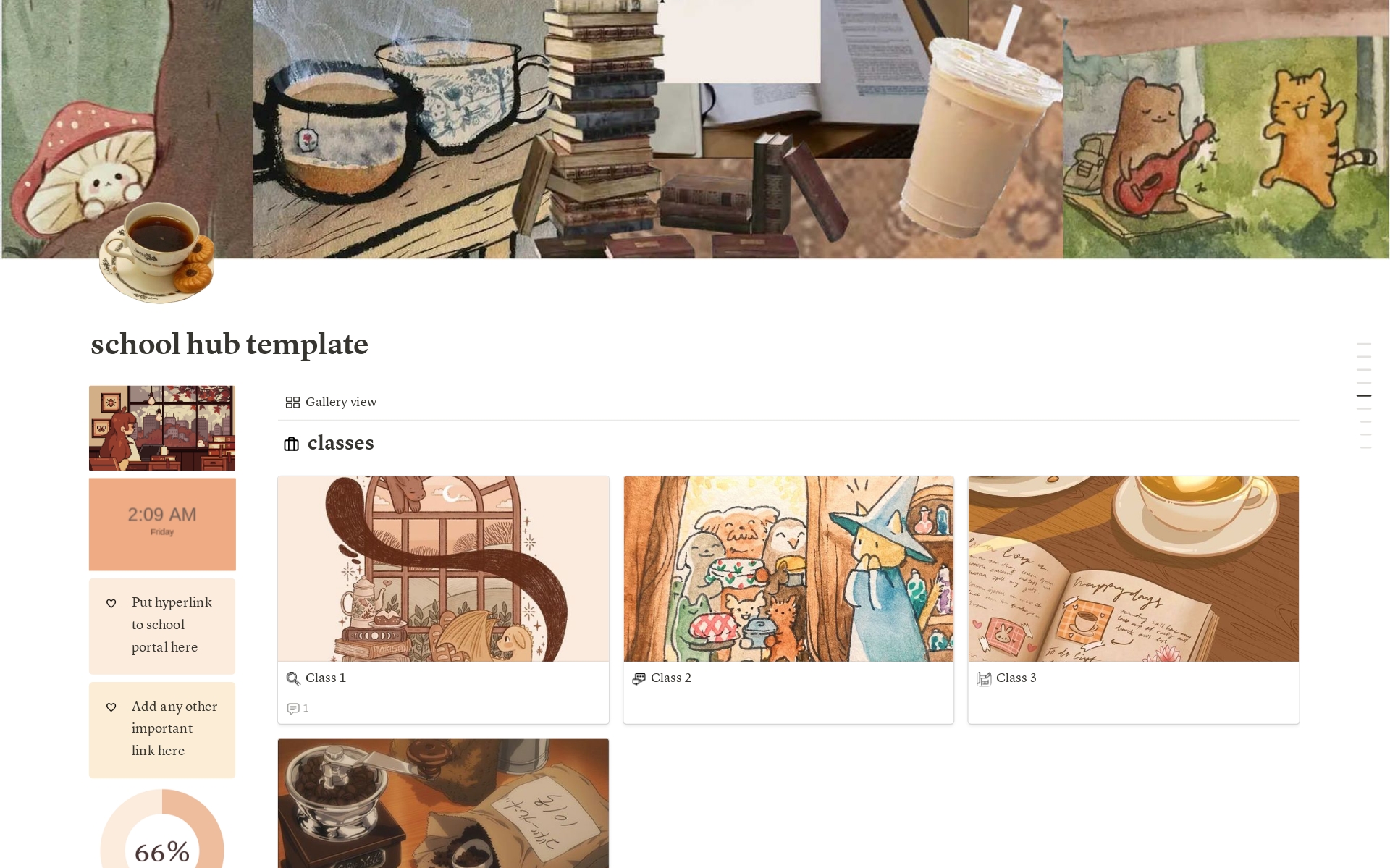
Wonyoung School Template
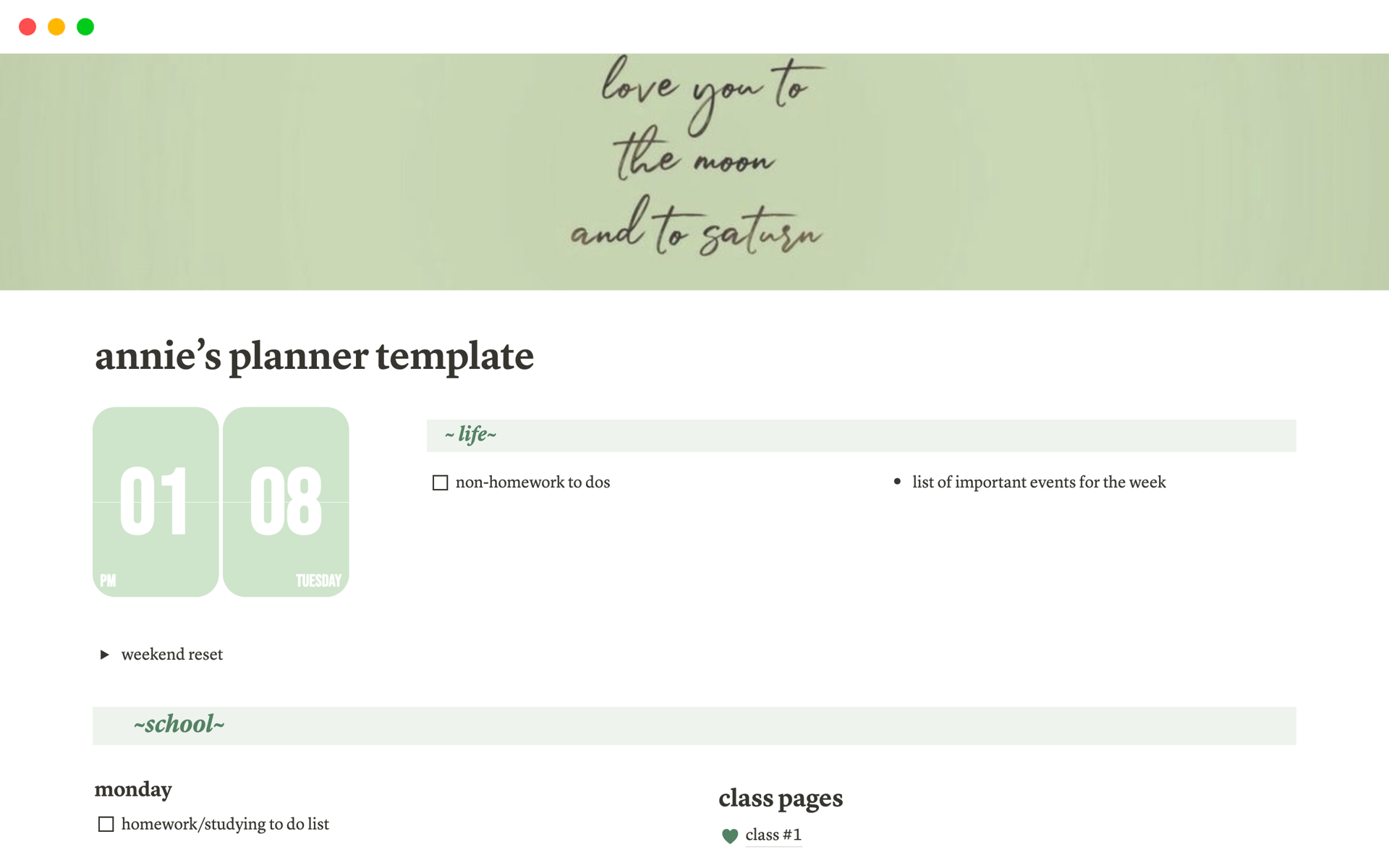
academic planner
annie's planners
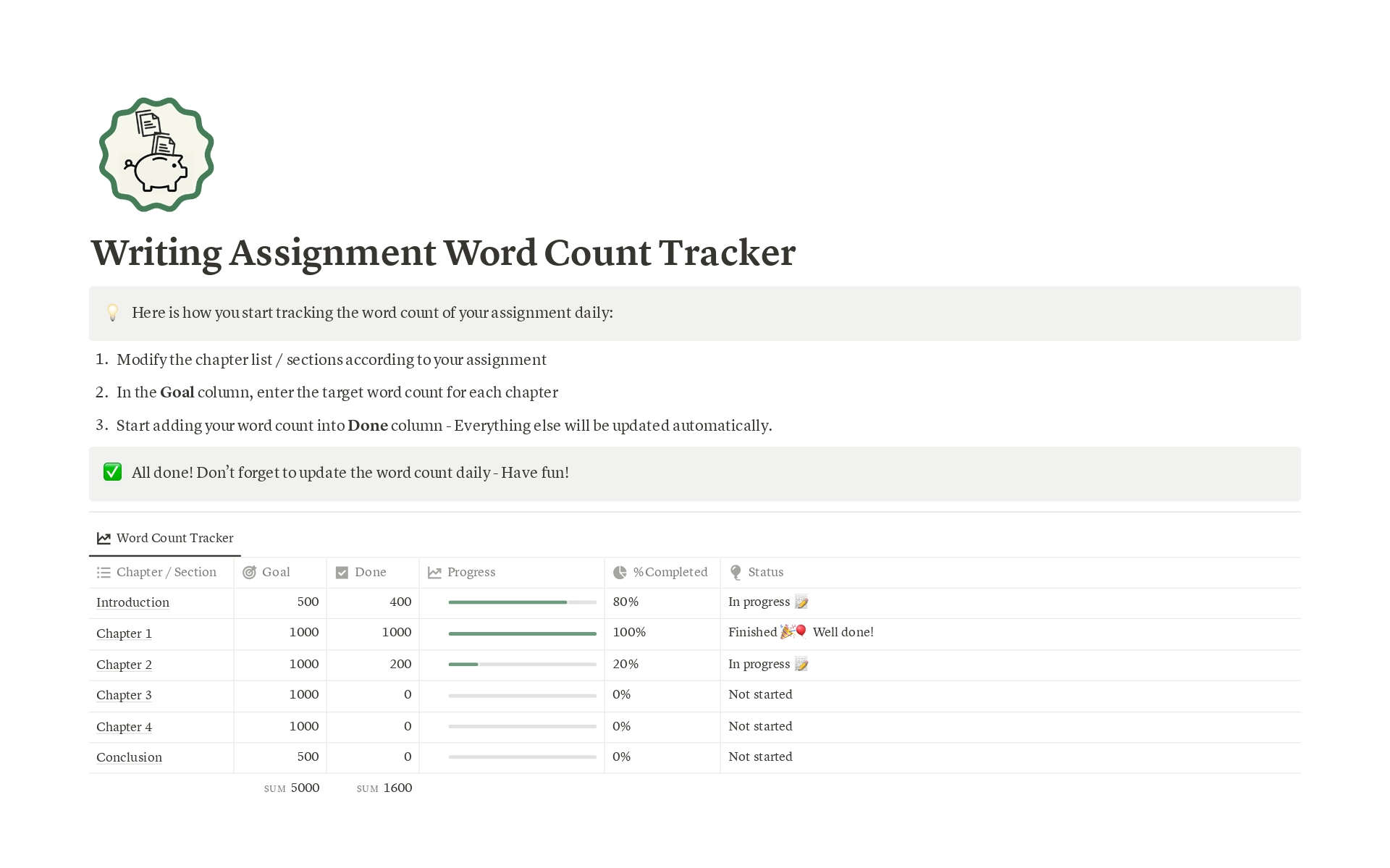
Writing Assignment Word Count Tracker
Simplicity Designs
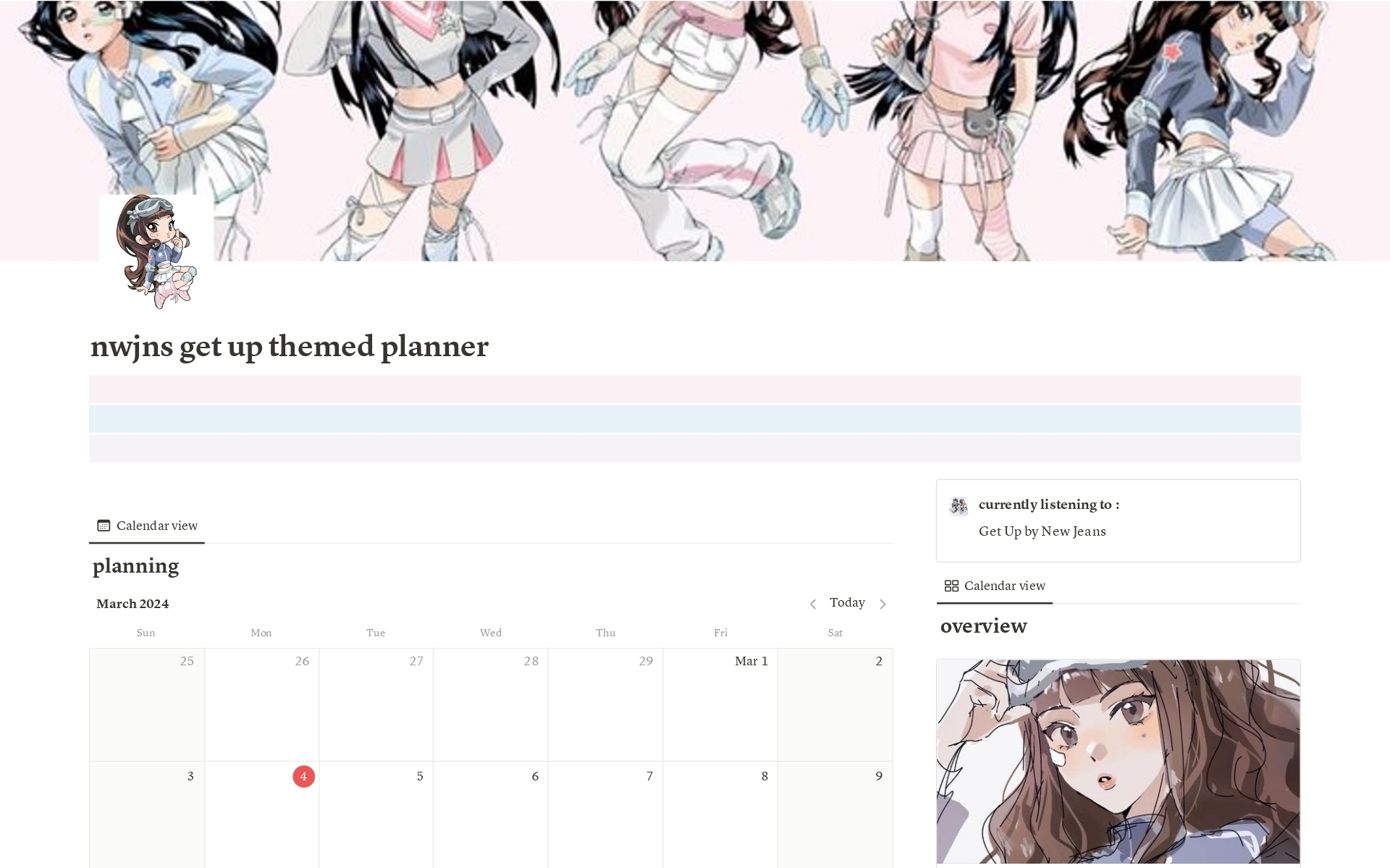
nwjns get up themed planner
Related Collections
Homework hero.

What is a Notion template?
A Notion template is any publicly shared page in Notion that can be duplicated. They allow you to share your favorite workflows with the community, or duplicate other workflows that you want to use.
How do I use a template?
Once you identify the template(s) you’d like to use, click the Start with this template or Get Template button. If you’re already signed into Notion, the template will automatically be added to your workspace in the Private section of your sidebar. If you’re logged out or don’t have a Notion account, you’ll be prompted to sign in or create an account first.
How do I make a Notion template?
You can make any Notion page a template by clicking Share in the top right, select the Publish tab, and click the Publish to web button. Make sure the Allow duplicate as template is toggled on. To share, use the public-facing URL or click the Copy web link button in the Publish tab.
How do I submit my template to the Notion Template Gallery?
To submit a template to the gallery, go to notion.so/templates and click the Submit a template button in the upper right corner or visit notion.so/submit-a-template . Fill out the form (including your public template link, template name, template descriptions, and template category) to share your template with the Notion community!
How can I customize the template?
Once you’ve added a template into your workspace, you have endless options for what you can change, edit, or update to fit your needs. Templates are just a starting point to help you create your ideal workspace.
Here are a few common updates and changes that you can make once duplicating a template:
Pages — Update the page cover photo, add/change an icon, and change the page title.
Text — Add formatting, like bold, italics, text color, and background color. Change heading levels, add bullet or numbered lists, and move sections around.
Blocks — Add blocks like callouts, toggle lists, or tables. Remove blocks that you don’t need to reduce clutter or make space for extra blocks.
Databases — Change property names, types, and icons, or add/remove properties that don’t fit your needs. Add new database views, like boards, lists, calendars, timelines, or galleries.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to do one-liner if else statement? [duplicate]
Please see https://golangdocs.com/ternary-operator-in-golang as pointed by @accdias (see comments)
Can I write a simple if-else statement with variable assignment in go (golang) as I would do in php? For example:
Currently I have to use the following:
Sorry I cannot remember the name if this control statement and I couldn't find the info in-site or through google search. :/
- if-statement
- conditional-operator
- 6 Its called a ternary operator ... and no, Go doesn't have one. – Simon Whitehead Commented Oct 24, 2014 at 10:11
- 6 I believe the word you're looking for is "ternary" – BenjaminRH Commented Oct 24, 2014 at 10:11
- 12 Just to clarify, a ternary operator is any operator of arity 3, that is any operator that binds 3 sub-expressions. C happens to have only one such operator. That's why it is usually called the ternary operator. Its real name is "conditional operator", though. – thwd Commented Oct 24, 2014 at 10:41
- Please, take a look at The ternary operator in GoLang . – accdias Commented Jun 30, 2021 at 14:01
12 Answers 12
As the comments mentioned, Go doesn't support ternary one liners. The shortest form I can think of is this:
But please don't do that, it's not worth it and will only confuse people who read your code.
- 115 @thoroc I'd actually probably avoid that in real life, as it is non intuitive IMHO, it's not worth saving 2 lines for less readability. – Not_a_Golfer Commented Oct 24, 2014 at 11:09
- 19 @thoroc, please listen to what Not_a_Golfer said. You should strive to write maintainable software, not show yourself off. Neat tricks are neat but the next guy reading your code (including you in a couple of months/years) won't appreciate them. – kostix Commented Oct 24, 2014 at 18:07
- 4 It depends, as far as readability. I think this is more readable, but like anything else, it can be abused. If you need to declare a variable that isn't needed outside of the if block, then I think this is a winner. – arjabbar Commented Jun 9, 2015 at 15:50
- 7 Oh, joy, look at how much Go gained by not having a ternary operator. Aside from the readability comments made, you've changed a lazily-evaluated construct where only one branch is ever run to one where the first is always run and the second may be run in addition. – itsbruce Commented Apr 1, 2017 at 7:57
- 2 @accdias no, it doesn't work in Go. – Not_a_Golfer Commented Jun 30, 2021 at 20:58
As the others mentioned, Go does not support ternary one-liners. However, I wrote a utility function that could help you achieve what you want.
Here are some test cases to show how you can use it
For fun, I wrote more useful utility functions such as:
If you would like to use any of these, you can find them here https://github.com/shomali11/util

- I prefer If(c bool).Then(a interface{}, b interface{}) from this answer , it looks more idiomatic for me – vladkras Commented Dec 8, 2020 at 11:47
- 1 What about: If(c bool).Then(a interface{}).Else(b interface{}) Can drop the else if only initial value required. even like this: If(c bool).Then(a interface{}).Default(b interface{}) – Cyberience Commented Dec 16, 2020 at 8:35
- Lovely! thanks for sharing. – earizon Commented Aug 17, 2021 at 15:13
- The parameters "a" and "b" must always be valid. You can't to check for nil s and contains in a slice. IfThenElse(len(a) > 1, a[1], a[0]) throw runtime error: index out of range [1] with length 1 – Geograph Commented Apr 19 at 15:51
I often use the following:
basically the same as @Not_a_Golfer's but using type inference .

- 10 With the same shortcoming: you complicate the understanding when using an asymmetric solution for an obviously symmetric requirement. – Wolf Commented Dec 13, 2016 at 7:48
- 4 And the same shortcoming of turning what should be a lazily-evaluated construct where only one of two branches will ever be used into one where one will always be evaluated, sometimes both (in which case the first evaluation was redundant) – itsbruce Commented Apr 1, 2017 at 8:01
- 1 Depending on the use-case, this still might be very useful. E.g. listeningPath := "production.some.com"; if DEBUG { listeningPath := "development.some.com" } Same speed as ternary for production, and imho pretty good readability. – Levite Commented Nov 9, 2017 at 11:42
- I usually cry when waste intentionally CPU cycle. – alessiosavi Commented Mar 3, 2020 at 22:17
Thanks for pointing toward the correct answer.
I have just checked the Golang FAQ (duh) and it clearly states, this is not available in the language:
Does Go have the ?: operator? There is no ternary form in Go. You may use the following to achieve the same result: if expr { n = trueVal } else { n = falseVal }
additional info found that might be of interest on the subject:
- Rosetta Code for Conditional Structures in Go
- Ternary Operator in Go experiment from this guy
- it's also possible in one line: var c int; if a > b { c = a } else { c = b } ? But I'd suggest keeping it in 5 lines to form a light block for the reader's recreation ;) – Wolf Commented Dec 13, 2016 at 7:38
One possible way to do this in just one line by using a map, simple I am checking whether a > b if it is true I am assigning c to a otherwise b
However, this looks amazing but in some cases it might NOT be the perfect solution because of evaluation order. For example, if I am checking whether an object is not nil get some property out of it, look at the following code snippet which will panic in case of myObj equals nil
Because map will be created and built first before evaluating the condition so in case of myObj = nil this will simply panic.
Not to forget to mention that you can still do the conditions in just one simple line, check the following:

- 2 Just think of all that effort you wasted with this approach. You allocated the memory, initialized a map, added two entries to it, fully evaluating both, then hashed the lookup value, looked up and then let the map be collected by GC. Yes, it's a one-liner, but does it worth it? It's even worse than doing Optional.ofNullable(x).ifPresent(...) in Java. – Alexey Nezhdanov Commented Aug 8, 2021 at 4:02
- I'd not use this but I like the fact that it is doable. 😂 – Anticro Commented Jul 25, 2023 at 14:41
A very similar construction is available in the language
Use lambda function instead of ternary operator
to give the max int
Suppose you have this must(err error) function to handle errors and you want to use it when a condition isn't fulfilled. (enjoy at https://play.golang.com/p/COXyo0qIslP )

- 2 interesting approach, It might be useful in more complex cases. :) – thoroc Commented Nov 26, 2019 at 13:50
Sometimes, I try to use anonymous function to achieve defining and assigning happen at the same line. like below:
https://play.golang.org/p/rMjqytMYeQ0
- This also might be useful in more complex cases +1. – user12817546 Commented Sep 12, 2020 at 2:39
Like user2680100 said, in Golang you can have the structure:
This is useful to shortcut some expressions that need error checking, or another kind of boolean checking, like:
With this you can achieve something like (in C):
But is evident that this sugar in Golang have to be used with moderation, for me, personally, I like to use this sugar with max of one level of nesting, like:
You can also implement ternary expressions with functions like func Ternary(b bool, a interface{}, b interface{}) { ... } but i don't like this approach, looks like a creation of a exception case in syntax, and creation of this "features", in my personal opinion, reduce the focus on that matters, that is algorithm and readability, but, the most important thing that makes me don't go for this way is that fact that this can bring a kind of overhead, and bring more cycles to in your program execution.

You can use a closure for this:
The only gripe I have with the closure syntax in Go is there is no alias for the default zero parameter zero return function, then it would be much nicer (think like how you declare map, array and slice literals with just a type name).
Or even the shorter version, as a commenter just suggested:
You would still need to use a closure if you needed to give parameters to the functions. This could be obviated in the case of passing methods rather than just functions I think, where the parameters are the struct associated with the methods.

- Shorter version doif(condition, dothis, dothat) – vellotis Commented Nov 26, 2018 at 18:44
- 1 Yes, that would be even shorter, passing just the functions. One only has to have this function once in one utility library, and you can use it all through your code. – Louki Sumirniy Commented Nov 27, 2018 at 19:16
- Why call the function instead of simply returning it? – GLRoman Commented Mar 17, 2021 at 22:19
As everyone else pointed out, there's no ternary operator in Go.
For your particular example though, if you want to use a single liner, you could use Max .

- 1 Looks like the people who voted down here did not think about the use-case. Thank you for your answer! – Anticro Commented Jul 25, 2023 at 14:46
Ternary ? operator alternatives | golang if else one line You can’t write a short one-line conditional in Go language ; there is no ternary conditional operator. Read more about if..else of Golang
- 3 Nothing new here. This has already been said in other answers, in a better way. – Eric Aya Commented Jan 17, 2021 at 13:25
- 2 Furthermore, self promotion without any real use. – Felix Schütz Commented Mar 26, 2021 at 15:55
Not the answer you're looking for? Browse other questions tagged go if-statement conditional-operator or ask your own question .
- The Overflow Blog
- One of the best ways to get value for AI coding tools: generating tests
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- How many engineers/scientists believed that human flight was imminent as of the late 19th/early 20th century?
- Why were there so many OSes that had the name "DOS" in them?
- "Famous award" - "on ships"
- What is a natural-sounding verb form for the word dorveille?
- Subject verb agreement - I as well as he is/am the culprit
- How to expand argument in the Expl3 command \str_if_eq?
- The meaning of an implication in an existential quantifier
- Is it possible to change the AirDrop location on my Mac without downloading random stuff from the internet?
- Why does Sfas Emes start his commentary on Parshat Noach by saying he doesn't know it? Is the translation faulty?
- Definition of annuity
- What does "break an arm" mean?
- Creating good tabularx
- Help updating 34 year old document to run with modern LaTeX
- Copyright Fair Use: Is using the phrase "Courtesy of" legally acceptable when no permission has been given?
- How to decrease by 1 integers in an expl3's clist?
- pseudo-periodic time series with stochastic period and amplitude
- What came of the Trump campaign's complaint to the FEC that Harris 'stole' (or at least illegally received) Biden's funding?
- Doesn't nonlocality follow from nonrealism in the EPR thought experiment and Bell tests?
- How much better is using quad trees than simple relational database for storing location data?
- cat file contents to clipboard over ssh and across different OS
- Look for mistakes!
- Why would the GPL be viral, while EUPL isn't, according to the EUPL authors?
- Why is resonance such a widespread phenomenon?
- How did people know that the war against the mimics was over?

IMAGES
VIDEO
COMMENTS
Assignation Global variables As in Go lang, you must initially declare your global variable using the := assignment operator and subsequent overwrite use the = operator. Razor expression Go Template Note @string := "string value"; {{- set $ "string" "string value" }} Global declare and assign of string @numeric1 := 10; {{- set $ "numeric1" 10 }} Global declare and assign of integer @numeric2 := 1.
This is the biggest (and only) problem I have with Go. I simply do not understand why this functionality has not been implemented yet. When the template package is used in a generic context (eg. Executing an arbitrary template file with an arbitrary json file), you might not have the luxury of doing precalculations.
Associated templates. Nested template definitions. Package template implements data-driven templates for generating textual output. To generate HTML output, see html/template, which has the same interface as this package but automatically secures HTML output against certain attacks. Templates are executed by applying them to a data structure.
The Go standard library provides a set of packages to generate output. The text/template package implements templates for generating text output, while the html/template package implements templates for generating HTML output that is safe against certain attacks. Both packages use the same interface but the following examples of the core features are directed towards HTML applications.
Sprig: Template functions for Go templates. The Go language comes with a built-in template language, but not very many template functions. Sprig is a library that provides more than 100 commonly used template functions. It is inspired by the template functions found in Twig and in various JavaScript libraries, such as underscore.js.
Template Actions and Nested Templates in Go. This article is part of a series. This is the second part in a four part series where we are learning about creating dynamic HTML and text files with Go's template packages. If you haven't already, I suggest you check out the first part of the series (linked above - look for "Previous Article").
Introduction. hugo templates are based on the go language, but are derived from the go template library. It is simple and relies on only basic logic. go templates are html documents whose variables and functions are accessible via {{-}}. For purposes of the blog, the templates are contained in the layout folder.
Go templates made easy. Florin Pățan. December 14, 2018. Go ships with its own template engine, split into two packages, text/template and html/template. These packages are similar in functionality, with the difference that html/template allows a user to generate HTML code that is safe against code injection, making it suitable for use on web ...
Golang provides the text/template and html/template packages for handling templates straight out of the box. The first package is the most general one — You can use it for creating templates for all kinds of text strings. The second package is more specific for HTML — it is handy for dealing with insecure variables in an HTML web environment.
The Template Data Structure. As mentioned, Go's Template is a recursive data type. Each Template instance is itself a collection made up of one or more Template instances. This structure is represented internally by the parse.Tree type. In this data structure, a child template can be embedded into multiple parent templates.
As mentioned by this answer, the scope of that variable "re-assignment" ends with the {{end}} block. Therefore using standard variables only there's no way around the problem and it should be solved inside the Go program executing the template. In some frameworks however this is not that easy (e.g. protoc-gen-gotemplate).
But bear with me. In go templates you can pass a single "argument" (pipeline in go parlance) to a "sub-template" defined block. But by creating a simple helper function you can pass as many arguments as you want. Simply add this function to your FuncMap: func(els ...any) []any { return els } And you'll be able to create constructs such as:
Template definitions must appear at the top level of the 426 template, much like global variables in a Go program. 427 428 The syntax of such definitions is to surround each template declaration with a 429 "define" and "end" action. 430 431 The define action names the template being created by providing a string 432 constant. Here is a simple ...
A template is a file in the layouts directory of a project, theme, or module. Templates use variables , functions, and methods to transform your content, resources, and data into a published page. Hugo uses Go's text/template and html/template packages. The text/template package implements data-driven templates for generating textual output ...
Template Method pattern in Go. Full code example in Go with detailed comments and explanation. Template Method is a behavioral design pattern that allows you to define a skeleton of an algorithm in a base class and let subclasses override the steps without changing the overall algorithm's structure.
Teacher On Special Assignment - TOSA - Co-teaching Template Download the Teacher On Special Assignment - TOSA - Co-teaching Template presentation for PowerPoint or Google Slides. The education sector constantly demands dynamic and effective ways to present information. This template is created with that very purpose in mind.
Prefix a function or method call with the go keyword to run the call in a new goroutine. When the call completes, the goroutine exits, silently. (The effect is similar to the Unix shell's & notation for running a command in the background.) go list.Sort() // run list.Sort concurrently; don't wait for it.
This is an opinionated Go project template you can use as a starting point for your project. It doesn't include any code generation, so you'll need to replace the placeholder variables/values/names with your own. Clone the repository, keep what you need and delete everything else! Feel free to replace the parts that don't align with your use ...
The problem is either in your Go code or in the part of the template that you've omitted. When asking questions on SO, you should strive to provide a minimal, complete, and verifiable example . - mkopriva
4 To first explain behavior and the practice of behavioral science lets go over the four related domains. It is explained well here (The science of behavior analysis and its application to human problems consists of four domains: the three branches of behavior analysis—radical behaviorism, EAB, and ABA—and professional practice in various fields that is informed and guided by that science.
To submit a template to the gallery, go to notion.so/templates and click the Submit a template button in the upper right corner or visit notion.so/submit-a-template. Fill out the form (including your public template link, template name, template descriptions, and template category) to share your template with the Notion community!
So layout.html gets rendered before home.html, so you cant pass a value back. In your example it would be the best solution to use a struct and pass it from the layout.html to the home.html using the dot: main.go. package main. import (. "html/template". "net/http". ) type WebData struct {.
12. One possible way to do this in just one line by using a map, simple I am checking whether a > b if it is true I am assigning c to a otherwise b. c := map[bool]int{true: a, false: b}[a > b] However, this looks amazing but in some cases it might NOT be the perfect solution because of evaluation order.