Python Programming

Practice Python Exercises and Challenges with Solutions
Free Coding Exercises for Python Developers. Exercises cover Python Basics , Data structure , to Data analytics . As of now, this page contains 18 Exercises.
What included in these Python Exercises?
Each exercise contains specific Python topic questions you need to practice and solve. These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges.
- All exercises are tested on Python 3.
- Each exercise has 10-20 Questions.
- The solution is provided for every question.
- Practice each Exercise in Online Code Editor
These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Select the exercise you want to solve .
Basic Exercise for Beginners
Practice and Quickly learn Python’s necessary skills by solving simple questions and problems.
Topics : Variables, Operators, Loops, String, Numbers, List
Python Input and Output Exercise
Solve input and output operations in Python. Also, we practice file handling.
Topics : print() and input() , File I/O
Python Loop Exercise
This Python loop exercise aims to help developers to practice branching and Looping techniques in Python.
Topics : If-else statements, loop, and while loop.
Python Functions Exercise
Practice how to create a function, nested functions, and use the function arguments effectively in Python by solving different questions.
Topics : Functions arguments, built-in functions.
Python String Exercise
Solve Python String exercise to learn and practice String operations and manipulations.
Python Data Structure Exercise
Practice widely used Python types such as List, Set, Dictionary, and Tuple operations in Python
Python List Exercise
This Python list exercise aims to help Python developers to learn and practice list operations.
Python Dictionary Exercise
This Python dictionary exercise aims to help Python developers to learn and practice dictionary operations.
Python Set Exercise
This exercise aims to help Python developers to learn and practice set operations.
Python Tuple Exercise
This exercise aims to help Python developers to learn and practice tuple operations.
Python Date and Time Exercise
This exercise aims to help Python developers to learn and practice DateTime and timestamp questions and problems.
Topics : Date, time, DateTime, Calendar.
Python OOP Exercise
This Python Object-oriented programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts.
Topics : Object, Classes, Inheritance
Python JSON Exercise
Practice and Learn JSON creation, manipulation, Encoding, Decoding, and parsing using Python
Python NumPy Exercise
Practice NumPy questions such as Array manipulations, numeric ranges, Slicing, indexing, Searching, Sorting, and splitting, and more.
Python Pandas Exercise
Practice Data Analysis using Python Pandas. Practice Data-frame, Data selection, group-by, Series, sorting, searching, and statistics.
Python Matplotlib Exercise
Practice Data visualization using Python Matplotlib. Line plot, Style properties, multi-line plot, scatter plot, bar chart, histogram, Pie chart, Subplot, stack plot.
Random Data Generation Exercise
Practice and Learn the various techniques to generate random data in Python.
Topics : random module, secrets module, UUID module
Python Database Exercise
Practice Python database programming skills by solving the questions step by step.
Use any of the MySQL, PostgreSQL, SQLite to solve the exercise
Exercises for Intermediate developers
The following practice questions are for intermediate Python developers.
If you have not solved the above exercises, please complete them to understand and practice each topic in detail. After that, you can solve the below questions quickly.
Exercise 1: Reverse each word of a string
Expected Output
- Use the split() method to split a string into a list of words.
- Reverse each word from a list
- finally, use the join() function to convert a list into a string
Steps to solve this question :
- Split the given string into a list of words using the split() method
- Use a list comprehension to create a new list by reversing each word from a list.
- Use the join() function to convert the new list into a string
- Display the resultant string
Exercise 2: Read text file into a variable and replace all newlines with space
Given : Assume you have a following text file (sample.txt).
Expected Output :
- First, read a text file.
- Next, use string replace() function to replace all newlines ( \n ) with space ( ' ' ).
Steps to solve this question : -
- First, open the file in a read mode
- Next, read all content from a file using the read() function and assign it to a variable.
- Display final string
Exercise 3: Remove items from a list while iterating
Description :
In this question, You need to remove items from a list while iterating but without creating a different copy of a list.
Remove numbers greater than 50
Expected Output : -
- Get the list's size
- Iterate list using while loop
- Check if the number is greater than 50
- If yes, delete the item using a del keyword
- Reduce the list size
Solution 1: Using while loop
Solution 2: Using for loop and range()
Exercise 4: Reverse Dictionary mapping
Exercise 5: display all duplicate items from a list.
- Use the counter() method of the collection module.
- Create a dictionary that will maintain the count of each item of a list. Next, Fetch all keys whose value is greater than 2
Solution 1 : - Using collections.Counter()
Solution 2 : -
Exercise 6: Filter dictionary to contain keys present in the given list
Exercise 7: print the following number pattern.
Refer to Print patterns in Python to solve this question.
- Use two for loops
- The outer loop is reverse for loop from 5 to 0
- Increment value of x by 1 in each iteration of an outer loop
- The inner loop will iterate from 0 to the value of i of the outer loop
- Print value of x in each iteration of an inner loop
- Print newline at the end of each outer loop
Exercise 8: Create an inner function
Question description : -
- Create an outer function that will accept two strings, x and y . ( x= 'Emma' and y = 'Kelly' .
- Create an inner function inside an outer function that will concatenate x and y.
- At last, an outer function will join the word 'developer' to it.
Exercise 9: Modify the element of a nested list inside the following list
Change the element 35 to 3500
Exercise 10: Access the nested key increment from the following dictionary
Under Exercises: -
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 52 Comments
Python Date and Time Exercise with Solutions
Updated on: December 8, 2021 | 10 Comments
Python Dictionary Exercise with Solutions
Updated on: May 6, 2023 | 57 Comments
Python Tuple Exercise with Solutions
Updated on: December 8, 2021 | 96 Comments
Python Set Exercise with Solutions
Updated on: October 20, 2022 | 28 Comments

Python if else, for loop, and range() Exercises with Solutions
Updated on: September 3, 2024 | 301 Comments
Updated on: August 2, 2022 | 158 Comments
Updated on: September 6, 2021 | 109 Comments
Python List Exercise with Solutions
Updated on: December 8, 2021 | 203 Comments
Updated on: December 8, 2021 | 7 Comments
Python Data Structure Exercise for Beginners
Updated on: December 8, 2021 | 116 Comments
Python String Exercise with Solutions
Updated on: October 6, 2021 | 222 Comments
Updated on: March 9, 2021 | 24 Comments
Updated on: March 9, 2021 | 52 Comments
Updated on: July 20, 2021 | 29 Comments
Python Basic Exercise for Beginners
Updated on: August 29, 2024 | 504 Comments
Useful Python Tips and Tricks Every Programmer Should Know
Updated on: May 17, 2021 | 23 Comments
Python random Data generation Exercise
Updated on: December 8, 2021 | 13 Comments
Python Database Programming Exercise
Updated on: March 9, 2021 | 17 Comments
- Online Python Code Editor
Updated on: June 1, 2022 |
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
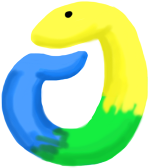
Practice Python
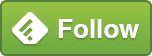
Beginner Python exercises
- Why Practice Python?
- Why Chilis?
- Resources for learners
All Exercises

All Solutions
- 1: Character Input Solutions
- 2: Odd Or Even Solutions
- 3: List Less Than Ten Solutions
- 4: Divisors Solutions
- 5: List Overlap Solutions
- 6: String Lists Solutions
- 7: List Comprehensions Solutions
- 8: Rock Paper Scissors Solutions
- 9: Guessing Game One Solutions
- 10: List Overlap Comprehensions Solutions
- 11: Check Primality Functions Solutions
- 12: List Ends Solutions
- 13: Fibonacci Solutions
- 14: List Remove Duplicates Solutions
- 15: Reverse Word Order Solutions
- 16: Password Generator Solutions
- 17: Decode A Web Page Solutions
- 18: Cows And Bulls Solutions
- 19: Decode A Web Page Two Solutions
- 20: Element Search Solutions
- 21: Write To A File Solutions
- 22: Read From File Solutions
- 23: File Overlap Solutions
- 24: Draw A Game Board Solutions
- 25: Guessing Game Two Solutions
- 26: Check Tic Tac Toe Solutions
- 27: Tic Tac Toe Draw Solutions
- 28: Max Of Three Solutions
- 29: Tic Tac Toe Game Solutions
- 30: Pick Word Solutions
- 31: Guess Letters Solutions
- 32: Hangman Solutions
- 33: Birthday Dictionaries Solutions
- 34: Birthday Json Solutions
- 35: Birthday Months Solutions
- 36: Birthday Plots Solutions
- 37: Functions Refactor Solution
- 38: f Strings Solution
- 39: Character Input Datetime Solution
- 40: Error Checking Solution
Browse Course Material
Course info.
- Sarina Canelake
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
A gentle introduction to programming using python, assignments.
If you are working on your own machine, you will probably need to install Python. We will be using the standard Python software, available here . You should download and install version 2.6.x, not 2.7.x or 3.x. All MIT Course 6 classes currently use a version of Python 2.6.
ASSN # | ASSIGNMENTS | SUPPORTING FILES |
---|---|---|
Homework 1 | Handout ( ) Written exercises ( ) | Code template ( ) |
Homework 2 | Handout ( ) Written exercises ( ) | Code template ( ) nims.py ( ) strings_and_lists.py ( ) |
Project 1: Hangman | Handout ( ) | hangman_template.py ( ) words.txt ( ) Optional extension: hangman_lib.py ( ) hangman_lib_demo.py ( ) |
Homework 3 | Handout ( ) Written exercises ( ) | Code template ( ) |
Homework 4 | Handout ( ) Written exercises ( ) | Graphics module documentation ( ) graphics.py ( ) — be sure to save this in the same directory where your code is saved! wheel.py ( ) rgb.txt ( ) The graphics.py package and documentation are courtesy of John Zelle, and are used with permission. |
Project 2: Conway’s game of life | Handout ( ) | game_of_life_template.py ( ) — download and save as game_of_life.py; be sure to save in the same directory as graphics.py |
Final project: Tetris | Handout ( ) | tetris_template.py ( ) — Download and save it in the same directory as graphics.py. Please edit this file, and place your code in the sections that say “YOUR CODE HERE”. |

You are leaving MIT OpenCourseWare
35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.
1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
12 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Recent Posts
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
My problem sets for CS50 Python 2022
aviiciii/cs50python
Folders and files.
Name | Name | |||
---|---|---|---|---|
17 Commits | ||||
Repository files navigation
Cs50 python problem sets.
This repository contains my solutions to the problem sets for CS50's Introduction to Programming with Python course. Each problem set is a collection of programming assignments that cover various concepts and topics in Python.
Table of Contents
Introduction, course information, installation, contributing.
This repository serves as a collection of my solutions to the problem sets for CS50's Introduction to Programming with Python course. Each problem set presents a set of programming assignments that allowed me to practice and demonstrate my understanding of different Python concepts and techniques.
CS50's Introduction to Programming with Python is an online course offered by Harvard University. It covers fundamental concepts of computer programming using the Python programming language. For more information about the course, please refer to the official CS50 website .
To run the problem sets locally or review the solutions, follow these steps:
- Clone the repository to your local machine.
- Navigate to the specific problem set folder you want to explore.
- Review the problem statement and requirements in the provided README or PDF file.
- Examine the solution code files to understand the implementation.
To use the solutions provided in this repository, you can follow these steps:
- Navigate to the specific problem set folder you are interested in.
- Examine the solution code files to understand the implementation or use them as a reference for your own work.
Contributions to this repository are generally not accepted, as it primarily serves as a collection of personal solutions to problem sets. However, if you have suggestions or improvements for the existing solutions, feel free to open an issue or submit a pull request.
This project is licensed under the MIT License . Please review the license file for more information.
Contributors 2
- Python 100.0%
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center
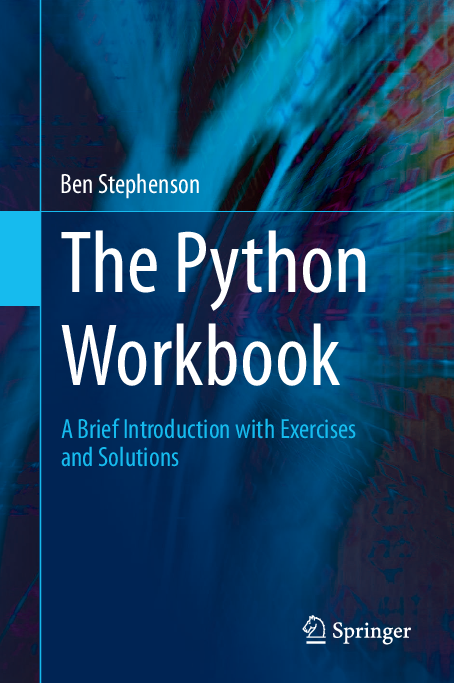
Download Free PDF
The Python Workbook A Brief Introduction with Exercises and Solutions

Related papers
a general overview of the most common algorithm and how to write them using python
If you wish to have receipt of your submission acknowledged, please include a selfaddressed, stamped envelope. Each problem and solution should be typed on separate sheets. Solutions to problems in this issue must be received by May 15, 2004- If a problem is not original, the proposer should inform the Problem Editor of the history of the problem. A problem should not be submitted elsewhere while it is under consideration for publication in this Journal. Solvers are asked to include references rather than quoting "well-known results".
Loading Preview
Sorry, preview is currently unavailable. You can download the paper by clicking the button above.
A Simple Introduction to Python, 2024
ACM SIGCSE Bulletin, 1985
ACM SIGCSE Bulletin, 1988
adrian, 2020
Python for Scientific Computing and Artificial Intelligence, 2023
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024

COMMENTS
To run a cell of code, click the "play button" icon to the left of the cell or click on the cell and press "Shift+Enter" on your keyboard. This will execute the Python code contained in the cell. Executing a cell that defines a variable is important before executing or authoring a cell that depends on that previously created variable assignment.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. Practice each Exercise in Online Code Editor. These Python programming exercises are suitable ...
WhatPythonistasSayAboutPython Basics: A Practical In- troductiontoPython3 "I love [the book]! The wording is casual, easy to understand, and makestheinformation @owwell. Ineverfeellostinthematerial,and
A Python Book A Python Book: Beginning Python, Advanced Python, and Python Exercises Author: Dave Kuhlman Contact: [email protected]
Beginner Python exercises. Home; Why Practice Python? Why Chilis? Resources for learners; All Exercises. 1: Character Input 2: Odd Or Even 3: List Less Than Ten 4: Divisors 5: List Overlap 6: String Lists 7: List Comprehensions 8: Rock Paper Scissors 9: Guessing Game One 10: List Overlap Comprehensions 11: Check Primality Functions 12: List Ends 13: Fibonacci 14: List Remove Duplicates
Welcome to your journey through Python Bookcamp: Exercises and Hand-on Projects. This is an introductory guide to the Python programming. Before you jump into the topics, I want to highlight a few points about the goal and the organization of the book. The primary goal of this book is to make you familiar with Python programming as quickly as ...
Assignment operators in Python assign the right-hand side values to the operand that is present on the left-hand side. The basic assignment operator is "=". For example, x = 5 assigns 5 to the variable x. Exercises Exercise 1: Hello World Print "Hello World" to the screen.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
This section provides the homework assignments and projects for the course along with handouts and supporting files. Assignments | A Gentle Introduction to Programming Using Python | Electrical Engineering and Computer Science | MIT OpenCourseWare
The re-written instructions are now embedded within the Jupyter Notebook along with the python starter code. For each assignment, all work is done solely within the notebook. The python assignments can be submitted for grading. They were tested to work perfectly well with the original Coursera grader that is currently used to grade the MATLAB ...
Ashwin Joy. I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology.
This repository contains assignments and their solutions for the course: Learn to Program: The Fundamentals. They were added after successfully completing the University of Toronto's non-credit...
Texas Summer Discovery Slideset 3: 5 Variables and Assignments. Naming Variables. Below are (most of) the rules for naming variables: Variable names must begin with a letter or underscore (\ ") character. After that, use any number of letters, underscores, or digits. Case matters: \score" is a di erent variable than \Score."
variety of tasks. Python is a true object-oriented language, and is available on a wide variety of platforms. There's even a python interpreter written entirely in Java, further enhancing python's position as an excellent solution for internet-based problems. Python was developed in the early 1990's by Guido van Rossum, then
Assignment: first evaluate right-hand side, then assign to the variable on the left-hand side. Consider the following code: total = 5 total = total + 1. Variables are only visible inside the function in which they are created (called "scope" of variable) If you create a variable in main(), its only visible in main()
To run the problem sets locally or review the solutions, follow these steps: Clone the repository to your local machine. Navigate to the specific problem set folder you want to explore. Review the problem statement and requirements in the provided README or PDF file. Examine the solution code files to understand the implementation.
the Python documentation (or it may be more intuitive to you to just create a Stack and a Queue class). Objects Complete this problem in a le called \pa1pr2.py": Develop an object for managing a game of Tic Tac Toe. While we covered the basics of creating objects in Python in class, you will probably want to refer to Python's documentation on ...
The Assignment Statement and Types Topics: Python's Interactive Mode Variables Expressions Assignment Strings, Ints, and Floats . ... In Python if one operand has type float and the other has type int, then the type int value is converted to float and the evaluation proceeds. >>> x = 30.
Download Free PDF. Download Free PDF. The Python Workbook A Brief Introduction with Exercises and Solutions. saket chaurasia. See full PDF download Download PDF. Related papers. Python Programming: An Introduction to Computer Science. Lara Alnajjar. a general overview of the most common algorithm and how to write them using python.