Monday, February 12, 2018
Groovy: basic concepts: variables, storage and commands, simple variables, composite variables, copy and reference, assignments, sequential commands, conditional commands, switch-case, iterative commands, each and eachwithindex, expressions with side effects, no comments:.
Post a Comment

Groovy Variable Scope
Last updated: February 13, 2024
It's finally here:
>> The Road to Membership and Baeldung Pro .
Going into ads, no-ads reading , and bit about how Baeldung works if you're curious :)
Azure Container Apps is a fully managed serverless container service that enables you to build and deploy modern, cloud-native Java applications and microservices at scale. It offers a simplified developer experience while providing the flexibility and portability of containers.
Of course, Azure Container Apps has really solid support for our ecosystem, from a number of build options, managed Java components, native metrics, dynamic logger, and quite a bit more.
To learn more about Java features on Azure Container Apps, visit the documentation page .
You can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Java applications have a notoriously slow startup and a long warmup time. The CRaC (Coordinated Restore at Checkpoint) project from OpenJDK can help improve these issues by creating a checkpoint with an application's peak performance and restoring an instance of the JVM to that point.
To take full advantage of this feature, BellSoft provides containers that are highly optimized for Java applications. These package Alpaquita Linux (a full-featured OS optimized for Java and cloud environment) and Liberica JDK (an open-source Java runtime based on OpenJDK).
These ready-to-use images allow us to easily integrate CRaC in a Spring Boot application:
Improve Java application performance with CRaC support
Modern software architecture is often broken. Slow delivery leads to missed opportunities, innovation is stalled due to architectural complexities, and engineering resources are exceedingly expensive.
Orkes is the leading workflow orchestration platform built to enable teams to transform the way they develop, connect, and deploy applications, microservices, AI agents, and more.
With Orkes Conductor managed through Orkes Cloud, developers can focus on building mission critical applications without worrying about infrastructure maintenance to meet goals and, simply put, taking new products live faster and reducing total cost of ownership.
Try a 14-Day Free Trial of Orkes Conductor today.
To learn more about Java features on Azure Container Apps, you can get started over on the documentation page .
And, you can also ask questions and leave feedback on the Azure Container Apps GitHub page .
Whether you're just starting out or have years of experience, Spring Boot is obviously a great choice for building a web application.
Jmix builds on this highly powerful and mature Boot stack, allowing devs to build and deliver full-stack web applications without having to code the frontend. Quite flexibly as well, from simple web GUI CRUD applications to complex enterprise solutions.
Concretely, The Jmix Platform includes a framework built on top of Spring Boot, JPA, and Vaadin , and comes with Jmix Studio, an IntelliJ IDEA plugin equipped with a suite of developer productivity tools.
The platform comes with interconnected out-of-the-box add-ons for report generation, BPM, maps, instant web app generation from a DB, and quite a bit more:
>> Become an efficient full-stack developer with Jmix
DbSchema is a super-flexible database designer, which can take you from designing the DB with your team all the way to safely deploying the schema .
The way it does all of that is by using a design model , a database-independent image of the schema, which can be shared in a team using GIT and compared or deployed on to any database.
And, of course, it can be heavily visual, allowing you to interact with the database using diagrams, visually compose queries, explore the data, generate random data, import data or build HTML5 database reports.
>> Take a look at DBSchema
Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push.
Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding.
Write code that works the way you meant it to:
>> CodiumAI. Meaningful Code Tests for Busy Devs
The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI .
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick.
Simplify Your Coding Journey with Machinet AI :
>> Install Machinet AI in your IntelliJ
Since its introduction in Java 8, the Stream API has become a staple of Java development. The basic operations like iterating, filtering, mapping sequences of elements are deceptively simple to use.
But these can also be overused and fall into some common pitfalls.
To get a better understanding on how Streams work and how to combine them with other language features, check out our guide to Java Streams:
Download the E-book
Do JSON right with Jackson
Get the most out of the Apache HTTP Client
Get Started with Apache Maven:
Working on getting your persistence layer right with Spring?
Explore the eBook
Building a REST API with Spring?
Get started with Spring and Spring Boot, through the Learn Spring course:
Explore Spring Boot 3 and Spring 6 in-depth through building a full REST API with the framework:
>> The New “REST With Spring Boot”
Get started with Spring and Spring Boot, through the reference Learn Spring course:
>> LEARN SPRING
Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework.
I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project .
You can explore the course here:
>> Learn Spring Security
1. Introduction
In this tutorial, we’ll talk about how to work with the different Groovy scopes and see how we can take advantage of its variable scope
2. Dependencies
Throughout, we’ll use the groovy-all and spock-core dependencies
3. All Scopes
Scopes in Groovy follow, above all, the rule that all variables are created public by default . This means that, unless specified, we’ll be able to access any variable we created from any other scope in the code.
We will see what these scopes mean and to tests this we will run Groovy scripts . To run a script we only need to run:
3.1. Global Variables
The easiest way to create a global variable in a Groovy script is to assign it anywhere in the script without any special keywords. We don’t even need to define the type:
Then, if we run the following groovy script:
we’ll see that we can reach our variable from the global scope.
3.2. Accessing Global Variables from Function Scope
Another way of accessing a global variable is by using the function scope:
This function is defined within the scope script. We add 1 to our global x variable.
If we run the following script:
We will get 201 as a result. This proves that we can access our global variable from the local scope of a function.
3.3. Creating Global Variables from a Function Scope
We can also create global variables from inside a function scope. In this local scope, if we don’t use any keyword in creating the variable, we’ll create it in the global scope. Let’s, then, create a global variable z in a new function:
and try and access it by running the following script:
We will see that we can access z from the global scope. So this finally proves that our variable has been created in the global scope.
3.4. Non-Global Variables
In the case of non-global variables, we’ll have a quick look at variables created for the local scope only.
Specifically, we’ll be looking at keyword def . This way, we define this variable to be part of the scope where the thread is running.
So, let’s try and define a global variable y and a function-local variable:
If we run this script, it will fail. The reason why it’s failing is that q is a local variable, which belongs to the scope of function fLocal . Since we create q with the def keyword, we won’t be able to access it via the global scope.
Evidently, we can access q using the fLocal function:
So now we can see that even though we have created one q variable, that variable isn’t available anymore in other scopes. If we call fLocal again, we’ll just create a new variable.
4. Conclusion
In this article, we have seen how Groovy scopes are created and how they can be accessed from different areas in the code.
As always, the full source code for the examples is available over on GitHub .
Baeldung Pro comes with both absolutely No-Ads as well as finally with Dark Mode , for a clean learning experience:
>> Explore a clean Baeldung
Once the early-adopter seats are all used, the price will go up and stay at $33/year.
Looking for the ideal Linux distro for running modern Spring apps in the cloud?
Meet Alpaquita Linux : lightweight, secure, and powerful enough to handle heavy workloads.
This distro is specifically designed for running Java apps . It builds upon Alpine and features significant enhancements to excel in high-density container environments while meeting enterprise-grade security standards.
Specifically, the container image size is ~30% smaller than standard options, and it consumes up to 30% less RAM:
>> Try Alpaquita Containers now.
Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE:
Basically, write code that works the way you meant it to.
AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code.
Get started with Spring Boot and with core Spring, through the Learn Spring course:
>> CHECK OUT THE COURSE
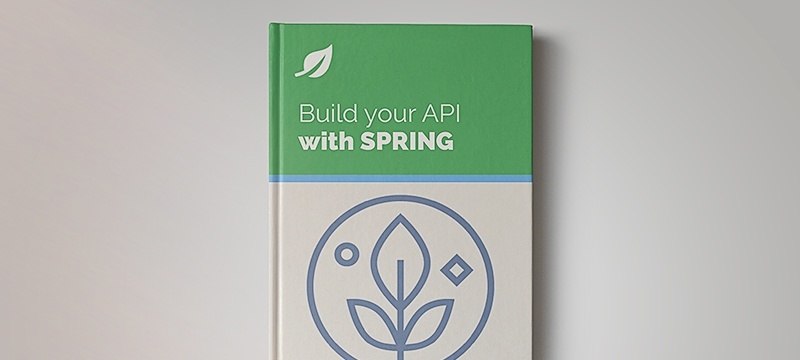
Variables are like containers which holds the data values. A variable specifies the name of the memory location.
How to declare variables
There are three ways to declare variables in Groovy.
- Native syntax similar to Java
- Using def keyword
- Variables in groovy do not require a type definition.
In the above syntax, assigning value is optional as you can just declare the variable and then assign value at later point in the program. Also, the value of a variable can be changed at any time.
Naming convention of variables
- Variable's name should consists of only letters (both uppercase and lowercase letters), digits and underscore( _ ).
- Variable's name cannot contain white spaces like first name which is a invalid variable name.
- First letter of a variable should be either a letter or an underscore( _ ).
- Variable names are case sensitive and hence Name and name both are different.
- It is always advisable to give some meaningful names to variables.
The above can also be written like below
Sample program:
Check result here.

September 1, 2009
Groovy goodness: multiple assignments.
Since Groovy 1.6 we can define and assign values to several variables at once. This is especially useful when a method returns multiple values and we want to assign them to separate variables.
- BI Publisher SQL
- Oracle Digital Assistant
- Oracle Visual Builder
- Oracle Intelligent Adviser
- Game Development
- Chrome Extensions
Field Value Assignment Methods
Values from fields are pulled into variables or even other fields by 2 possible methods:
1) Direct Assignment
The simplest and most direct way of getting the value of a field is by using the assignment operator to put the value from the field into a variable by utilizing the field API name.
On opportunities, the name of the opportunity has its API name as Name. We just do:
If we have a custom field, the API name of a custom field is FieldName_c (custom fields always have a _c )
The variable will take the data type of the field we assign to it. So if the field is a numeric field, the variable will also be a numeric variable.
2) Using a Get Method
Groovy in Sales Cloud provides several get methods to pull values from fields:
- getAttribute()
- getSelectedListDisplayValue()
- getSelectedListDisplayValues()
- getOriginalAttributeValue()
These methods belong to the Row class, which is part of the ADF framework on which Fusion is built.
Note: These get methods always return a String. This is important because, as we will learn in later tutorials, when assigning values to fields or variables, we must respect their data types.
From all the methods listed above, the most used are getAttribute() and getSelectedListDisplayValue() .
We use getAttribute() to pull values from all fields. For example, if we have a text field like the Name field of opportunities and we want to get its value, we would do:
If we want to assign this value to a variable, we would first define a variable and then use the assignment operator to put the value of the Name field into it:
Let's see another example:
If we are in, let's say, a trigger on the opportunity object and we want to get the value of a field called Comments, we type the following code:
When we want to save our work, we get this warning: Some fields whose value may be null are not protected by the nvl() function: Comments.
- Hello World in Groovy
- Groovy: read from console (STDIN, keyboard)
Groovy value types
- Groovy: Undeclared variable - runtime exception - groovy.lang.MissingPropertyException
- Groovy: Number guessing game
- Groovy: Determine type of an object
- Groovy: Lists
- Groovy: sum of numbers
- Groovy: color selector
- Groovy: read CSV file
- Count digits in Groovy
- Groovy: reading and writing files - appending content
- Groovy: listing the content of a directory, traversing a directory tree
- Groovy - Regular Expressions - regexes
- Groovy map (dictionary, hash, associative array)
- Groovy: JSON - reading and writing
- Groovy: Date, Time, Timezone
- Groovy: import and use functions from another file
- Groovy: Random numbers, random selection from list of values
- Groovy: Closures
- Groovy: remove spaces from a string
- Groovy: temporary file with autodelete
- Groovy: relative path
- Return multiple values from a function
- Groovy string length
- Groovy: substring
- Groovy: for loop - break - continue
- Groovy code reuse
- Groovy functions
- Groovy: evaluate code in another file
- Groovy classes
- Groovy function overloading
- Groovy variable scope
- Groovy recursive functions
- Groovy command line arguments (args)
- Groovy exit - System.exit - early exit from Groovy script
- Groovy file and directory attributes
- Groovy: join elements of an array
- Groovy pop push
- Groovy: Formatted printing with printf and sprintf
- Groovy System properties
- Groovy path to current executable script
- Groovy Exception handling (try, catch, Exception)
- Groovy throw (raise) exception
- Groovy casting
- Printing Unicode characters from Groovy
- Groovy: import standard libraries
- Groovy iterate over map keys
- Groovy get the list of keys of a map as an ArrayList
Declare variables with def
Declare variable as integer.
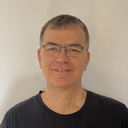
Published on 2018-05-27
Author: Gabor Szabo
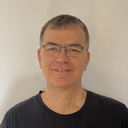

Get the Reddit app
A subreddit for all questions related to programming in any language.
Variable Assignment and Groovy : Or how i learned to hate code and start drinking.
First and foremost i want to list that i am not a developer.
I am an automation engineer and i spend 99% of my day writing code in PowerShell and Python.
I would consider myself an expert in PowerShell and competent in Python. I have had to start learning Groovy to put together some pipelines in Jenkins and i am running into some issues where variables are scoped in ways i don't seem to understand.
Below are some examples :
The output is :
When i extend this code two a second stage weird things start happening.
I get the following output :
Now, i am aware this might not be Groovy related and it may have something to with how jenkins implements Pipelines but why on earth does a variable i declare like value2 work in the next stage and the value i declare with 'def' not work in the next stage?
By continuing, you agree to our User Agreement and acknowledge that you understand the Privacy Policy .
Enter the 6-digit code from your authenticator app
You’ve set up two-factor authentication for this account.
Enter a 6-digit backup code
Create your username and password.
Reddit is anonymous, so your username is what you’ll go by here. Choose wisely—because once you get a name, you can’t change it.
Reset your password
Enter your email address or username and we’ll send you a link to reset your password
Check your inbox
An email with a link to reset your password was sent to the email address associated with your account
Choose a Reddit account to continue
1.1. Normal arithmetic operators
1.2. unary operators, 1.3. assignment arithmetic operators, 2. relational operators, 3.1. precedence, 3.2. short-circuiting, 4. bitwise operators, 5.1. not operator, 5.2. ternary operator, 5.3. elvis operator, 6.1. safe navigation operator, 6.2. direct field access operator, 6.3. method pointer operator, 7.1. pattern operator, 7.2. find operator, 7.3. match operator, 8.1.1. spreading method arguments, 8.1.2. spread list elements, 8.1.3. spread map elements, 8.2. range operator, 8.3. spaceship operator, 8.4. subscript operator, 8.5. membership operator, 8.6. identity operator, 8.7. coercion operator, 8.8. diamond operator, 8.9. call operator, 9. operator precedence, 10. operator overloading.
This chapter covers the operators of the Groovy programming language.
1. Arithmetic operators
Groovy supports the usual familiar arithmetic operators you find in mathematics and in other programming languages like Java. All the Java arithmetic operators are supported. Let’s go through them in the following examples.
The following binary arithmetic operators are available in Groovy:
Operator | Purpose | Remarks |
---|---|---|
| addition | |
| subtraction | |
| multiplication | |
| division | Use for integer division, and see the section about for more information on the return type of the division. |
| modulo | |
| power | See the section about for more information on the return type of the operation. |
Here are a few examples of usage of those operators:
The + and - operators are also available as unary operators:
Note the usage of parentheses to surround an expression to apply the unary minus to that surrounded expression. |
In terms of unary arithmetics operators, the ++ (increment) and -- (decrement) operators are available, both in prefix and postfix notation:
The postfix increment will increment after the expression has been evaluated and assigned into | |
The postfix decrement will decrement after the expression has been evaluated and assigned into | |
The prefix increment will increment before the expression is evaluated and assigned into | |
The prefix decrement will decrement before the expression is evaluated and assigned into |
From the binary arithmetic operators we have seen above, certain of them are also available in an assignment form:
Let’s see them in action:
Relational operators allow comparisons between objects, to know if two objects are the same or different, or if one is greater than, less than, or equal to the other.
The following operators are available:
Operator | Purpose |
---|---|
| equal |
| different |
| less than |
| less than or equal |
| greater than |
| greater than or equal |
Here are some examples of simple number comparisons using these operators:
3. Logical operators
Groovy offers three logical operators for boolean expressions:
&& : logical "and"
|| : logical "or"
! : logical "not"
Let’s illustrate them with the following examples:
"not" false is true | |
true "and" true is true | |
true "or" false is true |
The logical "not" has a higher priority than the logical "and".
Here, the assertion is true (as the expression in parentheses is false), because "not" has a higher precedence than "and", so it only applies to the first "false" term; otherwise, it would have applied to the result of the "and", turned it into true, and the assertion would have failed |
The logical "and" has a higher priority than the logical "or".
Here, the assertion is true, because "and" has a higher precedence than "or", therefore the "or" is executed last and returns true, having one true argument; otherwise, the "and" would have executed last and returned false, having one false argument, and the assertion would have failed |
The logical || operator supports short-circuiting: if the left operand is true, it knows that the result will be true in any case, so it won’t evaluate the right operand. The right operand will be evaluated only if the left operand is false.
Likewise for the logical && operator: if the left operand is false, it knows that the result will be false in any case, so it won’t evaluate the right operand. The right operand will be evaluated only if the left operand is true.
We create a function that sets the flag to true whenever it’s called | |
In the first case, after resetting the called flag, we confirm that if the left operand to is true, the function is not called, as short-circuits the evaluation of the right operand | |
In the second case, the left operand is false and so the function is called, as indicated by the fact our flag is now true | |
Likewise for , we confirm that the function is not called with a false left operand | |
But the function is called with a true left operand |
Groovy offers 4 bitwise operators:
& : bitwise "and"
| : bitwise "or"
^ : bitwise "xor" (exclusive "or")
~ : bitwise negation
Bitwise operators can be applied on a byte or an int and return an int :
bitwise and | |
bitwise and returns common bits | |
bitwise or | |
bitwise or returns all '1' bits | |
setting a mask to check only the last 8 bits | |
bitwise exclusive or on self returns 0 | |
bitwise exclusive or | |
bitwise negation |
It’s worth noting that the internal representation of primitive types follow the Java Language Specification . In particular, primitive types are signed, meaning that for a bitwise negation, it is always good to use a mask to retrieve only the necessary bits.
In Groovy, bitwise operators have the particularity of being overloadable , meaning that you can define the behavior of those operators for any kind of object.
5. Conditional operators
The "not" operator is represented with an exclamation mark ( ! ) and inverts the result of the underlying boolean expression. In particular, it is possible to combine the not operator with the Groovy truth :
the negation of is | |
'foo' is a non empty string, evaluating to , so negation returns | |
'' is an empty string, evaluating to , so negation returns |
The ternary operator is a shortcut expression that is equivalent to an if/else branch assigning some value to a variable.
Instead of:
You can write:
The ternary operator is also compatible with the Groovy truth , so you can make it even simpler:
The "Elvis operator" is a shortening of the ternary operator. One instance of where this is handy is for returning a 'sensible default' value if an expression resolves to false or null . A simple example might look like this:
with the ternary operator, you have to repeat the value you want to assign | |
with the Elvis operator, the value which is tested is used if it is not or |
Usage of the Elvis operator reduces the verbosity of your code and reduces the risks of errors in case of refactorings, by removing the need to duplicate the expression which is tested in both the condition and the positive return value.
6. Object operators
The Safe Navigation operator is used to avoid a NullPointerException . Typically when you have a reference to an object you might need to verify that it is not null before accessing methods or properties of the object. To avoid this, the safe navigation operator will simply return null instead of throwing an exception, like so:
will return a instance | |
use of the null-safe operator prevents from a | |
result is |
Normally in Groovy, when you write code like this:
public field | |
a getter for that returns a custom string | |
calls the getter |
The user.name call triggers a call to the property of the same name, that is to say, here, to the getter for name . If you want to retrieve the field instead of calling the getter, you can use the direct field access operator:
use of forces usage of the field instead of the getter |
The method pointer operator ( .& ) call be used to store a reference to a method in a variable, in order to call it later:
the variable contains a | |
we store a reference to the method on the instance inside a variable named | |
can be called like a regular method | |
we can check that the result is the same as if we had called it directly on |
There are multiple advantages in using method pointers. First of all, the type of such a method pointer is a groovy.lang.Closure , so it can be used in any place a closure would be used. In particular, it is suitable to convert an existing method for the needs of the strategy pattern:
the method takes each element of the list and calls the closure on them, returning a new list | |
we define a function that takes a a returns a | |
we create a method pointer on that function | |
we create the list of elements we want to collect the descriptors | |
the method pointer can be used where a was expected |
Method pointers are bound by the receiver and a method name. Arguments are resolved at runtime, meaning that if you have multiple methods with the same name, the syntax is not different, only resolution of the appropriate method to be called will be done at runtime:
define an overloaded method accepting a as an argument | |
define an overloaded method accepting an as an argument | |
create a single method pointer on , without specifying argument types | |
using the method pointer with a calls the version of | |
using the method pointer with an calls the version of |
7. Regular expression operators
The pattern operator ( ~ ) provides a simple way to create a java.util.regex.Pattern instance:
while in general, you find the pattern operator with an expression in a slashy-string, it can be used with any kind of String in Groovy:
using single quote strings | |
using double quotes strings | |
the dollar-slashy string lets you use slashes and the dollar sign without having to escape them | |
you can also use a GString! |
Alternatively to building a pattern, you can directly use the find operator =~ to build a java.util.regex.Matcher instance:
creates a matcher against the variable, using the pattern on the right hand side | |
the return type of is a | |
equivalent to calling |
Since a Matcher coerces to a boolean by calling its find method, the =~ operator is consistent with the simple use of Perl’s =~ operator, when it appears as a predicate (in if , while , etc.).
The match operator ( ==~ ) is a slight variation of the find operator, that does not return a Matcher but a boolean and requires a strict match of the input string:
matches the subject with the regular expression, but match must be strict | |
the return type of is therefore a | |
equivalent to calling |
8. Other operators
8.1. spread operator.
The Spread Operator ( *. ) is used to invoke an action on all items of an aggregate object. It is equivalent to calling the action on each item and collecting the result into a list:
build a list of items. The list is an aggregate of objects. | |
call the spread operator on the list, accessing the property of each item | |
returns a list of strings corresponding to the collection of items |
The spread operator is null-safe, meaning that if an element of the collection is null, it will return null instead of throwing a NullPointerException :
build a list for which of of the elements is | |
using the spread operator will throw a | |
the receiver might also be null, in which case the return value is |
The spread operator can be used on any class which implements the Iterable interface:
There may be situations when the arguments of a method call can be found in a list that you need to adapt to the method arguments. In such situations, you can use the spread operator to call the method. For example, imagine you have the following method signature:
then if you have the following list:
you can call the method without having to define intermediate variables:
It is even possible to mix normal arguments with spread ones:
When used inside a list literal, the spread operator acts as if the spread element contents were inlined into the list:
is a list | |
we want to insert the contents of the list directly into without having to call | |
the contents of has been inlined into |
The spread map operator works in a similar manner as the spread list operator, but for maps. It allows you to inline the contents of a map into another map literal, like in the following example:
is the map that we want to inline | |
we use the notation to spread the contents of into | |
contains all the elements of |
The position of the spread map operator is relevant, like illustrated in the following example:
is the map that we want to inline | |
we use the notation to spread the contents of into , but redefine the key *after spreading | |
contains all the expected keys, but was redefined |
Groovy supports the concept of ranges and provides a notation ( .. ) to create ranges of objects:
a simple range of integers, stored into a local variable | |
an , with inclusive bounds | |
an , with exclusive upper bound | |
a implements the interface | |
meaning that you can call the method on it |
Ranges implementation is lightweight, meaning that only the lower and upper bounds are stored. You can create a range from any Comparable object. For example, you can create a range of characters this way:
The spaceship operator ( ) delegates to the compareTo method:
The subscript operator is a short hand notation for getAt or putAt , depending on whether you find it on the left hand side or the right hand side of an assignment:
can be used instead of | |
if on left hand side of an assignment, will call | |
also supports ranges | |
so does | |
the list is mutated |
The subscript operator, in combination with a custom implementation of getAt / putAt is a convenient way for destructuring objects:
the class defines a custom implementation | |
the class defines a custom implementation | |
create a sample user | |
using the subscript operator with index 0 allows retrieving the user id | |
using the subscript operator with index 1 allows retrieving the user name | |
we can use the subscript operator to write to a property thanks to the delegation to | |
and check that it’s really the property which was changed |
The membership operator ( in ) is equivalent to calling the isCase method. In the context of a List , it is equivalent to calling contains , like in the following example:
equivalent to calling or |
In Groovy, using == to test equality is different from using the same operator in Java. In Groovy, it is calling equals . If you want to compare reference equality, you should use is like in the following example:
Create a list of strings | |
Create another list of strings containing the same elements | |
using , we test object equality | |
but using , we can check that references are distinct |
The coercion operator ( as ) is a variant of casting. Coercion converts object from one type to another without them being compatible for assignment. Let’s take an example:
is not assignable to a , so it will produce a at runtime |
This can be fixed by using coercion instead:
is not assignable to a , but use of will it to a |
When an object is coerced into another, unless the target type is the same as the source type, coercion will return a new object. The rules of coercion differ depending on the source and target types, and coercion may fail if no conversion rules are found. Custom conversion rules may be implemented thanks to the asType method:
the class defines a custom conversion rule from to | |
we create an instance of | |
we coerce the instance into an | |
the target is an instance of | |
the target is not an instance of anymore |
The diamond operator ( <> ) is a syntactic sugar only operator added to support compatibility with the operator of the same name in Java 7. It is used to indicate that generic types should be inferred from the declaration:
In dynamic Groovy, this is totally unused. In statically type checked Groovy, it is also optional since the Groovy type checker performs type inference whether this operator is present or not.
The call operator () is used to call a method named call implicitly. For any object which defines a call method, you can omit the .call part and use the call operator instead:
defines a method named . Note that it doesn’t need to implement | |
we can call the method using the classic method call syntax | |
or we can omit thanks to the call operator |
The table below lists all groovy operators in order of precedence.
Level | Operator(s) | Name(s) |
---|---|---|
1 |
| scope escape |
| new, explicit parentheses | |
| method call, closure, list/map | |
| dot, safe dereferencing, spread-dot | |
| bitwise negate, not, typecast | |
2 |
| power |
3 |
| pre/post increment/decrement, unary plus, unary minus |
4 |
| multiply, div, modulo |
5 |
| binary plus, binary minus |
6 |
| left/right (unsigned) shift, inclusive/exclusive range |
7 |
| less/greater than/or equal, instanceof, type coercion |
8 |
| equal, not equal, compare to |
9 |
| binary and |
10 |
| binary xor |
11 |
| binary or |
12 |
| logical and |
13 |
| logical or |
14 |
| ternary conditional |
15 |
| various assignments |
Groovy allows you to overload the various operators so that they can be used with your own classes. Consider this simple class:
implements a special method called |
Just by implementing the plus() method, the Bucket class can now be used with the + operator like so:
The two objects can be added together with the operator |
All (non-comparator) Groovy operators have a corresponding method that you can implement in your own classes. The only requirements are that your method is public, has the correct name, and has the correct number of arguments. The argument types depend on what types you want to support on the right hand side of the operator. For example, you could support the statement
by implementing the plus() method with this signature:
Here is a complete list of the operators and their corresponding methods:
Operator | Method | Operator | Method |
---|---|---|---|
| a.plus(b) |
| a.getAt(b) |
| a.minus(b) |
| a.putAt(b, c) |
| a.multiply(b) |
| a.leftShift(b) |
| a.div(b) |
| a.rightShift(b) |
| a.mod(b) |
| a.next() |
| a.power(b) |
| a.previous() |
| a.or(b) |
| a.positive() |
| a.and(b) |
| a.negative() |
| a.xor(b) |
| a.bitwiseNegative() |
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to assign values to array using groovy?
I want to extract the all name from response and assign it to array/list?
It is retrieving the first row, name (i.e) vicky only.. i wanted to extract name of all rows and assign it to list.. How to do this?

Change this line:
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged groovy or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- 3D printed teffilin?
- Background for the Elkies-Klagsbrun curve of rank 29
- Why is there no article after 'by'?
- Parody of Fables About Authenticity
- If inflation/cost of living is such a complex difficult problem, then why has the price of drugs been absoultly perfectly stable my whole life?
- How is it possible to know a proposed perpetual motion machine won't work without even looking at it?
- How to remove obligation to run as administrator in Windows?
- Has the US said why electing judges is bad in Mexico but good in the US?
- How much easier/harder would it be to colonize space if humans found a method of giving ourselves bodies that could survive in almost anything?
- Book or novel about an intelligent monolith from space that crashes into a mountain
- Does there always exist an algebraic formula for a function?
- Is there a nonlinear resistor with a zero or infinite differential resistance?
- My supervisor wants me to switch to another software/programming language that I am not proficient in. What to do?
- High voltage, low current connectors
- Could someone tell me what this part of an A320 is called in English?
- I overstayed 90 days in Switzerland. I have EU residency and never got any stamps in passport. Can I exit/enter at airport without trouble?
- How to count mismatches between two rows, column by column R?
- Whence “uniform distribution”?
- What unique phenomena would be observed in a system around a hypervelocity star?
- How do we reconcile the story of the woman caught in adultery in John 8 and the man stoned for picking up sticks on Sabbath in Numbers 15?
- How does the summoned monster know who is my enemy?
- Dutch Public Transportation Electronic Payment Options
- Cannot open and HTML file stored on RAM-disk with a browser
- What is the highest apogee of a satellite in Earth orbit?

IMAGES
VIDEO
COMMENTS
27. You just need parenthesis instead of brackets: def str = "xyz=abc". def (name, value) = str.split("=") Note that you'll need to know how many elements you're expecting or you'll have unexpected results. answered Mar 28, 2012 at 20:36. Eric Wendelin. 44k 9 71 94.
The switch statement in Groovy is backwards compatible with Java code; so you can fall through cases sharing the same code for multiple matches. One difference though is that the Groovy switch statement can handle any kind of switch value and different kinds of matching can be performed. def x = 1.23. def result = "".
Groovy supports multiple assignment, i.e. where multiple variables can be assigned at once, e.g.: def (a, b, c) = [10, 20, 'foo'] assert a == 10 && b == 20 && c == 'foo' ... One difference though is that the Groovy switch statement can handle any kind of switch value and different kinds of matching can be performed.
Assignments Groovy supports both single and multiple variable assignment. int a = 1 def b = c = 2 Sequential Commands ... The switch statement can accept a single variable and jump to a specific code block indexed with a specific value. Unlike Java, Groovy can perform a broader set of matching in the case statement, including strings, types and ...
Scopes in Groovy follow, above all, the rule that all variables are created public by default. This means that, unless specified, we'll be able to access any variable we created from any other scope in the code. We will see what these scopes mean and to tests this we will run Groovy scripts. To run a script we only need to run:
This operator is a shorthand for an assignment where we want to assign a value to a variable or property if the variable or property is null or false (following Groovy truth). If the value of the variable or property is not null or false (again apply Groovy truth rules), the value stays the same. In the following example code we use the elvis ...
Use an assignment to set a new value for the third element of the list: 4: ... Groovy has always supported literal list/array definitions using square brackets and has avoided Java-style curly braces so as not to conflict with closure definitions. In the case where the curly braces come immediately after an array type declaration however, there ...
There are three ways to declare variables in Groovy. Native syntax similar to Java. data-type variable-name = value; Using def keyword. def variable-name = value; Variables in groovy do not require a type definition. variable-name = value; // no type definition is required. In the above syntax, assigning value is optional as you can just ...
Groovy Goodness: Multiple Assignments. Since Groovy 1.6 we can define and assign values to several variables at once. This is especially useful when a method returns multiple values and we want to assign them to separate variables. // Assign and declare variables.
Use an assignment to set a new value for the third element of the list: 4: ... Groovy has always supported literal list/array definitions using square brackets and has avoided Java-style curly braces so as not to conflict with closure definitions. In the case where the curly braces come immediately after an array type declaration however, there ...
Groovy supports the usual familiar arithmetic operators you find in mathematics and in other programming languages like Java. ... The binary arithmetic operators we have seen above are also available in an assignment form: ... The ternary operator is a shortcut expression that is equivalent to an if/else branch assigning some value to a ...
Constructor usage, using coercion in assignment: 3.1.2. Named parameters ... Methods in Groovy always return some value. If no return statement is provided, the value evaluated in the last line executed will be returned. For instance, note that none of the following methods uses the return keyword.
Field Value Assignment Methods. Values from fields are pulled into variables or even other fields by 2 possible methods: 1) Direct Assignment. The simplest and most direct way of getting the value of a field is by using the assignment operator to put the value from the field into a variable by utilizing the field API name.
Groovy provides native support for various collection types, including lists ... This can be confusing if you define a variable named a and that you want the value of a to be the key in your map. If this is the case, then you must escape the key by adding parenthesis, ...
Groovy value types. Groovy: Undeclared variable - runtime exception - groovy.lang.MissingPropertyException. Groovy: reading and writing files - appending content. Groovy: listing the content of a directory, traversing a directory tree. Groovy: Random numbers, random selection from list of values.
3. "Yes, the "?:" operator will return the value to the left, if it is not null." - That is incorrect. There are non-null values for which the operator will return the value to the right (empty list, empty String, number zero, false, etc.). The operator returns the value on the left if the value on the left evaluates to "true" per Groovy truth ...
Test on 1st node 1st stage [Pipeline] echo Value 1 should be test value 1 [Pipeline] echo Value 2 should be test value 2 [Pipeline] echo Value 3 should be test value 3 [Pipeline] echo Value 4 should be test value 4
1: We create a function that sets the called flag to true whenever it's called: 2: In the first case, after resetting the called flag, we confirm that if the left operand to || is true, the function is not called, as || short-circuits the evaluation of the right operand: 3: In the second case, the left operand is false and so the function is called, as indicated by the fact our flag is now true
I want to extract the all name from response and assign it to array/list? Code: responseContentParsed = new JsonSlurper().parseText( context.responseContent ) context.mptuValidAlias = responseContentParsed.name[0] log.info(context.mptuValidAlias) It is retrieving the first row, name (i.e) vicky only.. i wanted to extract name of all rows and ...