- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary

Compound assignment operators in C#
A compound assignment operator has a shorter syntax to assign the result. The operation is performed on the two operands before the result is assigned to the first operand.
The following are the compound assignment operators in C#.
Sr.No | Operator & Operator Name |
---|---|
1 | Addition Assignment |
2 | Subtraction Assignment |
3 | Multiplication Assignment |
4 | Division Assignment |
5 | Modulo Assignment |
6 | Bitwise AND Assignment |
7 | Bitwise OR Assignment |
8 | Bitwise XOR Assignment |
9 | Left Shift Assignment |
10 | Right Shift Assignment |
11 | Lambda Operator |
Let us see an example to learn how to work with compound assignment operators in C#.
Live Demo

- Related Articles
- Compound Assignment Operators in C++
- Compound assignment operators in Java\n
- Perl Assignment Operators
- Assignment operators in Dart Programming
- Compound operators in Arduino
- What is the difference between = and: = assignment operators?
- Passing the Assignment in C++
- Airplane Seat Assignment Probability in C++
- Copy constructor vs assignment operator in C++
- Unary operators in C/C++
- Ternary Operators in C/C++
- # and ## Operators in C ?
- Operators Precedence in C++
- Unary operators in C++
- Conversion Operators in C++
Kickstart Your Career
Get certified by completing the course
C# 8 - Null coalescing/compound assignment
There are a lot of cool new features in C# 8 and one of my favorites is the new Null coalescing assignment operator.
Stefán Jökull Sigurðarson
Hi friends.
There are a lot of cool new features in C# 8 and one of my favorites is the new Null coalescing assignment (or compound assignment, whichever you prefer) operator. If you have ever written code like this:
You can simplify this code A LOT!
That is the new ??= operator in action, which takes care of doing the null check and assignment for you in one sweet syntactic sugar-rush! I also find it more readable, but some people might disagree, and that fine. Just pick whatever you prefer :)
And the best part is that it actually compiles down to the same efficient code! Just take a look at this sharplab.io sample to see what I mean.
You might have solved this with a Lazy<string> or by using something like _someValue = _someValue ?? InitializeMyValue(); as well but that's still more code to write than the new operator and less efficient as well. The Lazy<T> approach has some overhead and the null-coalescing operator + assignment has the added inefficiency of always making the variable assignment even if there is no need to (you can take a closer look at the ASM part of the SharpLab example above) but there it is for reference:
Hope this helps! :)
Sign up for more like this.

- Introduction to C#
- Download and Install Visual Studio
- The First C# Program
- C# Data Types
- C# variables, keywords , constants
- C# If Statement
- C# If Else Statement
- C# Else If Statement
- C# Nested If Statement
- C# While Loop
- C# Do While Loop
- C# Break Statement
- C# Continue Statement
- C# Switch Statement
- C# Goto Statement
- C# Arithmetic Operator
- C# Increment Operator
- C# Decrement Operator
- C# Compound Assignment Operator
- C# Relational Operators
- C# Logical Operators
- C# Conditional Operator
- C# is Operator
- C# 2D Array
- C# 3D Array
- C# Jagged Array
- C# Class and its members
- C# Class Member Access Modifiers
- C# Interface
- C# Abstract Class
- C# Method Return Types
- C# This Keyword
- C# Static Keyword
- C# Polymorphism
- C# Inheritance
- C# Constructors
- C# Destructors
- C# Method Overloading
- C# Method Overriding
- C# Call By Value
- C# Call By Reference
- C# ref parameter
- C# out parameter
- C# Recursion
- C# Garbage Collection
- C# String Intern Pool
- C# String Immutability
- C# String Methods
- C# String Comparison with ==
- C# String Concat()
- C# String Trim()
- C# String ToUpper()
- C# String ToLower()
- C# String StartsWith
- C# String EndsWith()
- C# String Replace()
- C# String Insert()
- C# String Intern()
- C# String Substring()
- C# ToString() Method
- C# String LastIndexOf()
- C# String Equals()
- C# String Split()
- C# String Remove()
- C# String Contains()
- C# String Join()
- C# What is Exception?
- C# Exception Propagation
- C# Try-Catch block
- C# Multiple Catch Blocks
- C# Nested TryCatch Block
- C# Finally block
- C# Throw Keyword
- C# Checked and Unchecked Keywords
- C# User Defined Exception
- C# FileStream
- C# FileMode and FileAccess
- C# StreamWriter
- C# StreamReader
- C# TextWriter
- C# TextReader
- C# StringWriter
- C# StringReader
- C# BinaryWriter
- C# BinaryReader
- C# File Class
- C# Directory Class
- C# MemoryStream
- C# Random Access Files
- C# Serialization and Deserialization
- C# Lifecycle of Thread
- C# Creating a Thread
- C# Passing an Argument to Thread
- C# Creating Multiple Threads
- C# Thread Priorities
- C# Thread Join() Method
- C# Thread Interrupt() Method
- C# ThreadStateException in Thread
- C# Thread Abort() Method
- C# Thread Synchronization
- C# Interthread Communication
- C# Deadlock in Threads
- C# ArrayList
- C# Hashtable
- C# SortedList
- C# Generic SortedList
- C# Generic Stack
- C# Generic Queue
- C# Generic LinkedList
- C# Generic List
- C# Generic HashSet
- C# Generic Dictionary
Advertisement
+= operator
- Add operation.
- Assignment of the result of add operation.
- Understanding += operator with a code -
- Statement i+=2 is equal to i=i+2 , hence 2 will be added to the value of i, which gives us 4.
- Finally, the result of addition, 4 is assigned back to i, updating its original value from 2 to 4.
- A special case scenario for all the compound assigned operators
- All compound assignment operators perform implicit casting.
- Casting the char value ( smaller data type ) to an int value( larger data type ), so it could be added to an int value, 2.
- Finally, the result of performing the addition resulted in an int value, which was casted to a char value before it could be assigned to a char variable, ch .
Example with += operator
-= operator.
- Subtraction operation.
- Assignment of the result of subtract operation.
- Statement i-=2 is equal to i=i-2 , hence, 2 will be subtracted from the value of i, which gives us 0.
- Finally, the result of subtraction i.e. 0 is assigned back to i, updating its value to 0.
Example with -= operator
*= operator.
- Multiplication operation.
- Assignment of the result of multiplication operation.
- Statement i*=2 is equal to i=i*2 , hence 2 will be multiplied with the value of i, which gives us 4.
- Finally, the result of multiplication, 4 is assigned back to i, updating its value to 4.
Example with *= operator
/= operator.
- Division operation.
- Assignment of the result of division operation.
- Statement i/=2 is equal to i=i/2 , hence 4 will be divided by the value of i, which gives us 2.
- Finally, the result of division i.e. 2 is assigned back to i, updating its value from 4 to 2.
Example with /= operator
%= operator.
- Modulus operation, which finds the remainder of a division operation.
- Assignment of the result of modulus operation.
- Statement i%=2 is equal to i=i%2 , hence 4 will be divided by the value of i and its remainder gives us 0.
- Finally, the result of this modulus operation i.e. 0 is assigned back to i, updating its value from 4 to 0.
Example with %= operator
Please share this article -.

Please Subscribe

Notifications
Please check our latest addition C#, PYTHON and DJANGO
© Copyright 2020 Decodejava.com. All Rights Reserved.

DEV Community

Posted on Jan 5, 2020
Coalescing operator and Compound assignment operator in C#
Hello! Today i want to ask you this: Have you ever used a Null Coalescing operator or even a Compound assignment operator in C# ?
Until today i had never heard about this things, so i want to share with you what i learned about and how it can be applied to your code.
The problem
Let's say you want to give a given variable the value of null.
Now, if we want to print the value on the screen it will accuse the following error:
Let's see how to get around this...
The old way 👎
The old and 'commom way' to check this is using if else operators like this:
We see that in this case we cannot place a default value to x and y operators. So we display on screen when it is null.
The Null Coalescing operator way 👌
First, a null coalescing operator (??) is used to define a default value for nullable value types or reference types. It returns the left-hand operand if the operand is not null, otherwise, it returns the right operand.
So it's mainly used to simplify checking for null values and also assign a default value to a variable when the value is null.
Using our example:
This way i can make a default value on x and y when one of them is null. And so, we can print on screen!
But can it be better?
The Compound assignment operator way 😎
The Compound assignment operator (??=) was introduced on C# 8.0 and has made our job easier. It simply reduces what we have to write and has the same result.
Instead of writing double x = x ?? 0.0; We can just write double x ??= 0.0;
Simple, right?
Hope you enjoyed this post, it's simple but it's something worth sharing for me.
Thanks for your time!😊
Links: https://dzone.com/articles/nullable-types-and-null-coalescing-operator-in-c
https://dev.to/mpetrinidev/the-null-coalescing-operator-in-c-8-0-4ib4
https://docs.microsoft.com/pt-br/dotnet/csharp/language-reference/operators/null-coalescing-operator
Top comments (3)
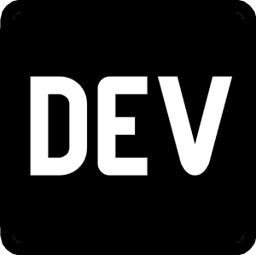
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location São José dos Campos
- Work Cloud Engineer | AWS Community Builder
- Joined May 21, 2019

- Joined Dec 16, 2017
Good article. Thank you.

- Location Brazil
- Work Intern at Agrotools
- Joined Oct 22, 2019
Awesome Dude, simple but unknown by most programmers
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
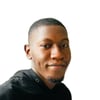
Beginner's Guide to Test Coverage with NUnit, Coverlet, and ReportGenerator
Olabamiji Oyetubo - Jun 17
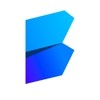
Top 5 Best C# Books for Beginners in 2024
ByteHide - Jun 17
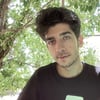
Cancellation Tokens in C#
Rasheed K Mozaffar - Jun 11
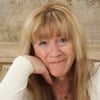

SQL-Server add record tip
Karen Payne - Jun 16
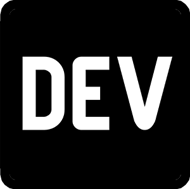
We're a place where coders share, stay up-to-date and grow their careers.
Compound Assignment in
About compound assignment.
Many operators can also be used as compound assignments , which are a shorthand notation where x = op y can be written as x op= y :
C# Compound Assignments
The core statement of the solution is as follows:
Compound Assignments
In this code, you use the compound assignment operator (+=), which is a shortcut that does the same thing as the previous solution.
You will see compound assignments in all C-family programming languages.
A program that illustrates compound assignment in connection with subtraction, multiplication, and division.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?? and ??= operators - the null-coalescing operators
- 9 contributors
The null-coalescing operator ?? returns the value of its left-hand operand if it isn't null ; otherwise, it evaluates the right-hand operand and returns its result. The ?? operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null. The null-coalescing assignment operator ??= assigns the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . The ??= operator doesn't evaluate its right-hand operand if the left-hand operand evaluates to non-null.
The left-hand operand of the ??= operator must be a variable, a property , or an indexer element.
The type of the left-hand operand of the ?? and ??= operators can't be a non-nullable value type. In particular, you can use the null-coalescing operators with unconstrained type parameters:
The null-coalescing operators are right-associative. That is, expressions of the form
are evaluated as
The ?? and ??= operators can be useful in the following scenarios:
In expressions with the null-conditional operators ?. and ?[] , you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null :
When you work with nullable value types and need to provide a value of an underlying value type, use the ?? operator to specify the value to provide in case a nullable type value is null :
Use the Nullable<T>.GetValueOrDefault() method if the value to be used when a nullable type value is null should be the default value of the underlying value type.
You can use a throw expression as the right-hand operand of the ?? operator to make the argument-checking code more concise:
The preceding example also demonstrates how to use expression-bodied members to define a property.
You can use the ??= operator to replace the code of the form
with the following code:
Operator overloadability
The operators ?? and ??= can't be overloaded.
C# language specification
For more information about the ?? operator, see The null coalescing operator section of the C# language specification .
For more information about the ??= operator, see the feature proposal note .
- Null check can be simplified (IDE0029, IDE0030, and IDE0270)
- C# operators and expressions
- ?. and ?[] operators
- ?: operator
Additional resources
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Why it is not possible to overload compound assignment operator in C#?
The title is be misleading, so please read entire question :-) .
By "compound assignment operator" I have in mind a construct like this op= , for example += . Pure assignment operator ( = ) does not belong to my question.
By "why" I don't mean an opinion, but resource (book, article, etc) when some of the designers, or their coworkers, etc. express their reasoning (i.e. the source of the design choice).
I am puzzled by asymmetry found in C++ and C# ( yes, I know C# is not C++ 2.0 ) -- in C++ you would overload operator += and then almost automatically write appropriate + operator relying on previously defined operator. In C# it is in reverse -- you overload + and += is synthesised for you.
If I am not mistaken the later approach kills a chance for optimization in case of actual += , because new object has to be created. So there has to be some big advantage of such approach, but MSDN is too shy to tell about it.
And I wonder what that advantage is -- so if you spotted explanation in some C# book, tech-talk video, blog entry, I will be grateful for the reference.
The closest thing I found is a comment on Eric Lippert blog Why are overloaded operators always static in C#? by Tom Brown. If the static overloading was decided first it simply dictates which operators can be overloaded for structs. This further dictates what can be overloaded for classes.
- language-design

- 3 Do you have a link for the new C++ style? Naively, overloading += first seems absurd; why would you overload a combined operation rather than the parts of it? – Telastyn Commented May 30, 2015 at 16:18
- 1 I believe the term for these is "compound assignment operators". – Ixrec Commented May 30, 2015 at 16:30
- 2 @greenoldman Telastyn was probably implying that implementing += in terms of + seems more natural than the other way around, since semantically += is a composition of + and =. To the best of my knowledge , having + call += is largely an optimization trick. – Ixrec Commented May 30, 2015 at 16:33
- 1 @Ixrec: Sometimes, a+=b makes sense, while a=a+b doesn't: Consider that identity might be important, an A cannot be duplicated, whatever. Sometimes, a=a+b makes sense, while a+=b doesn't: Consider immutable strings. So, one actually needs the ability to decide which one to overload separately. Of course, auto-generating the missing one, if all neccessary building-blocks exist and it's not explicitly disables, is a good idea. Not that C# allows that atm, afaik. – Deduplicator Commented May 30, 2015 at 16:40
- 4 @greenoldman - I understand that motivation, but personally, I would find *= mutating a reference type to be semantically incorrect. – Telastyn Commented May 30, 2015 at 17:52
I can't find the reference for this, but my understanding was that the C# team wanted to provide a sane subset of operator overloading. At the time, operator overloading had a bad rap. People asserted that it obfuscated code, and could only be used for evil. By the time C# was being designed, Java had shown us that no operator overloading was kind of annoying.
So C# wanted to balance operator overloading so that it was more difficult to do evil, but you could make nice things too. Changing the semantics of assignment was one of those things that was deemed always evil. By allowing += and its kin to be overloaded, it would allow that sort of thing. For example, if += mutated the reference rather than making a new one, it wouldn't follow the expected semantics, leading to bugs.

- Thank you, if you don't mind I will keep this question open bit longer, and if nobody beat you with the reasoning of actual design, I will close it with accepting yours. Once again -- thank you. – greenoldman Commented May 30, 2015 at 18:29
- @greenoldman - as well you should. I too hope someone come with a answer with more concrete references. – Telastyn Commented May 30, 2015 at 20:04
- So, this is a place where the impossibility of talking both about the pointer and the pointee comfortably and unambiguously rears it's head? Damn shame. – Deduplicator Commented May 30, 2015 at 21:15
- "People asserted that it obfuscated code" - it's still true though, have you seen some of the F# libraries? Operator noise. E.g. quanttec.com/fparsec – Den Commented Jun 3, 2015 at 9:39
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# language-design operators or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Is 3 Ohm resistance value of a PCB fuse reasonable?
- Could someone tell me what this part of an A320 is called in English?
- 3D printed teffilin?
- Memory-optimizing arduino code to be able to print all files from SD card
- Where to donate foreign-language academic books?
- What unique phenomena would be observed in a system around a hypervelocity star?
- How can judicial independence be jeopardised by politicians' criticism?
- Image Intelligence concerning alien structures on the moon
- Cannot open and HTML file stored on RAM-disk with a browser
- Parse Minecraft's VarInt
- Is there a way to resist spells or abilities with an AOE coming from my teammates, or exclude certain beings from the effect?
- A short story about a boy who was the son of a "normal" woman and a vaguely human denizen of the deep
- Trying to install MediaInfo Python 3.13 (Ubuntu) and it is not working out as expected
- If inflation/cost of living is such a complex difficult problem, then why has the price of drugs been absoultly perfectly stable my whole life?
- I am a sailor. I am planning to call my family onboard with me on the ship from the suez
- What's the meaning of "running us off an embankment"?
- How did Oswald Mosley escape treason charges?
- Encode a VarInt
- My supervisor wants me to switch to another software/programming language that I am not proficient in. What to do?
- Ring Buffer Implementation in C++
- Is there a phrase for someone who's really bad at cooking?
- What prevents a browser from saving and tracking passwords entered to a site?
- What does "seeing from one end of the world to the other" mean?
- Motion of the COM of 2-body system
C# Tutorial
C# examples, c# assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
Try it Yourself »
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Advantage of using compound assignment
What is the real advantage of using compound assignment in C/C++ (or may be applicable to many other programming languages as well)?
I looked at few links like microsoft site , SO post1 , SO Post2 . But the advantage says exp1 is evaluated only once in case of compound statement. How exp1 is really evaluated twice in first case? I understand that current value of exp1 is read first and then new value is added. Updated value is written back to the same location. How this really happens at lower level in case of compound statement? I tried to compare assembly code of two cases, but I did not see any difference between them.
- compound-assignment
- There's a good chance that your compiler optimised it. Anyway, assembly language usually has increment opcodes. In fact, they don't have anything else. If you do something like 1 + 2 in C, it would be compiled to something like move 1,a and add 2,a . – SeverityOne Commented Apr 19, 2018 at 13:22
- @SeverityOne's response should be the answer... – Frederick Commented Apr 19, 2018 at 13:27
6 Answers 6
For simple expressions involving ordinary variables, the difference between
is syntactical only. The two expressions will behave exactly the same, and might well generate identical assembly code. (You're right; in this case it doesn't even make much sense to ask whether a is evaluated once or twice.)
Where it gets interesting is when the left-hand side of the assignment is an expression involving side effects. So if you have something like
it makes much more of a difference! The former tries to increment p twice (and is therefore undefined). But the latter evaluates p++ precisely once, and is well-defined.
As others have mentioned, there are also advantages of notational convenience and readability. If you have
it can be hard to spot the bug. But if you use
it's impossible to even have that bug, and a later reader doesn't have to scrutinize the terms to rule out the possibility.
A minor advantage is that it can save you a pair of parentheses (or from a bug if you leave those parentheses out). Consider:
Finally (thanks to Jens Gustedt for reminding me of this), we have to go back and think a little more carefully about what we meant when we said "Where it gets interesting is when the left-hand side of the assignment is an expression involving side effects." Normally, we think of modifications as being side effects, and accesses as being "free". But for variables qualified as volatile (or, in C11, as _Atomic ), an access counts as an interesting side effect, too. So if variable a has one of those qualifiers, a = a + b is not a "simple expression involving ordinary variables", and it may not be so identical to a += b , after all.

- Got the real issue now. I think most books does not highlight this concept of two time evaluation by giving good example. In fact this is the most important difference than the readability. @dbush also gave another good example where we can easily see the result of two time evaluation. Disadvantage indicated by chux also give us better clarity. – Rajesh Commented Apr 19, 2018 at 14:58
- No, they are not always the same. It depends on what a is. If it is volatile or _Atomic the result is fundamentally different. – Jens Gustedt Commented Apr 19, 2018 at 18:54
- How would tmp = *XYZ; be interpreted and why? my compiler interprets this as: Copy *XYZ to temp, and why not tmp = tmp * XYZ ? Note: XYZ is pointer. – M Sharath Hegde Commented Aug 30, 2018 at 6:58
- 1 @MSharathHegde If the compound assignment operator were spelled " =* ", there might be some ambiguity here, but since it is in fact spelled " *= ", it's perfectly clear that tmp=*XYZ must involve a pointer access, not a multiplication. (Now, in the earliest days of C, the compound assignment operator was spelled " =* ", and there was ambiguity, which is why the compound assignment operators were rejiggered to their modern form.) – Steve Summit Commented Aug 30, 2018 at 9:27
Evaluating the left side once can save you a lot if it's more than a simple variable name. For example:
In this case some_long_running_function() is only called once. This differs from:
Which calls the function twice.
There is a disadvantage too. Consider the effect of types.
10 + b addition below will use int math and overflow ( undefined behavior )

- even clang -Weverything don't produce warning... it's should ! – Stargateur Commented Apr 19, 2018 at 14:42
This is what the standard 6.5.16.2 says:
A compound assignment of the form E1 op = E2 is equivalent to the simple assignment expression E1 = E1 op ( E2 ), except that the lvalue E1 is evaluated only once
So the "evaluated once" is the difference. This mostly matters in embedded systems where you have volatile qualifiers and don't want to read a hardware register several times, as that could cause unwanted side-effects.
That's not really possible to reproduce here on SO, so instead here's an artificial example to demonstrate why multiple evaluations could lead to different program behavior:
The simple assignment version did not only give a different result, it also introduced unspecified behavior in the code, so that two different results are possible depending on the compiler.
- correct. in addition to volatile it can also make a difference for atomic qualified types. – Jens Gustedt Commented Apr 19, 2018 at 18:57
Not sure what you're after. Compound assignment is shorter, and therefore simpler (less complex) than using regular operations.
Consider this:
Which one is easier to read and understand, and verify?
This, to me, is a very very real advantage, and is just as true regardless of semantic details like how many times something is evaluated.
- Arguably the main issue with your example is that you don't follow the law of Demeter. The difference between x += dt * speed and x =x + dt * speed is less, but still worth having. – Pete Kirkham Commented Apr 19, 2018 at 13:38
- I agree with this answer. Modern compilers usually do not need any "help" by programmers using specific language constructs. In fact today better readability of a code fragment usually also means better optimization by the compiler. – Blue Commented Apr 19, 2018 at 13:41
- @unwind I am mainly talking about performance related issues rather than readability. According to Microsoft, "However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation". Here is what I am expecting some kind of explanation. – Rajesh Commented Apr 19, 2018 at 13:49
A language like C is always going to be an abstraction of the underlying machine opcodes. In the case of addition, the compiler would first move the left operand into the accumulator, and add the right operand to it. Something like this (pseudo-assembler code):
This is what 1+2 would compile to in assembler. Obviously, this is perhaps over-simplified, but you get the idea.
Also, compiler tend to optimise your code, so exp1=exp1+b would very likely compile to the same opcodes as exp1+=b .
And, as @unwind remarked, the compound statement is a lot more readable.

- I am using TDM-GCC-64 compiler. Optimization is turned off. Here is the log 'gcc.exe -Wall -s -pedantic -Wextra -Wall -g -c "C:\sample_Project_Only_Main\main.c" -o Debug\main.o g++.exe -o Debug\sample_Project_Only_Main.exe Debug\main.o " – Rajesh Commented Apr 19, 2018 at 13:54
- Fair enough. Still, assembly always works along the lines of "add something to a register or memory location", which is what the += operator does. – SeverityOne Commented Apr 19, 2018 at 17:03
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c c11 compound-assignment or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Has a tire ever exploded inside the Wheel Well?
- Why are complex coordinates outlawed in physics?
- Can you give me an example of an implicit use of Godel's Completeness Theorem, say for example in group theory?
- Ramification at particular level of a tower of extensions of local field
- Took a pic of old school friend in the 80s who is now quite famous and written a huge selling memoir. Pic has ben used in book without permission
- Parody of Fables About Authenticity
- What happens if all nine Supreme Justices recuse themselves?
- Is there a difference between these two layouts?
- My supervisor wants me to switch to another software/programming language that I am not proficient in. What to do?
- What's the average distance traveled on a typical single engine aircraft?
- Why is the movie titled "Sweet Smell of Success"?
- Why does flow separation cause an increase in pressure drag?
- Why is Legion helping you whilst geth are fighting you?
- Why did the Fallschirmjäger have such terrible parachutes?
- Reusing own code at work without losing licence
- Can Shatter damage Manifest Mind?
- Motion of the COM of 2-body system
- Why does a halfing's racial trait lucky specify you must use the next roll?
- How does the summoned monster know who is my enemy?
- Cannot open and HTML file stored on RAM-disk with a browser
- How can moral disagreements be resolved when the conflicting parties are guided by fundamentally different value systems?
- Is it possible to accurately describe something without describing the rest of the universe?
- Which hash algorithms support binary input of arbitrary bit length?
- Which version of Netscape is this, on which OS?

COMMENTS
If you want to suppress only a single violation, add preprocessor directives to your source file to disable and then re-enable the rule. C#. Copy. #pragma warning disable IDE0054 // Or IDE0074 // The code that's violating the rule is on this line. #pragma warning restore IDE0054 // Or IDE0074. To disable the rule for a file, folder, or project ...
myFactory.GetNextObject().MyProperty += 5; You _certainly wouldn't do. myFactory.GetNextObject().MyProperty = myFactory.GetNextObject().MyProperty + 5; You could again use a temp variable, but the compound assignment operator is obviously more succinct. Granted, these are edge cases, but it's not a bad habit to get into.
The left-hand operand of ref assignment can be a local reference variable, a ref field, and a ref, out, or in method parameter. Both operands must be of the same type. Compound assignment. For a binary operator op, a compound assignment expression of the form. x op= y is equivalent to. x = x op y except that x is only evaluated once.
In this video, we'll explain compound assignment operators in C#. We'll cover what they are, how they work, and provide examples of their usage. You'll learn...
For more information, see the Numeric promotions section of the C# language specification. The ++ and --operators are defined for all integral and floating-point numeric types and the char type. The result type of a compound assignment expression is the type of the left-hand operand. Increment operator ++
Compound assignment operators in C - A compound assignment operator has a shorter syntax to assign the result. The operation is performed on the two operands before the result is assigned to the first operand.The following are the compound assignment operators in C#.Sr.NoOperator & Operator Name1+=Addition Assignment2-=Subtraction Assi.
Compound assignment operators in C# are shorthand operators that combine an arithmetic or bitwise operation with an assignment. They allow you to perform the operation and assign the result in a single step. This can make your code more concise and readable. Here's how you can use compound assignment operators in C#:
There are a lot of cool new features in C# 8 and one of my favorites is the new Null coalescing assignment (or compound assignment, whichever you prefer) operator. If you have ever written code like this: private string _someValue; public string SomeMethod() { // Let's do an old-school null check and initialize if needed.
A special case scenario for all the compound assigned operators. int i= 2 ; i+= 2 * 2 ; //equals to, i = i+(2*2); In all the compound assignment operators, the expression on the right side of = is always calculated first and then the compound assignment operator will start its functioning. Hence, in the last code, statement i+=2*2; is equal to ...
Compound assignment operators in C# allow you to combine an assignment operation with another arithmetic or bitwise operation to perform actions on a variable in a single statement. ### Syntax. The general syntax for a compound assignment operator is: variable op= expression; where: - variable is the variable to assign the result to - op is the ...
Hello! Today i want to ask you this: Have you ever used a Null Coalescing operator or even a Compound assignment operator in C# ? I never. Until today i had never heard about this things, so i want to share with you what i learned about and how it can be applied to your code. The problem Let's say you want to give a given variable the value of ...
C# provides special compound assignment operators that simplify the coding of certain assignment statements. The assignment statement shown here: x = x + 10; can be written using a compound assignment as. x += 10; The operator pair += tells the compiler to assign to x the value of x plus 10. Here is another example.
About Compound Assignment. Many operators can also be used as compound assignments, which are a shorthand notation where x = op y can be written as x op= y: var features = PhoneFeatures.Call; features |= PhoneFeatures.Text; features &= ~PhoneFeatures.Call;
In this code, you use the compound assignment operator (+=), which is a shortcut that does the same thing as the previous solution. You will see compound assignments in all C-family programming languages. A program that illustrates compound assignment in connection with subtraction, multiplication, and division. static void Main( string [] args)
The source for this content can be found on GitHub, where you can also create and review issues and pull requests. For more information, see our contributor guide.
The title is be misleading, so please read entire question :-). By "compound assignment operator" I have in mind a construct like this op=, for example +=.Pure assignment operator (=) does not belong to my question.By "why" I don't mean an opinion, but resource (book, article, etc) when some of the designers, or their coworkers, etc. express their reasoning (i.e. the source of the design choice).
C# Assignment Operators Previous Next Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example int x = 10;
Compound assignments are not atomic. x += 1 for instance is a syntactic sugar for read x from memory, add 1 and write value back to memory. If you want a good explanation of what is and what is not atomic read Eric Lippert's blog post on the subject: Atomicity, volatitly and immutability are different. answered Jun 9, 2011 at 11:29.
A violent standoff between police and followers of a fugitive preacher accused of sexual abuse and human trafficking entered a fourth day on Tuesday as nearly 2,000 officers surrounded a sprawling ...
6. Normally a += would add the expression/variable on the right hand side to the one on the left and assign the result to the left hand side. // if a = 4, after this statement, a would be 5. a += 1; But in case the left hand side of the expression with a += is an event, then this is not the case, but it would be the event handler on the right ...
According to Microsoft, "However, the compound-assignment expression is not equivalent to the expanded version because the compound-assignment expression evaluates expression1 only once, while the expanded version evaluates expression1 twice: in the addition operation and in the assignment operation". Here is what I am expecting some kind of ...